Note
Go to the end to download the full example code.
Oscillating plate#
This example is a version of the Oscillating Plate case that is often used as a tutorial for System Coupling. This two-way, fluid-structure interaction (FSI) case is based on co-simulation of a transient oscillating plate with surface data transfers.
Ansys Mechanical APDL (MAPDL) is used to perform a transient structural analysis.
Ansys Fluent is used to perform a transient fluid-flow analysis.
System Coupling coordinates the coupled solution involving the above products to solve the multiphysics problem via co-simulation.
Problem description
An oscillating plate resides within a fluid-filled cavity. A thin plate is anchored to the bottom of a closed cavity filled with fluid (air):
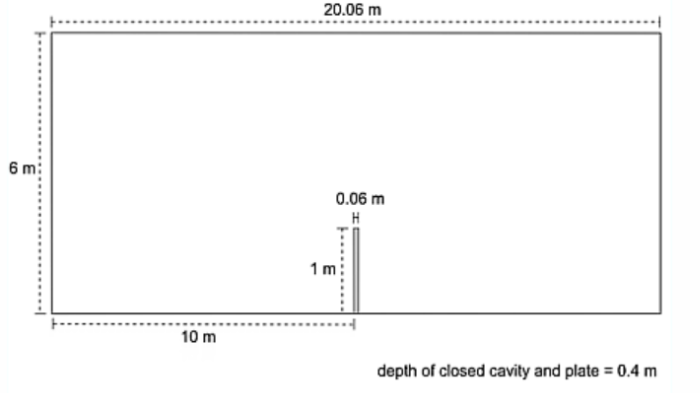
There is no friction between the plate and the side of the cavity. An initial constant force in x-direction is applied to one side of the thin plate for the first 0.5 seconds to distort it. Once this pressure is released, the plate oscillates back and forth to regain its equilibrium, and the surrounding air damps this oscillation. The plate and surrounding air are simulated for a few oscillations to allow an examination of the motion of the plate as it is damped.
Import modules, download files, launch products#
Setting up this example consists of performing imports, downloading the input file, and launching the required products.
Perform required imports#
Import ansys-systemcoupling-core
, ansys-fluent-core
and
ansys-mapdl-core
and other required packages.
import ansys.fluent.core as pyfluent
import ansys.mapdl.core as pymapdl
import ansys.systemcoupling.core as pysyc
from ansys.systemcoupling.core import examples
Download the input file#
This example uses one pre-created file - a Fluent input file that contains the fluids setup.
fluent_cas_file = examples.download_file(
"oscillating_plate.cas.h5", "pysystem-coupling/oscillating_plate"
)
Launch products#
Launch instances of the Mechanical APDL, Fluent, and System Coupling and return client (session) objects that allow you to interact with these products via APIs exposed into the current Python environment.
mapdl = pymapdl.launch_mapdl()
fluent = pyfluent.launch_fluent(start_transcript=False)
syc = pysyc.launch(start_output=True)
Setup#
The setup consists of setting up the structural analysis, the fluids analysis, and the coupled analysis.
Set up the structural analysis#
Enter Mechancal APDL setup
mapdl.prep7()
*****MAPDL VERIFICATION RUN ONLY*****
DO NOT USE RESULTS FOR PRODUCTION
***** MAPDL ANALYSIS DEFINITION (PREP7) *****
Define material properties.
mapdl.mp("DENS", 1, 2550) # density
mapdl.mp("ALPX", 1, 1.2e-05) # thermal expansion coefficient
mapdl.mp("EX", 1, 2500000) # Young's modulus
mapdl.mp("NUXY", 1, 0.35) # Poisson's ratio
MATERIAL 1 NUXY = 0.3500000
Set element types to SOLID186.
mapdl.et(1, 186)
mapdl.keyopt(1, 2, 1)
ELEMENT TYPE 1 IS SOLID186 3-D 20-NODE STRUCTURAL SOLID
KEYOPT( 1- 6)= 0 1 0 0 0 0
KEYOPT( 7-12)= 0 0 0 0 0 0
KEYOPT(13-18)= 0 0 0 0 0 0
CURRENT NODAL DOF SET IS UX UY UZ
THREE-DIMENSIONAL MODEL
Make geometry.
mapdl.block(10.00, 10.06, 0.0, 1.0, 0.0, 0.4)
mapdl.vsweep(1)
GENERATE NODES AND ELEMENTS IN VOLUME 1
SWEEPING VOLUME 1 FROM AREA 6 TO AREA 5
VOLUME 1 MESHED WITH 390 HEXAHEDRA AND 0 WEDGES
Volume Sweeping Complete
The Following Volumes Were Successfully Swept
1
MAXIMUM NODE NUMBER = 2374
MAXIMUM ELEMENT NUMBER = 390
Add fixed support at y=0.
mapdl.run("NSEL,S,LOC,Y,0")
mapdl.d("all", "all")
SPECIFIED CONSTRAINT UX FOR SELECTED NODES 1 TO 2374 BY 1
REAL= 0.00000000 IMAG= 0.00000000
ADDITIONAL DOFS= UY UZ
Add the FSI interface.
mapdl.nsel("S", "LOC", "X", 9.99, 10.01)
mapdl.nsel("A", "LOC", "Y", 0.99, 1.01)
mapdl.nsel("A", "LOC", "X", 10.05, 10.07)
mapdl.cm("FSIN_1", "NODE")
mapdl.sf("FSIN_1", "FSIN", 1)
GENERATE SURFACE LOAD FSIN ON SURFACE DEFINED BY ALL SELECTED NODES
FLUID SOLID INTERFACE NUMBER = 1.
NUMBER OF FSIN ELEMENT FACE LOADS STORED = 416
Set up the rest of the transient analysis
mapdl.allsel()
mapdl.run("/SOLU")
mapdl.antype(4) # transient analysis
mapdl.nlgeom("ON") # large deformations
mapdl.kbc(1)
mapdl.trnopt("full", "", "", "", "", "hht")
mapdl.tintp(0.1)
mapdl.autots("off")
mapdl.run("nsub,1,1,1")
mapdl.run("time,10.0")
mapdl.timint("on")
INCLUDE TRANSIENT EFFECTS FOR ALL DEGREES OF FREEDOM THIS LOAD STEP
Set up the fluid analysis#
Read the pre-created case file
fluent.file.read(file_type="case", file_name=fluent_cas_file)
Set up the coupled analysis#
System Coupling setup involves adding the structural and fluid participants, adding coupled interfaces and data transfers, and setting other coupled analysis properties.
Add participants by passing session handles to System Coupling.
solid_name = syc.setup.add_participant(participant_session=mapdl)
fluid_name = syc.setup.add_participant(participant_session=fluent)
syc.setup.coupling_participant[solid_name].display_name = "Solid"
syc.setup.coupling_participant[fluid_name].display_name = "Fluid"
Add a coupling interface and data transfers.
interface_name = syc.setup.add_interface(
side_one_participant=fluid_name,
side_one_regions=["wall_deforming"],
side_two_participant=solid_name,
side_two_regions=["FSIN_1"],
)
# set up 2-way FSI coupling - add force & displacement data transfers
dt_names = syc.setup.add_fsi_data_transfers(interface=interface_name)
# modify force transfer to apply constant initial loading for the first 0.5 [s]
force_transfer = syc.setup.coupling_interface[interface_name].data_transfer["FORC"]
force_transfer.option = "UsingExpression"
force_transfer.value = "vector(5.0 [N], 0.0 [N], 0.0 [N]) if Time < 0.5 [s] else force"
Time step size, end time, output controls
syc.setup.solution_control.time_step_size = "0.1 [s]" # time step is 0.1 [s]
syc.setup.solution_control.end_time = 10 # end time is 10.0 [s]
syc.setup.output_control.option = "EveryStep"
syc.setup.output_control.generate_csv_chart_output = True
Solution#
syc.solution.solve()
+=============================================================================+
| Coupling Participants (2) |
+=============================================================================+
| Fluid |
+-----------------------------------------------------------------------------+
| Internal Name : FLUENT-2 |
| Participant Type : FLUENT |
| Participant Display Name : FLUENT-2 |
| Dimension : 3D |
| Input Parameters : [] |
| Output Parameters : [] |
| Participant Analysis Type : Transient |
| Use New APIs : True |
| Restarts Supported : True |
| Variables (3) |
| Variable : Lorentz_Force |
| Internal Name : lorentz-force |
| Quantity Type : Force |
| Participant Display Name : Lorentz Force |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : True |
| |
| Variable : displacement |
| Internal Name : displacement |
| Quantity Type : Incremental Displacement |
| Participant Display Name : displacement |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : False |
| |
| Variable : force |
| Internal Name : force |
| Quantity Type : Force |
| Participant Display Name : force |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : True |
| Regions (6) |
| Region : part-fluid |
| Internal Name : part-fluid |
| Topology : Volume |
| Input Variables : [] |
| Output Variables : [] |
| Region Discretization Type : Mesh Region |
| |
| Region : wall_bottom |
| Internal Name : wall_bottom |
| Topology : Surface |
| Input Variables : [] |
| Output Variables : [force] |
| Region Discretization Type : Mesh Region |
| |
| Region : wall_deforming |
| Internal Name : wall_deforming |
| Topology : Surface |
| Input Variables : [displacement] |
| Output Variables : [force] |
| Region Discretization Type : Mesh Region |
| |
| Region : wall_inlet |
| Internal Name : wall_inlet |
| Topology : Surface |
| Input Variables : [] |
| Output Variables : [force] |
| Region Discretization Type : Mesh Region |
| |
| Region : wall_outlet |
| Internal Name : wall_outlet |
| Topology : Surface |
| Input Variables : [] |
| Output Variables : [force] |
| Region Discretization Type : Mesh Region |
| |
| Region : wall_top |
| Internal Name : wall_top |
| Topology : Surface |
| Input Variables : [] |
| Output Variables : [force] |
| Region Discretization Type : Mesh Region |
| Properties |
| Accepts New Inputs : False |
| Time Integration : Implicit |
| Update Control |
| Option : ProgramControlled |
| Execution Control |
| Option : ExternallyManaged |
+-----------------------------------------------------------------------------+
| Solid |
+-----------------------------------------------------------------------------+
| Internal Name : MAPDL-1 |
| Participant Type : MAPDL |
| Participant Display Name : MAPDL-1 |
| Dimension : 3D |
| Input Parameters : [] |
| Output Parameters : [] |
| Participant Analysis Type : Transient |
| Restarts Supported : True |
| Variables (3) |
| Variable : Force |
| Internal Name : FORC |
| Quantity Type : Force |
| Location : Node |
| Participant Display Name : Force |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : True |
| |
| Variable : Force_Density |
| Internal Name : FDNS |
| Quantity Type : Force |
| Location : Element |
| Participant Display Name : Force Density |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : False |
| |
| Variable : Incremental_Displacement |
| Internal Name : INCD |
| Quantity Type : Incremental Displacement |
| Location : Node |
| Participant Display Name : Incremental Displacement |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : False |
| Regions (1) |
| Region : System Coupling (Surface) Region 0 |
| Internal Name : FSIN_1 |
| Topology : Surface |
| Input Variables : [FORC, FDNS] |
| Output Variables : [INCD] |
| Region Discretization Type : Mesh Region |
| Properties |
| Accepts New Inputs : False |
| Time Integration : Implicit |
| Update Control |
| Option : ProgramControlled |
| Execution Control |
| Option : ExternallyManaged |
+=============================================================================+
| Analysis Control |
+=============================================================================+
| Analysis Type : Transient |
| Optimize If One Way : True |
| Allow Simultaneous Update : False |
| Partitioning Algorithm : SharedAllocateMachines |
| Global Stabilization |
| Option : None |
+=============================================================================+
| Coupling Interfaces (1) |
+=============================================================================+
| Interface-1 |
+-----------------------------------------------------------------------------+
| Internal Name : Interface-1 |
| Side |
| Side: One |
| Coupling Participant : FLUENT-2 |
| Region List : [wall_deforming] |
| Reference Frame : GlobalReferenceFrame |
| Instancing : None |
| Side: Two |
| Coupling Participant : MAPDL-1 |
| Region List : [FSIN_1] |
| Reference Frame : GlobalReferenceFrame |
| Instancing : None |
| Data Transfers (2) |
| DataTransfer : Force |
| Internal Name : FORC |
| Suppress : False |
| Target Side : Two |
| Option : UsingExpression |
| Target Variable : FORC |
| Value : vector(5.0 [N], 0.0 [N], 0.0 [N]) if Time < 0.5 [s] |
| else force |
| Ramping Option : None |
| Relaxation Factor : 1.00e+00 |
| Convergence Target : 1.00e-02 |
| Mapping Type : Conservative |
| DataTransfer : displacement |
| Internal Name : displacement |
| Suppress : False |
| Target Side : One |
| Option : UsingVariable |
| Source Variable : INCD |
| Target Variable : displacement |
| Ramping Option : None |
| Relaxation Factor : 1.00e+00 |
| Convergence Target : 1.00e-02 |
| Mapping Type : ProfilePreserving |
| Unmapped Value Option : ProgramControlled |
| Mapping Control |
| Stop If Poor Intersection : True |
| Poor Intersection Threshold : 5.00e-01 |
| Face Alignment : ProgramControlled |
| Absolute Gap Tolerance : 0.0 [m] |
| Relative Gap Tolerance : 1.00e+00 |
+=============================================================================+
| Solution Control |
+=============================================================================+
| Duration Option : EndTime |
| End Time : 1.00e+01 |
| Time Step Size : 0.1 [s] |
| Minimum Iterations : 1 |
| Maximum Iterations : 5 |
| Use IP Address When Possible : True |
+=============================================================================+
| Output Control |
+=============================================================================+
| Option : EveryStep |
| Generate CSV Chart Output : True |
| Write Initial Snapshot : True |
| Results |
| Option : ProgramControlled |
| Include Instances : ProgramControlled |
| Type |
| Option : EnsightGold |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warnings were found during data model validation. |
+-----------------------------------------------------------------------------+
| Warning: Participant Fluid (CouplingParticipant:FLUENT-2) has the |
| ExecutionControl 'Option' set to 'ExternallyManaged'. System Coupling |
| will not control the startup/shutdown behavior of this participant. |
| Warning: Participant Solid (CouplingParticipant:MAPDL-1) has the |
| ExecutionControl 'Option' set to 'ExternallyManaged'. System Coupling |
| will not control the startup/shutdown behavior of this participant. |
| Warning: Unused input variables ['Force_Density'] (FDNS) on region FSIN_1 |
| for Solid (CouplingParticipant:MAPDL-1). |
+-----------------------------------------------------------------------------+
+=============================================================================+
| Execution Information |
+=============================================================================+
| |
| System Coupling |
| Command Line Arguments: |
| -m cosimgui --grpcport=0.0.0.0:33011 |
| Working Directory: |
| /working |
| |
| Fluid |
| Not started by System Coupling |
| |
| Solid |
| Not started by System Coupling |
| |
+=============================================================================+
Awaiting connections from coupling participants... done.
+=============================================================================+
| Build Information |
+-----------------------------------------------------------------------------+
| System Coupling |
| 2025 R1: Build ID: 51e1bda Build Date: 2025-03-17T11:36 |
| Fluid |
| ANSYS Fluent 24.0 2.0 0.0 Build Time: May 13 2024 11:01:41 EDT Build Id: |
| 10192 |
| Solid |
| Mechanical APDL Release Build 24.2 UP20240603 |
| DISTRIBUTED LINUX x64 Version |
+=============================================================================+
+-----------------------------------------------------------------------------+
| MESH STATISTICS |
+-----------------------------------------------------------------------------+
| Participant: FLUENT-2 |
| Number of face regions 1 |
| Number of faces 11 |
| Quadrilateral 11 |
| Area (m2) 8.240e-01 |
| Bounding Box (m) |
| Minimum [ 1.000e+01 0.000e+00 0.000e+00] |
| Maximum [ 1.006e+01 1.000e+00 4.000e-01] |
| |
| Participant: MAPDL-1 |
| Number of face regions 1 |
| Number of faces 416 |
| Quadrilateral8 416 |
| Area (m2) 8.240e-01 |
| Bounding Box (m) |
| Minimum [ 1.000e+01 0.000e+00 0.000e+00] |
| Maximum [ 1.006e+01 1.000e+00 4.000e-01] |
| |
| Total |
| Number of cells 0 |
| Number of faces 427 |
| Number of nodes 1 363 |
+-----------------------------------------------------------------------------+
+-----------------------------------------------------------------------------+
| MAPPING SUMMARY |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| Interface-1 | |
| Force | |
| Mapped Area [%] | 100 100 |
| Mapped Elements [%] | 100 100 |
| Mapped Nodes [%] | 100 100 |
| displacement | |
| Mapped Area [%] | 100 100 |
| Mapped Elements [%] | 100 100 |
| Mapped Nodes [%] | 100 100 |
+-----------------------------------------------------------------------------+
+-----------------------------------------------------------------------------+
| Transfer Diagnostics |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | |
| Weighted Average x | 0.00E+00 0.00E+00 |
| Weighted Average y | 0.00E+00 0.00E+00 |
| Weighted Average z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
===============================================================================
+=============================================================================+
| |
| Analysis Initialization |
| |
+=============================================================================+
===============================================================================
===============================================================================
+=============================================================================+
| |
| Coupled Solution |
| |
+=============================================================================+
===============================================================================
+=============================================================================+
| COUPLING STEP = 1 SIMULATION TIME = 1.00000E-01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.00E+00 1.00E+00 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.00E+00 1.00E+00 |
| Weighted Average x | 4.45E-03 4.48E-03 |
| Weighted Average y | -1.81E-05 -1.83E-05 |
| Weighted Average z | 3.99E-16 4.29E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Weighted Average x | 4.45E-03 4.48E-03 |
| Weighted Average y | -1.81E-05 -1.83E-05 |
| Weighted Average z | 3.99E-16 4.29E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Weighted Average x | 4.45E-03 4.48E-03 |
| Weighted Average y | -1.81E-05 -1.83E-05 |
| Weighted Average z | 3.99E-16 4.29E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 2 SIMULATION TIME = 2.00000E-01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.94E-01 8.04E-01 |
| Weighted Average x | 1.51E-02 1.52E-02 |
| Weighted Average y | -3.30E-04 -3.32E-04 |
| Weighted Average z | -7.71E-16 -8.59E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.71E-03 3.81E-03 |
| Weighted Average x | 1.51E-02 1.51E-02 |
| Weighted Average y | -3.33E-04 -3.34E-04 |
| Weighted Average z | -7.46E-16 -8.34E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.85E-04 1.97E-04 |
| Weighted Average x | 1.51E-02 1.51E-02 |
| Weighted Average y | -3.32E-04 -3.34E-04 |
| Weighted Average z | -7.66E-16 -8.58E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 3 SIMULATION TIME = 3.00000E-01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.85E-01 7.96E-01 |
| Weighted Average x | 2.68E-02 2.68E-02 |
| Weighted Average y | -1.82E-03 -1.83E-03 |
| Weighted Average z | -2.92E-15 -2.96E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.93E-03 2.09E-03 |
| Weighted Average x | 2.67E-02 2.68E-02 |
| Weighted Average y | -1.83E-03 -1.83E-03 |
| Weighted Average z | -2.83E-15 -2.87E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.04E-04 1.17E-04 |
| Weighted Average x | 2.67E-02 2.68E-02 |
| Weighted Average y | -1.83E-03 -1.83E-03 |
| Weighted Average z | -2.79E-15 -2.82E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 4 SIMULATION TIME = 4.00000E-01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.57E-01 7.72E-01 |
| Weighted Average x | 3.67E-02 3.67E-02 |
| Weighted Average y | -5.48E-03 -5.47E-03 |
| Weighted Average z | -2.09E-16 -1.87E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.18E-03 1.22E-03 |
| Weighted Average x | 3.67E-02 3.67E-02 |
| Weighted Average y | -5.49E-03 -5.47E-03 |
| Weighted Average z | -1.39E-16 -1.25E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Sum x | 1.20E+02 1.20E+02 |
| Sum y | 0.00E+00 0.00E+00 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.52E-05 2.52E-05 |
| Weighted Average x | 3.67E-02 3.67E-02 |
| Weighted Average y | -5.49E-03 -5.47E-03 |
| Weighted Average z | -1.97E-16 -1.62E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 5 SIMULATION TIME = 5.00000E-01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 6.17E+01 5.51E+01 |
| Sum x | -1.41E+00 -1.41E+00 |
| Sum y | 3.43E-01 3.43E-01 |
| Sum z | -2.40E-13 -2.40E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.48E-01 7.64E-01 |
| Weighted Average x | 4.02E-02 4.02E-02 |
| Weighted Average y | -1.08E-02 -1.07E-02 |
| Weighted Average z | 4.51E-15 4.72E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.01E-01 1.66E-01 |
| Sum x | -1.12E+00 -1.12E+00 |
| Sum y | 4.86E-01 4.86E-01 |
| Sum z | 1.61E-13 1.61E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.02E-03 2.14E-03 |
| Weighted Average x | 4.02E-02 4.02E-02 |
| Weighted Average y | -1.07E-02 -1.07E-02 |
| Weighted Average z | 4.33E-15 4.50E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.14E-03 4.17E-03 |
| Sum x | -1.11E+00 -1.11E+00 |
| Sum y | 4.84E-01 4.84E-01 |
| Sum z | 1.43E-13 1.43E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.09E-05 5.23E-05 |
| Weighted Average x | 4.02E-02 4.02E-02 |
| Weighted Average y | -1.07E-02 -1.07E-02 |
| Weighted Average z | 4.34E-15 4.51E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 6 SIMULATION TIME = 6.00000E-01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.95E-04 2.41E-04 |
| Sum x | -1.11E+00 -1.11E+00 |
| Sum y | 4.84E-01 4.84E-01 |
| Sum z | 1.46E-13 1.46E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.48E-01 7.65E-01 |
| Weighted Average x | 3.58E-02 3.58E-02 |
| Weighted Average y | -1.48E-02 -1.47E-02 |
| Weighted Average z | 1.45E-15 1.58E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 4.84E-01 3.55E-01 |
| Sum x | -5.65E-01 -5.65E-01 |
| Sum y | 4.36E-01 4.36E-01 |
| Sum z | 1.24E-12 1.24E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.58E-03 2.69E-03 |
| Weighted Average x | 3.59E-02 3.58E-02 |
| Weighted Average y | -1.47E-02 -1.46E-02 |
| Weighted Average z | 1.29E-15 1.41E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.25E-03 6.65E-03 |
| Sum x | -5.62E-01 -5.62E-01 |
| Sum y | 4.32E-01 4.32E-01 |
| Sum z | 5.71E-13 5.71E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.05E-04 1.08E-04 |
| Weighted Average x | 3.58E-02 3.58E-02 |
| Weighted Average y | -1.47E-02 -1.46E-02 |
| Weighted Average z | 1.22E-15 1.35E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 7 SIMULATION TIME = 7.00000E-01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.70E-04 5.56E-04 |
| Sum x | -5.61E-01 -5.61E-01 |
| Sum y | 4.32E-01 4.32E-01 |
| Sum z | 5.63E-13 5.63E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.44E-01 7.62E-01 |
| Weighted Average x | 2.96E-02 2.96E-02 |
| Weighted Average y | -1.51E-02 -1.50E-02 |
| Weighted Average z | -9.69E-15 -9.70E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 5.59E-01 3.55E-01 |
| Sum x | -2.58E-01 -2.58E-01 |
| Sum y | 3.22E-01 3.22E-01 |
| Sum z | 3.00E-13 3.00E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.64E-03 1.78E-03 |
| Weighted Average x | 2.96E-02 2.96E-02 |
| Weighted Average y | -1.50E-02 -1.50E-02 |
| Weighted Average z | -9.57E-15 -9.63E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.22E-02 7.68E-03 |
| Sum x | -2.55E-01 -2.55E-01 |
| Sum y | 3.19E-01 3.19E-01 |
| Sum z | 9.56E-13 9.56E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.12E-05 5.60E-05 |
| Weighted Average x | 2.96E-02 2.96E-02 |
| Weighted Average y | -1.50E-02 -1.50E-02 |
| Weighted Average z | -9.54E-15 -9.60E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 8 SIMULATION TIME = 8.00000E-01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.25E-04 5.75E-04 |
| Sum x | -2.55E-01 -2.55E-01 |
| Sum y | 3.19E-01 3.19E-01 |
| Sum z | 9.59E-13 9.59E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.63E-01 7.77E-01 |
| Weighted Average x | 2.31E-02 2.32E-02 |
| Weighted Average y | -1.28E-02 -1.27E-02 |
| Weighted Average z | -1.51E-14 -1.54E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.23E+00 6.76E-01 |
| Sum x | -5.43E-03 -5.43E-03 |
| Sum y | 1.55E-01 1.55E-01 |
| Sum z | 6.46E-13 6.46E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.09E-03 1.10E-03 |
| Weighted Average x | 2.30E-02 2.31E-02 |
| Weighted Average y | -1.28E-02 -1.27E-02 |
| Weighted Average z | -1.47E-14 -1.50E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.99E-02 2.19E-02 |
| Sum x | 9.93E-04 9.93E-04 |
| Sum y | 1.50E-01 1.50E-01 |
| Sum z | 7.22E-13 7.22E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.74E-05 6.14E-05 |
| Weighted Average x | 2.30E-02 2.31E-02 |
| Weighted Average y | -1.28E-02 -1.27E-02 |
| Weighted Average z | -1.47E-14 -1.50E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.56E-03 1.38E-03 |
| Sum x | 1.11E-03 1.11E-03 |
| Sum y | 1.50E-01 1.50E-01 |
| Sum z | 7.22E-13 7.22E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 4.33E-06 4.79E-06 |
| Weighted Average x | 2.30E-02 2.31E-02 |
| Weighted Average y | -1.28E-02 -1.27E-02 |
| Weighted Average z | -1.47E-14 -1.50E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 9 SIMULATION TIME = 9.00000E-01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 8.35E-05 4.51E-05 |
| Sum x | 1.12E-03 1.12E-03 |
| Sum y | 1.50E-01 1.50E-01 |
| Sum z | 7.22E-13 7.22E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.09E-01 8.19E-01 |
| Weighted Average x | 1.52E-02 1.53E-02 |
| Weighted Average y | -9.18E-03 -9.20E-03 |
| Weighted Average z | -3.54E-15 -3.84E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.31E+00 9.29E-01 |
| Sum x | 3.25E-01 3.25E-01 |
| Sum y | -8.88E-02 -8.88E-02 |
| Sum z | 9.24E-13 9.24E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.51E-03 3.91E-03 |
| Weighted Average x | 1.52E-02 1.53E-02 |
| Weighted Average y | -9.17E-03 -9.19E-03 |
| Weighted Average z | -3.20E-15 -3.47E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.45E-02 2.39E-02 |
| Sum x | 3.32E-01 3.32E-01 |
| Sum y | -9.43E-02 -9.43E-02 |
| Sum z | 8.54E-13 8.54E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.83E-04 2.07E-04 |
| Weighted Average x | 1.52E-02 1.53E-02 |
| Weighted Average y | -9.17E-03 -9.19E-03 |
| Weighted Average z | -3.12E-15 -3.39E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.53E-03 1.06E-03 |
| Sum x | 3.32E-01 3.32E-01 |
| Sum y | -9.44E-02 -9.44E-02 |
| Sum z | 8.58E-13 8.58E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.26E-05 1.41E-05 |
| Weighted Average x | 1.52E-02 1.53E-02 |
| Weighted Average y | -9.17E-03 -9.19E-03 |
| Weighted Average z | -3.18E-15 -3.45E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 10 SIMULATION TIME = 1.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.07E-05 3.46E-05 |
| Sum x | 3.32E-01 3.32E-01 |
| Sum y | -9.44E-02 -9.44E-02 |
| Sum z | 8.58E-13 8.58E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.91E-01 8.01E-01 |
| Weighted Average x | 6.64E-03 6.69E-03 |
| Weighted Average y | -4.26E-03 -4.26E-03 |
| Weighted Average z | 1.72E-14 1.72E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 5.34E-01 4.18E-01 |
| Sum x | 6.47E-01 6.47E-01 |
| Sum y | -3.52E-01 -3.52E-01 |
| Sum z | 1.45E-12 1.45E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.34E-03 2.55E-03 |
| Weighted Average x | 6.64E-03 6.68E-03 |
| Weighted Average y | -4.26E-03 -4.26E-03 |
| Weighted Average z | 1.69E-14 1.69E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.49E-02 1.14E-02 |
| Sum x | 6.53E-01 6.53E-01 |
| Sum y | -3.57E-01 -3.57E-01 |
| Sum z | 1.51E-12 1.51E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.18E-05 8.81E-05 |
| Weighted Average x | 6.64E-03 6.68E-03 |
| Weighted Average y | -4.26E-03 -4.26E-03 |
| Weighted Average z | 1.69E-14 1.70E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.08E-03 8.33E-04 |
| Sum x | 6.53E-01 6.53E-01 |
| Sum y | -3.57E-01 -3.57E-01 |
| Sum z | 1.51E-12 1.51E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 4.55E-06 5.00E-06 |
| Weighted Average x | 6.64E-03 6.68E-03 |
| Weighted Average y | -4.26E-03 -4.26E-03 |
| Weighted Average z | 1.69E-14 1.69E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 11 SIMULATION TIME = 1.10000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.29E-05 2.52E-05 |
| Sum x | 6.53E-01 6.53E-01 |
| Sum y | -3.57E-01 -3.57E-01 |
| Sum z | 1.52E-12 1.52E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.25E-01 8.40E-01 |
| Weighted Average x | -2.25E-03 -2.29E-03 |
| Weighted Average y | 1.14E-03 1.17E-03 |
| Weighted Average z | 2.57E-14 2.61E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.97E-01 2.33E-01 |
| Sum x | 9.13E-01 9.13E-01 |
| Sum y | -5.72E-01 -5.72E-01 |
| Sum z | 1.68E-12 1.68E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.72E-02 1.93E-02 |
| Weighted Average x | -2.23E-03 -2.27E-03 |
| Weighted Average y | 1.13E-03 1.15E-03 |
| Weighted Average z | 2.52E-14 2.56E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.27E-03 1.50E-03 |
| Sum x | 9.13E-01 9.13E-01 |
| Sum y | -5.72E-01 -5.72E-01 |
| Sum z | 1.71E-12 1.71E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.05E-04 1.04E-03 |
| Weighted Average x | -2.23E-03 -2.27E-03 |
| Weighted Average y | 1.13E-03 1.15E-03 |
| Weighted Average z | 2.51E-14 2.55E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 12 SIMULATION TIME = 1.20000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.29E-04 8.67E-05 |
| Sum x | 9.13E-01 9.13E-01 |
| Sum y | -5.72E-01 -5.72E-01 |
| Sum z | 1.72E-12 1.72E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.03E-01 8.12E-01 |
| Weighted Average x | -1.07E-02 -1.08E-02 |
| Weighted Average y | 6.19E-03 6.20E-03 |
| Weighted Average z | 1.62E-14 1.64E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.34E-01 1.09E-01 |
| Sum x | 1.06E+00 1.06E+00 |
| Sum y | -6.63E-01 -6.63E-01 |
| Sum z | 1.49E-12 1.49E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.63E-03 3.63E-03 |
| Weighted Average x | -1.07E-02 -1.08E-02 |
| Weighted Average y | 6.19E-03 6.20E-03 |
| Weighted Average z | 1.59E-14 1.61E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.56E-03 5.21E-03 |
| Sum x | 1.05E+00 1.05E+00 |
| Sum y | -6.59E-01 -6.59E-01 |
| Sum z | 1.52E-12 1.52E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.19E-04 1.20E-04 |
| Weighted Average x | -1.07E-02 -1.08E-02 |
| Weighted Average y | 6.19E-03 6.20E-03 |
| Weighted Average z | 1.59E-14 1.61E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 13 SIMULATION TIME = 1.30000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.66E-04 2.08E-04 |
| Sum x | 1.05E+00 1.05E+00 |
| Sum y | -6.59E-01 -6.59E-01 |
| Sum z | 1.52E-12 1.52E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.65E-01 7.78E-01 |
| Weighted Average x | -1.77E-02 -1.78E-02 |
| Weighted Average y | 1.09E-02 1.08E-02 |
| Weighted Average z | 4.39E-15 4.35E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 8.99E-02 7.28E-02 |
| Sum x | 1.11E+00 1.11E+00 |
| Sum y | -6.56E-01 -6.56E-01 |
| Sum z | 9.05E-13 9.05E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.14E-03 1.13E-03 |
| Weighted Average x | -1.77E-02 -1.78E-02 |
| Weighted Average y | 1.08E-02 1.08E-02 |
| Weighted Average z | 4.48E-15 4.45E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.74E-03 6.35E-03 |
| Sum x | 1.10E+00 1.10E+00 |
| Sum y | -6.52E-01 -6.52E-01 |
| Sum z | 1.42E-12 1.42E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.37E-05 2.39E-05 |
| Weighted Average x | -1.77E-02 -1.78E-02 |
| Weighted Average y | 1.08E-02 1.08E-02 |
| Weighted Average z | 4.20E-15 4.17E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 14 SIMULATION TIME = 1.40000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.95E-04 2.41E-04 |
| Sum x | 1.10E+00 1.10E+00 |
| Sum y | -6.52E-01 -6.52E-01 |
| Sum z | 1.43E-12 1.43E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.50E-01 7.66E-01 |
| Weighted Average x | -2.41E-02 -2.42E-02 |
| Weighted Average y | 1.41E-02 1.41E-02 |
| Weighted Average z | -3.97E-15 -4.19E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 9.72E-02 8.11E-02 |
| Sum x | 1.14E+00 1.14E+00 |
| Sum y | -5.82E-01 -5.82E-01 |
| Sum z | 1.58E-12 1.58E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.55E-03 1.62E-03 |
| Weighted Average x | -2.41E-02 -2.42E-02 |
| Weighted Average y | 1.41E-02 1.40E-02 |
| Weighted Average z | -3.86E-15 -4.08E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.69E-03 4.88E-03 |
| Sum x | 1.13E+00 1.13E+00 |
| Sum y | -5.79E-01 -5.79E-01 |
| Sum z | 1.58E-12 1.58E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.70E-05 5.80E-05 |
| Weighted Average x | -2.41E-02 -2.42E-02 |
| Weighted Average y | 1.41E-02 1.40E-02 |
| Weighted Average z | -3.87E-15 -4.06E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 15 SIMULATION TIME = 1.50000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.60E-04 3.06E-04 |
| Sum x | 1.13E+00 1.13E+00 |
| Sum y | -5.79E-01 -5.79E-01 |
| Sum z | 1.58E-12 1.58E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.43E-01 7.61E-01 |
| Weighted Average x | -3.11E-02 -3.11E-02 |
| Weighted Average y | 1.47E-02 1.46E-02 |
| Weighted Average z | -1.43E-14 -1.45E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.06E-01 8.82E-02 |
| Sum x | 1.24E+00 1.24E+00 |
| Sum y | -4.68E-01 -4.68E-01 |
| Sum z | 1.74E-12 1.74E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.98E-03 2.13E-03 |
| Weighted Average x | -3.11E-02 -3.11E-02 |
| Weighted Average y | 1.47E-02 1.46E-02 |
| Weighted Average z | -1.41E-14 -1.43E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.50E-03 2.05E-03 |
| Sum x | 1.24E+00 1.24E+00 |
| Sum y | -4.67E-01 -4.67E-01 |
| Sum z | 1.38E-12 1.38E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 4.92E-05 5.25E-05 |
| Weighted Average x | -3.11E-02 -3.11E-02 |
| Weighted Average y | 1.47E-02 1.46E-02 |
| Weighted Average z | -1.41E-14 -1.43E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 16 SIMULATION TIME = 1.60000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.95E-05 6.39E-05 |
| Sum x | 1.24E+00 1.24E+00 |
| Sum y | -4.67E-01 -4.67E-01 |
| Sum z | 1.38E-12 1.38E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.50E-01 7.67E-01 |
| Weighted Average x | -3.82E-02 -3.82E-02 |
| Weighted Average y | 1.30E-02 1.29E-02 |
| Weighted Average z | -2.05E-14 -2.05E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.20E-01 1.01E-01 |
| Sum x | 1.35E+00 1.35E+00 |
| Sum y | -3.16E-01 -3.16E-01 |
| Sum z | 9.92E-13 9.92E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.58E-03 1.69E-03 |
| Weighted Average x | -3.81E-02 -3.81E-02 |
| Weighted Average y | 1.30E-02 1.29E-02 |
| Weighted Average z | -2.02E-14 -2.03E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.93E-03 2.45E-03 |
| Sum x | 1.35E+00 1.35E+00 |
| Sum y | -3.15E-01 -3.15E-01 |
| Sum z | 1.02E-12 1.02E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.34E-05 3.77E-05 |
| Weighted Average x | -3.81E-02 -3.81E-02 |
| Weighted Average y | 1.30E-02 1.29E-02 |
| Weighted Average z | -2.02E-14 -2.02E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 17 SIMULATION TIME = 1.70000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.40E-05 3.44E-05 |
| Sum x | 1.35E+00 1.35E+00 |
| Sum y | -3.15E-01 -3.15E-01 |
| Sum z | 1.02E-12 1.02E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.66E-01 7.80E-01 |
| Weighted Average x | -4.39E-02 -4.39E-02 |
| Weighted Average y | 9.54E-03 9.52E-03 |
| Weighted Average z | -1.38E-14 -1.40E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.29E-01 1.07E-01 |
| Sum x | 1.36E+00 1.36E+00 |
| Sum y | -1.28E-01 -1.28E-01 |
| Sum z | 5.43E-13 5.43E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.24E-03 1.25E-03 |
| Weighted Average x | -4.38E-02 -4.39E-02 |
| Weighted Average y | 9.53E-03 9.50E-03 |
| Weighted Average z | -1.35E-14 -1.37E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.06E-03 4.19E-03 |
| Sum x | 1.35E+00 1.35E+00 |
| Sum y | -1.26E-01 -1.26E-01 |
| Sum z | 5.08E-13 5.08E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.36E-05 1.37E-05 |
| Weighted Average x | -4.38E-02 -4.39E-02 |
| Weighted Average y | 9.53E-03 9.50E-03 |
| Weighted Average z | -1.36E-14 -1.38E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 18 SIMULATION TIME = 1.80000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.26E-04 1.03E-04 |
| Sum x | 1.35E+00 1.35E+00 |
| Sum y | -1.26E-01 -1.26E-01 |
| Sum z | 5.08E-13 5.08E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.72E-01 7.84E-01 |
| Weighted Average x | -4.72E-02 -4.72E-02 |
| Weighted Average y | 4.78E-03 4.76E-03 |
| Weighted Average z | 7.44E-16 6.74E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.70E-01 1.35E-01 |
| Sum x | 1.22E+00 1.22E+00 |
| Sum y | 6.71E-02 6.71E-02 |
| Sum z | -3.53E-14 -3.53E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.28E-03 1.27E-03 |
| Weighted Average x | -4.71E-02 -4.72E-02 |
| Weighted Average y | 4.78E-03 4.76E-03 |
| Weighted Average z | 7.30E-16 6.67E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.00E-03 7.05E-03 |
| Sum x | 1.21E+00 1.21E+00 |
| Sum y | 6.75E-02 6.75E-02 |
| Sum z | -5.49E-14 -5.49E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.05E-05 3.26E-05 |
| Weighted Average x | -4.71E-02 -4.72E-02 |
| Weighted Average y | 4.78E-03 4.76E-03 |
| Weighted Average z | 6.85E-16 6.18E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 19 SIMULATION TIME = 1.90000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.76E-04 2.14E-04 |
| Sum x | 1.21E+00 1.21E+00 |
| Sum y | 6.75E-02 6.75E-02 |
| Sum z | -5.64E-14 -5.64E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.64E-01 7.78E-01 |
| Weighted Average x | -4.74E-02 -4.74E-02 |
| Weighted Average y | -5.08E-04 -5.10E-04 |
| Weighted Average z | 1.31E-14 1.32E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.41E-01 1.75E-01 |
| Sum x | 9.69E-01 9.69E-01 |
| Sum y | 2.23E-01 2.23E-01 |
| Sum z | -2.99E-14 -2.99E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.09E-03 1.09E-03 |
| Weighted Average x | -4.73E-02 -4.73E-02 |
| Weighted Average y | -5.02E-04 -5.04E-04 |
| Weighted Average z | 1.28E-14 1.29E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.07E-02 7.66E-03 |
| Sum x | 9.58E-01 9.58E-01 |
| Sum y | 2.21E-01 2.21E-01 |
| Sum z | -2.39E-14 -2.39E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.50E-05 1.52E-05 |
| Weighted Average x | -4.73E-02 -4.73E-02 |
| Weighted Average y | -5.02E-04 -5.04E-04 |
| Weighted Average z | 1.28E-14 1.29E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 20 SIMULATION TIME = 2.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.48E-04 3.17E-04 |
| Sum x | 9.57E-01 9.57E-01 |
| Sum y | 2.21E-01 2.21E-01 |
| Sum z | -2.28E-14 -2.28E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.55E-01 7.71E-01 |
| Weighted Average x | -4.48E-02 -4.48E-02 |
| Weighted Average y | -5.68E-03 -5.66E-03 |
| Weighted Average z | 1.32E-14 1.35E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.58E-01 1.71E-01 |
| Sum x | 7.14E-01 7.14E-01 |
| Sum y | 3.21E-01 3.21E-01 |
| Sum z | 3.58E-13 3.58E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.29E-03 1.36E-03 |
| Weighted Average x | -4.47E-02 -4.47E-02 |
| Weighted Average y | -5.67E-03 -5.64E-03 |
| Weighted Average z | 1.28E-14 1.30E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.59E-03 6.26E-03 |
| Sum x | 7.07E-01 7.07E-01 |
| Sum y | 3.19E-01 3.19E-01 |
| Sum z | 2.87E-13 2.87E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.89E-05 2.00E-05 |
| Weighted Average x | -4.47E-02 -4.47E-02 |
| Weighted Average y | -5.67E-03 -5.64E-03 |
| Weighted Average z | 1.29E-14 1.31E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 21 SIMULATION TIME = 2.10000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.70E-04 3.05E-04 |
| Sum x | 7.06E-01 7.06E-01 |
| Sum y | 3.19E-01 3.19E-01 |
| Sum z | 2.84E-13 2.84E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.50E-01 7.67E-01 |
| Weighted Average x | -4.05E-02 -4.05E-02 |
| Weighted Average y | -1.00E-02 -9.98E-03 |
| Weighted Average z | -2.53E-15 -2.18E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.14E-01 1.39E-01 |
| Sum x | 5.35E-01 5.35E-01 |
| Sum y | 3.75E-01 3.75E-01 |
| Sum z | 6.78E-13 6.78E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.63E-03 1.74E-03 |
| Weighted Average x | -4.05E-02 -4.05E-02 |
| Weighted Average y | -1.00E-02 -9.95E-03 |
| Weighted Average z | -2.90E-15 -2.56E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.42E-03 4.76E-03 |
| Sum x | 5.31E-01 5.31E-01 |
| Sum y | 3.72E-01 3.72E-01 |
| Sum z | 6.15E-13 6.15E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.47E-05 3.68E-05 |
| Weighted Average x | -4.05E-02 -4.05E-02 |
| Weighted Average y | -1.00E-02 -9.95E-03 |
| Weighted Average z | -2.96E-15 -2.62E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 22 SIMULATION TIME = 2.20000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.21E-04 3.32E-04 |
| Sum x | 5.30E-01 5.30E-01 |
| Sum y | 3.72E-01 3.72E-01 |
| Sum z | 6.12E-13 6.12E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.54E-01 7.69E-01 |
| Weighted Average x | -3.52E-02 -3.53E-02 |
| Weighted Average y | -1.25E-02 -1.25E-02 |
| Weighted Average z | -2.44E-14 -2.43E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.56E-01 1.64E-01 |
| Sum x | 3.63E-01 3.63E-01 |
| Sum y | 3.66E-01 3.66E-01 |
| Sum z | 5.83E-13 5.83E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.26E-03 1.33E-03 |
| Weighted Average x | -3.52E-02 -3.52E-02 |
| Weighted Average y | -1.25E-02 -1.24E-02 |
| Weighted Average z | -2.40E-14 -2.40E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.04E-02 6.61E-03 |
| Sum x | 3.58E-01 3.58E-01 |
| Sum y | 3.63E-01 3.63E-01 |
| Sum z | 5.93E-13 5.93E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.39E-05 2.50E-05 |
| Weighted Average x | -3.52E-02 -3.52E-02 |
| Weighted Average y | -1.25E-02 -1.24E-02 |
| Weighted Average z | -2.40E-14 -2.40E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 23 SIMULATION TIME = 2.30000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.55E-04 4.13E-04 |
| Sum x | 3.58E-01 3.58E-01 |
| Sum y | 3.63E-01 3.63E-01 |
| Sum z | 5.90E-13 5.90E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.64E-01 7.78E-01 |
| Weighted Average x | -2.90E-02 -2.91E-02 |
| Weighted Average y | -1.29E-02 -1.28E-02 |
| Weighted Average z | -2.98E-14 -3.04E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 5.78E-01 3.32E-01 |
| Sum x | 1.28E-01 1.28E-01 |
| Sum y | 2.69E-01 2.69E-01 |
| Sum z | 1.63E-13 1.63E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.42E-04 8.41E-04 |
| Weighted Average x | -2.90E-02 -2.90E-02 |
| Weighted Average y | -1.29E-02 -1.28E-02 |
| Weighted Average z | -2.90E-14 -2.96E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.93E-02 1.08E-02 |
| Sum x | 1.22E-01 1.22E-01 |
| Sum y | 2.65E-01 2.65E-01 |
| Sum z | 1.78E-13 1.78E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.12E-05 2.28E-05 |
| Weighted Average x | -2.90E-02 -2.90E-02 |
| Weighted Average y | -1.29E-02 -1.28E-02 |
| Weighted Average z | -2.90E-14 -2.96E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.20E-03 6.73E-04 |
| Sum x | 1.22E-01 1.22E-01 |
| Sum y | 2.65E-01 2.65E-01 |
| Sum z | 1.78E-13 1.78E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.63E-06 1.74E-06 |
| Weighted Average x | -2.90E-02 -2.90E-02 |
| Weighted Average y | -1.29E-02 -1.28E-02 |
| Weighted Average z | -2.91E-14 -2.97E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 24 SIMULATION TIME = 2.40000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.78E-05 3.23E-05 |
| Sum x | 1.22E-01 1.22E-01 |
| Sum y | 2.65E-01 2.65E-01 |
| Sum z | 1.78E-13 1.78E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.69E-01 7.82E-01 |
| Weighted Average x | -2.17E-02 -2.18E-02 |
| Weighted Average y | -1.13E-02 -1.13E-02 |
| Weighted Average z | -5.24E-15 -5.73E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.17E+00 7.67E-01 |
| Sum x | -1.54E-01 -1.54E-01 |
| Sum y | 9.74E-02 9.74E-02 |
| Sum z | -1.47E-13 -1.47E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.57E-04 8.68E-04 |
| Weighted Average x | -2.17E-02 -2.18E-02 |
| Weighted Average y | -1.13E-02 -1.13E-02 |
| Weighted Average z | -4.85E-15 -5.32E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.90E-02 2.60E-02 |
| Sum x | -1.61E-01 -1.61E-01 |
| Sum y | 9.25E-02 9.25E-02 |
| Sum z | -1.87E-13 -1.87E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.25E-05 3.47E-05 |
| Weighted Average x | -2.17E-02 -2.18E-02 |
| Weighted Average y | -1.13E-02 -1.13E-02 |
| Weighted Average z | -4.84E-15 -5.30E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.79E-03 1.86E-03 |
| Sum x | -1.62E-01 -1.62E-01 |
| Sum y | 9.23E-02 9.23E-02 |
| Sum z | -1.89E-13 -1.89E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.24E-06 2.35E-06 |
| Weighted Average x | -2.17E-02 -2.18E-02 |
| Weighted Average y | -1.13E-02 -1.13E-02 |
| Weighted Average z | -4.83E-15 -5.32E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 25 SIMULATION TIME = 2.50000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.74E-05 5.15E-05 |
| Sum x | -1.62E-01 -1.62E-01 |
| Sum y | 9.23E-02 9.23E-02 |
| Sum z | -1.89E-13 -1.89E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.58E-01 7.73E-01 |
| Weighted Average x | -1.36E-02 -1.37E-02 |
| Weighted Average y | -8.25E-03 -8.23E-03 |
| Weighted Average z | 3.23E-14 3.26E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 7.10E-01 5.50E-01 |
| Sum x | -4.41E-01 -4.41E-01 |
| Sum y | -1.14E-01 -1.14E-01 |
| Sum z | -5.97E-13 -5.97E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 4.51E-04 4.60E-04 |
| Weighted Average x | -1.36E-02 -1.37E-02 |
| Weighted Average y | -8.24E-03 -8.22E-03 |
| Weighted Average z | 3.17E-14 3.20E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.45E-02 1.11E-02 |
| Sum x | -4.45E-01 -4.45E-01 |
| Sum y | -1.18E-01 -1.18E-01 |
| Sum z | -6.20E-13 -6.20E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.51E-05 1.47E-05 |
| Weighted Average x | -1.36E-02 -1.37E-02 |
| Weighted Average y | -8.24E-03 -8.22E-03 |
| Weighted Average z | 3.18E-14 3.20E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.13E-04 6.92E-04 |
| Sum x | -4.46E-01 -4.46E-01 |
| Sum y | -1.18E-01 -1.18E-01 |
| Sum z | -6.20E-13 -6.20E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.40E-06 1.45E-06 |
| Weighted Average x | -1.36E-02 -1.37E-02 |
| Weighted Average y | -8.24E-03 -8.22E-03 |
| Weighted Average z | 3.18E-14 3.21E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 26 SIMULATION TIME = 2.60000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.18E-05 4.69E-05 |
| Sum x | -4.46E-01 -4.46E-01 |
| Sum y | -1.18E-01 -1.18E-01 |
| Sum z | -6.19E-13 -6.19E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.31E-01 7.52E-01 |
| Weighted Average x | -5.46E-03 -5.47E-03 |
| Weighted Average y | -4.06E-03 -4.04E-03 |
| Weighted Average z | 4.73E-14 4.79E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 4.96E-01 3.59E-01 |
| Sum x | -6.71E-01 -6.71E-01 |
| Sum y | -3.10E-01 -3.10E-01 |
| Sum z | -1.05E-12 -1.05E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.70E-03 2.81E-03 |
| Weighted Average x | -5.48E-03 -5.49E-03 |
| Weighted Average y | -4.05E-03 -4.04E-03 |
| Weighted Average z | 4.60E-14 4.66E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.20E-03 4.76E-03 |
| Sum x | -6.70E-01 -6.70E-01 |
| Sum y | -3.11E-01 -3.11E-01 |
| Sum z | -1.06E-12 -1.06E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.10E-05 8.27E-05 |
| Weighted Average x | -5.48E-03 -5.49E-03 |
| Weighted Average y | -4.05E-03 -4.04E-03 |
| Weighted Average z | 4.60E-14 4.66E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 27 SIMULATION TIME = 2.70000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.06E-04 6.29E-04 |
| Sum x | -6.70E-01 -6.70E-01 |
| Sum y | -3.11E-01 -3.11E-01 |
| Sum z | -1.06E-12 -1.06E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.79E-01 8.81E-01 |
| Weighted Average x | 2.15E-03 2.17E-03 |
| Weighted Average y | 7.90E-04 8.02E-04 |
| Weighted Average z | 2.69E-14 2.72E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.94E-01 2.29E-01 |
| Sum x | -8.43E-01 -8.43E-01 |
| Sum y | -4.71E-01 -4.71E-01 |
| Sum z | -1.21E-12 -1.21E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.34E-02 1.38E-02 |
| Weighted Average x | 2.12E-03 2.15E-03 |
| Weighted Average y | 7.89E-04 8.01E-04 |
| Weighted Average z | 2.63E-14 2.66E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.44E-03 2.57E-03 |
| Sum x | -8.41E-01 -8.41E-01 |
| Sum y | -4.70E-01 -4.70E-01 |
| Sum z | -1.17E-12 -1.17E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.91E-04 4.06E-04 |
| Weighted Average x | 2.12E-03 2.15E-03 |
| Weighted Average y | 7.89E-04 8.01E-04 |
| Weighted Average z | 2.64E-14 2.67E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 28 SIMULATION TIME = 2.80000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.24E-04 1.55E-04 |
| Sum x | -8.41E-01 -8.41E-01 |
| Sum y | -4.70E-01 -4.70E-01 |
| Sum z | -1.17E-12 -1.17E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.63E-01 7.77E-01 |
| Weighted Average x | 9.06E-03 9.10E-03 |
| Weighted Average y | 5.70E-03 5.70E-03 |
| Weighted Average z | -8.30E-15 -8.53E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.58E-01 1.28E-01 |
| Sum x | -9.55E-01 -9.55E-01 |
| Sum y | -5.52E-01 -5.52E-01 |
| Sum z | -1.04E-12 -1.04E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.43E-03 1.43E-03 |
| Weighted Average x | 9.05E-03 9.09E-03 |
| Weighted Average y | 5.69E-03 5.68E-03 |
| Weighted Average z | -7.96E-15 -8.18E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.35E-03 4.21E-03 |
| Sum x | -9.51E-01 -9.51E-01 |
| Sum y | -5.49E-01 -5.49E-01 |
| Sum z | -1.06E-12 -1.06E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.72E-05 2.74E-05 |
| Weighted Average x | 9.05E-03 9.09E-03 |
| Weighted Average y | 5.69E-03 5.68E-03 |
| Weighted Average z | -8.06E-15 -8.28E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 29 SIMULATION TIME = 2.90000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.27E-04 1.83E-04 |
| Sum x | -9.51E-01 -9.51E-01 |
| Sum y | -5.49E-01 -5.49E-01 |
| Sum z | -1.06E-12 -1.06E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.46E-01 7.64E-01 |
| Weighted Average x | 1.58E-02 1.59E-02 |
| Weighted Average y | 9.75E-03 9.72E-03 |
| Weighted Average z | -3.28E-14 -3.32E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.17E-01 9.03E-02 |
| Sum x | -1.06E+00 -1.06E+00 |
| Sum y | -5.72E-01 -5.72E-01 |
| Sum z | -1.19E-12 -1.19E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.74E-03 1.85E-03 |
| Weighted Average x | 1.58E-02 1.59E-02 |
| Weighted Average y | 9.73E-03 9.70E-03 |
| Weighted Average z | -3.20E-14 -3.24E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.78E-03 2.91E-03 |
| Sum x | -1.06E+00 -1.06E+00 |
| Sum y | -5.70E-01 -5.70E-01 |
| Sum z | -1.16E-12 -1.16E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 4.02E-05 4.13E-05 |
| Weighted Average x | 1.58E-02 1.59E-02 |
| Weighted Average y | 9.73E-03 9.70E-03 |
| Weighted Average z | -3.21E-14 -3.25E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 30 SIMULATION TIME = 3.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.57E-04 1.22E-04 |
| Sum x | -1.06E+00 -1.06E+00 |
| Sum y | -5.70E-01 -5.70E-01 |
| Sum z | -1.16E-12 -1.16E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.46E-01 7.63E-01 |
| Weighted Average x | 2.29E-02 2.29E-02 |
| Weighted Average y | 1.22E-02 1.21E-02 |
| Weighted Average z | -3.16E-14 -3.19E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.09E-01 8.15E-02 |
| Sum x | -1.18E+00 -1.18E+00 |
| Sum y | -5.33E-01 -5.33E-01 |
| Sum z | -1.60E-12 -1.60E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.71E-03 1.83E-03 |
| Weighted Average x | 2.29E-02 2.29E-02 |
| Weighted Average y | 1.22E-02 1.21E-02 |
| Weighted Average z | -3.10E-14 -3.12E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.46E-03 2.55E-03 |
| Sum x | -1.18E+00 -1.18E+00 |
| Sum y | -5.32E-01 -5.32E-01 |
| Sum z | -1.64E-12 -1.64E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.89E-05 4.07E-05 |
| Weighted Average x | 2.29E-02 2.29E-02 |
| Weighted Average y | 1.22E-02 1.21E-02 |
| Weighted Average z | -3.09E-14 -3.12E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 31 SIMULATION TIME = 3.10000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 8.77E-05 6.41E-05 |
| Sum x | -1.18E+00 -1.18E+00 |
| Sum y | -5.32E-01 -5.32E-01 |
| Sum z | -1.64E-12 -1.64E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.54E-01 7.69E-01 |
| Weighted Average x | 3.01E-02 3.01E-02 |
| Weighted Average y | 1.27E-02 1.27E-02 |
| Weighted Average z | -1.05E-14 -1.03E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.17E-01 8.62E-02 |
| Sum x | -1.28E+00 -1.28E+00 |
| Sum y | -4.37E-01 -4.37E-01 |
| Sum z | -2.03E-12 -2.03E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.30E-03 1.36E-03 |
| Weighted Average x | 3.00E-02 3.01E-02 |
| Weighted Average y | 1.27E-02 1.26E-02 |
| Weighted Average z | -1.06E-14 -1.04E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.18E-03 2.36E-03 |
| Sum x | -1.28E+00 -1.28E+00 |
| Sum y | -4.35E-01 -4.35E-01 |
| Sum z | -2.12E-12 -2.12E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.02E-05 2.09E-05 |
| Weighted Average x | 3.00E-02 3.01E-02 |
| Weighted Average y | 1.27E-02 1.26E-02 |
| Weighted Average z | -1.06E-14 -1.04E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 32 SIMULATION TIME = 3.20000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.85E-05 7.16E-05 |
| Sum x | -1.28E+00 -1.28E+00 |
| Sum y | -4.35E-01 -4.35E-01 |
| Sum z | -2.13E-12 -2.13E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.62E-01 7.76E-01 |
| Weighted Average x | 3.64E-02 3.65E-02 |
| Weighted Average y | 1.13E-02 1.13E-02 |
| Weighted Average z | 9.57E-15 9.89E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.24E-01 9.35E-02 |
| Sum x | -1.32E+00 -1.32E+00 |
| Sum y | -2.88E-01 -2.88E-01 |
| Sum z | -1.81E-12 -1.81E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.09E-03 1.10E-03 |
| Weighted Average x | 3.64E-02 3.64E-02 |
| Weighted Average y | 1.13E-02 1.13E-02 |
| Weighted Average z | 9.25E-15 9.53E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.69E-03 3.56E-03 |
| Sum x | -1.31E+00 -1.31E+00 |
| Sum y | -2.86E-01 -2.86E-01 |
| Sum z | -1.78E-12 -1.78E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.13E-05 1.14E-05 |
| Weighted Average x | 3.64E-02 3.64E-02 |
| Weighted Average y | 1.13E-02 1.13E-02 |
| Weighted Average z | 9.30E-15 9.57E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 33 SIMULATION TIME = 3.30000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.24E-04 9.11E-05 |
| Sum x | -1.31E+00 -1.31E+00 |
| Sum y | -2.86E-01 -2.86E-01 |
| Sum z | -1.78E-12 -1.78E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.65E-01 7.78E-01 |
| Weighted Average x | 4.11E-02 4.12E-02 |
| Weighted Average y | 8.44E-03 8.41E-03 |
| Weighted Average z | 1.69E-14 1.71E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.45E-01 1.12E-01 |
| Sum x | -1.25E+00 -1.25E+00 |
| Sum y | -1.09E-01 -1.09E-01 |
| Sum z | -1.16E-12 -1.16E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.08E-03 1.07E-03 |
| Weighted Average x | 4.10E-02 4.11E-02 |
| Weighted Average y | 8.43E-03 8.40E-03 |
| Weighted Average z | 1.65E-14 1.67E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.33E-03 4.86E-03 |
| Sum x | -1.24E+00 -1.24E+00 |
| Sum y | -1.07E-01 -1.07E-01 |
| Sum z | -1.23E-12 -1.23E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.53E-05 1.57E-05 |
| Weighted Average x | 4.10E-02 4.11E-02 |
| Weighted Average y | 8.43E-03 8.40E-03 |
| Weighted Average z | 1.65E-14 1.67E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 34 SIMULATION TIME = 3.40000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.16E-04 1.61E-04 |
| Sum x | -1.24E+00 -1.24E+00 |
| Sum y | -1.07E-01 -1.07E-01 |
| Sum z | -1.23E-12 -1.23E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.62E-01 7.76E-01 |
| Weighted Average x | 4.35E-02 4.36E-02 |
| Weighted Average y | 4.35E-03 4.34E-03 |
| Weighted Average z | 1.08E-14 1.08E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.69E-01 1.30E-01 |
| Sum x | -1.10E+00 -1.10E+00 |
| Sum y | 6.37E-02 6.37E-02 |
| Sum z | -4.92E-13 -4.92E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.07E-03 1.08E-03 |
| Weighted Average x | 4.35E-02 4.35E-02 |
| Weighted Average y | 4.35E-03 4.33E-03 |
| Weighted Average z | 1.07E-14 1.07E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.81E-03 5.21E-03 |
| Sum x | -1.09E+00 -1.09E+00 |
| Sum y | 6.41E-02 6.41E-02 |
| Sum z | -5.37E-13 -5.37E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 7.98E-06 7.90E-06 |
| Weighted Average x | 4.35E-02 4.35E-02 |
| Weighted Average y | 4.35E-03 4.33E-03 |
| Weighted Average z | 1.07E-14 1.07E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 35 SIMULATION TIME = 3.50000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.80E-04 2.10E-04 |
| Sum x | -1.09E+00 -1.09E+00 |
| Sum y | 6.41E-02 6.41E-02 |
| Sum z | -5.34E-13 -5.34E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.57E-01 7.73E-01 |
| Weighted Average x | 4.38E-02 4.38E-02 |
| Weighted Average y | -3.63E-04 -3.63E-04 |
| Weighted Average z | -1.81E-15 -1.79E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.71E-01 1.31E-01 |
| Sum x | -9.25E-01 -9.25E-01 |
| Sum y | 2.05E-01 2.05E-01 |
| Sum z | -2.36E-13 -2.36E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.19E-03 1.24E-03 |
| Weighted Average x | 4.37E-02 4.37E-02 |
| Weighted Average y | -3.58E-04 -3.58E-04 |
| Weighted Average z | -1.78E-15 -1.81E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.18E-03 4.66E-03 |
| Sum x | -9.18E-01 -9.18E-01 |
| Sum y | 2.04E-01 2.04E-01 |
| Sum z | -2.13E-13 -2.13E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.23E-05 1.33E-05 |
| Weighted Average x | 4.37E-02 4.37E-02 |
| Weighted Average y | -3.58E-04 -3.58E-04 |
| Weighted Average z | -1.82E-15 -1.81E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 36 SIMULATION TIME = 3.60000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.94E-04 1.43E-04 |
| Sum x | -9.17E-01 -9.17E-01 |
| Sum y | 2.04E-01 2.04E-01 |
| Sum z | -2.16E-13 -2.16E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.55E-01 7.71E-01 |
| Weighted Average x | 4.21E-02 4.21E-02 |
| Weighted Average y | -4.97E-03 -4.95E-03 |
| Weighted Average z | -1.26E-14 -1.26E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.66E-01 1.25E-01 |
| Sum x | -7.59E-01 -7.59E-01 |
| Sum y | 3.04E-01 3.04E-01 |
| Sum z | -2.09E-14 -2.09E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.26E-03 1.33E-03 |
| Weighted Average x | 4.20E-02 4.20E-02 |
| Weighted Average y | -4.95E-03 -4.93E-03 |
| Weighted Average z | -1.24E-14 -1.25E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.27E-03 4.65E-03 |
| Sum x | -7.53E-01 -7.53E-01 |
| Sum y | 3.02E-01 3.02E-01 |
| Sum z | -2.02E-13 -2.02E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.75E-05 1.89E-05 |
| Weighted Average x | 4.20E-02 4.20E-02 |
| Weighted Average y | -4.95E-03 -4.93E-03 |
| Weighted Average z | -1.23E-14 -1.24E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 37 SIMULATION TIME = 3.70000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.06E-04 1.51E-04 |
| Sum x | -7.53E-01 -7.53E-01 |
| Sum y | 3.02E-01 3.02E-01 |
| Sum z | -1.96E-13 -1.96E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.58E-01 7.73E-01 |
| Weighted Average x | 3.87E-02 3.87E-02 |
| Weighted Average y | -8.65E-03 -8.61E-03 |
| Weighted Average z | -1.34E-14 -1.35E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.96E-01 1.43E-01 |
| Sum x | -5.71E-01 -5.71E-01 |
| Sum y | 3.47E-01 3.47E-01 |
| Sum z | -5.14E-13 -5.14E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.12E-03 1.16E-03 |
| Weighted Average x | 3.86E-02 3.86E-02 |
| Weighted Average y | -8.63E-03 -8.59E-03 |
| Weighted Average z | -1.31E-14 -1.33E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.75E-03 5.59E-03 |
| Sum x | -5.65E-01 -5.65E-01 |
| Sum y | 3.45E-01 3.45E-01 |
| Sum z | -5.33E-13 -5.33E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.35E-05 1.39E-05 |
| Weighted Average x | 3.86E-02 3.86E-02 |
| Weighted Average y | -8.63E-03 -8.59E-03 |
| Weighted Average z | -1.31E-14 -1.32E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 38 SIMULATION TIME = 3.80000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.66E-04 1.89E-04 |
| Sum x | -5.65E-01 -5.65E-01 |
| Sum y | 3.45E-01 3.45E-01 |
| Sum z | -5.29E-13 -5.29E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.62E-01 7.76E-01 |
| Weighted Average x | 3.37E-02 3.38E-02 |
| Weighted Average y | -1.08E-02 -1.08E-02 |
| Weighted Average z | -1.43E-15 -1.59E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.23E-01 2.16E-01 |
| Sum x | -3.41E-01 -3.41E-01 |
| Sum y | 3.21E-01 3.21E-01 |
| Sum z | -8.69E-13 -8.69E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.25E-04 9.32E-04 |
| Weighted Average x | 3.37E-02 3.37E-02 |
| Weighted Average y | -1.08E-02 -1.08E-02 |
| Weighted Average z | -1.27E-15 -1.38E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.17E-02 7.79E-03 |
| Sum x | -3.34E-01 -3.34E-01 |
| Sum y | 3.17E-01 3.17E-01 |
| Sum z | -9.15E-13 -9.15E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.43E-05 1.45E-05 |
| Weighted Average x | 3.37E-02 3.37E-02 |
| Weighted Average y | -1.08E-02 -1.08E-02 |
| Weighted Average z | -1.32E-15 -1.50E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 39 SIMULATION TIME = 3.90000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.50E-04 1.65E-04 |
| Sum x | -3.34E-01 -3.34E-01 |
| Sum y | 3.17E-01 3.17E-01 |
| Sum z | -9.14E-13 -9.14E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.61E-01 7.76E-01 |
| Weighted Average x | 2.74E-02 2.75E-02 |
| Weighted Average y | -1.13E-02 -1.13E-02 |
| Weighted Average z | 1.47E-14 1.48E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 7.21E-01 3.97E-01 |
| Sum x | -7.68E-02 -7.68E-02 |
| Sum y | 2.23E-01 2.23E-01 |
| Sum z | -1.13E-12 -1.13E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.02E-04 8.04E-04 |
| Weighted Average x | 2.74E-02 2.75E-02 |
| Weighted Average y | -1.13E-02 -1.13E-02 |
| Weighted Average z | 1.44E-14 1.44E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.46E-02 1.35E-02 |
| Sum x | -7.08E-02 -7.08E-02 |
| Sum y | 2.20E-01 2.20E-01 |
| Sum z | -7.14E-13 -7.14E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.58E-05 1.60E-05 |
| Weighted Average x | 2.74E-02 2.75E-02 |
| Weighted Average y | -1.13E-02 -1.13E-02 |
| Weighted Average z | 1.44E-14 1.45E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.17E-03 6.35E-04 |
| Sum x | -7.07E-02 -7.07E-02 |
| Sum y | 2.20E-01 2.20E-01 |
| Sum z | -7.10E-13 -7.10E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.31E-06 1.33E-06 |
| Weighted Average x | 2.74E-02 2.75E-02 |
| Weighted Average y | -1.13E-02 -1.13E-02 |
| Weighted Average z | 1.44E-14 1.45E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 40 SIMULATION TIME = 4.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.47E-05 3.49E-05 |
| Sum x | -7.07E-02 -7.07E-02 |
| Sum y | 2.20E-01 2.20E-01 |
| Sum z | -7.10E-13 -7.10E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.56E-01 7.71E-01 |
| Weighted Average x | 2.03E-02 2.03E-02 |
| Weighted Average y | -1.01E-02 -1.01E-02 |
| Weighted Average z | 2.12E-14 2.14E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.45E+00 7.18E-01 |
| Sum x | 1.83E-01 1.83E-01 |
| Sum y | 7.89E-02 7.89E-02 |
| Sum z | -8.05E-13 -8.05E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.06E-04 8.37E-04 |
| Weighted Average x | 2.03E-02 2.03E-02 |
| Weighted Average y | -1.01E-02 -1.01E-02 |
| Weighted Average z | 2.08E-14 2.10E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.66E-02 1.80E-02 |
| Sum x | 1.88E-01 1.88E-01 |
| Sum y | 7.52E-02 7.52E-02 |
| Sum z | -6.24E-13 -6.24E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.91E-05 1.91E-05 |
| Weighted Average x | 2.03E-02 2.03E-02 |
| Weighted Average y | -1.01E-02 -1.01E-02 |
| Weighted Average z | 2.08E-14 2.10E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.70E-03 8.30E-04 |
| Sum x | 1.88E-01 1.88E-01 |
| Sum y | 7.52E-02 7.52E-02 |
| Sum z | -6.24E-13 -6.24E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.44E-06 1.44E-06 |
| Weighted Average x | 2.03E-02 2.03E-02 |
| Weighted Average y | -1.01E-02 -1.01E-02 |
| Weighted Average z | 2.08E-14 2.10E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 41 SIMULATION TIME = 4.10000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.15E-04 5.58E-05 |
| Sum x | 1.88E-01 1.88E-01 |
| Sum y | 7.52E-02 7.52E-02 |
| Sum z | -6.24E-13 -6.24E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.47E-01 7.64E-01 |
| Weighted Average x | 1.28E-02 1.28E-02 |
| Weighted Average y | -7.48E-03 -7.46E-03 |
| Weighted Average z | 1.02E-14 1.03E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 6.57E-01 4.76E-01 |
| Sum x | 4.07E-01 4.07E-01 |
| Sum y | -8.43E-02 -8.43E-02 |
| Sum z | -5.38E-13 -5.38E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.23E-03 1.30E-03 |
| Weighted Average x | 1.28E-02 1.28E-02 |
| Weighted Average y | -7.47E-03 -7.45E-03 |
| Weighted Average z | 9.98E-15 1.01E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.90E-03 7.07E-03 |
| Sum x | 4.10E-01 4.10E-01 |
| Sum y | -8.71E-02 -8.71E-02 |
| Sum z | -5.94E-13 -5.94E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.38E-05 3.41E-05 |
| Weighted Average x | 1.28E-02 1.28E-02 |
| Weighted Average y | -7.47E-03 -7.45E-03 |
| Weighted Average z | 9.97E-15 1.01E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 42 SIMULATION TIME = 4.20000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.69E-04 2.56E-04 |
| Sum x | 4.10E-01 4.10E-01 |
| Sum y | -8.72E-02 -8.72E-02 |
| Sum z | -5.93E-13 -5.93E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.37E-01 7.56E-01 |
| Weighted Average x | 5.58E-03 5.59E-03 |
| Weighted Average y | -3.68E-03 -3.67E-03 |
| Weighted Average z | -1.17E-14 -1.18E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.70E-01 2.90E-01 |
| Sum x | 5.84E-01 5.84E-01 |
| Sum y | -2.39E-01 -2.39E-01 |
| Sum z | -3.71E-13 -3.71E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.92E-03 1.99E-03 |
| Weighted Average x | 5.60E-03 5.60E-03 |
| Weighted Average y | -3.68E-03 -3.66E-03 |
| Weighted Average z | -1.16E-14 -1.16E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.53E-03 2.62E-03 |
| Sum x | 5.85E-01 5.85E-01 |
| Sum y | -2.41E-01 -2.41E-01 |
| Sum z | -3.49E-13 -3.49E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.94E-05 6.03E-05 |
| Weighted Average x | 5.59E-03 5.60E-03 |
| Weighted Average y | -3.68E-03 -3.66E-03 |
| Weighted Average z | -1.16E-14 -1.16E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 43 SIMULATION TIME = 4.30000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.62E-04 1.92E-04 |
| Sum x | 5.85E-01 5.85E-01 |
| Sum y | -2.41E-01 -2.41E-01 |
| Sum z | -3.49E-13 -3.49E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.84E-01 7.95E-01 |
| Weighted Average x | -1.30E-03 -1.31E-03 |
| Weighted Average y | 7.23E-04 7.23E-04 |
| Weighted Average z | -8.26E-15 -8.35E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.52E-01 2.03E-01 |
| Sum x | 7.50E-01 7.50E-01 |
| Sum y | -3.82E-01 -3.82E-01 |
| Sum z | -1.84E-13 -1.84E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.57E-03 6.63E-03 |
| Weighted Average x | -1.29E-03 -1.29E-03 |
| Weighted Average y | 7.16E-04 7.17E-04 |
| Weighted Average z | -8.17E-15 -8.26E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.11E-03 1.49E-03 |
| Sum x | 7.49E-01 7.49E-01 |
| Sum y | -3.82E-01 -3.82E-01 |
| Sum z | -1.75E-13 -1.75E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.36E-05 6.51E-05 |
| Weighted Average x | -1.29E-03 -1.30E-03 |
| Weighted Average y | 7.16E-04 7.17E-04 |
| Weighted Average z | -8.17E-15 -8.26E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 44 SIMULATION TIME = 4.40000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.33E-04 1.76E-04 |
| Sum x | 7.49E-01 7.49E-01 |
| Sum y | -3.82E-01 -3.82E-01 |
| Sum z | -1.75E-13 -1.75E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.46E-01 7.63E-01 |
| Weighted Average x | -8.08E-03 -8.09E-03 |
| Weighted Average y | 5.00E-03 4.98E-03 |
| Weighted Average z | 1.52E-14 1.55E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.49E-01 1.24E-01 |
| Sum x | 8.89E-01 8.89E-01 |
| Sum y | -4.66E-01 -4.66E-01 |
| Sum z | -1.26E-13 -1.26E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.11E-03 2.24E-03 |
| Weighted Average x | -8.07E-03 -8.09E-03 |
| Weighted Average y | 4.98E-03 4.96E-03 |
| Weighted Average z | 1.48E-14 1.50E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.94E-03 2.30E-03 |
| Sum x | 8.87E-01 8.87E-01 |
| Sum y | -4.65E-01 -4.65E-01 |
| Sum z | -1.36E-13 -1.36E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 4.11E-05 4.29E-05 |
| Weighted Average x | -8.07E-03 -8.09E-03 |
| Weighted Average y | 4.98E-03 4.97E-03 |
| Weighted Average z | 1.50E-14 1.52E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 45 SIMULATION TIME = 4.50000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.79E-04 1.43E-04 |
| Sum x | 8.87E-01 8.87E-01 |
| Sum y | -4.65E-01 -4.65E-01 |
| Sum z | -1.36E-13 -1.36E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.48E-01 7.65E-01 |
| Weighted Average x | -1.50E-02 -1.50E-02 |
| Weighted Average y | 8.43E-03 8.41E-03 |
| Weighted Average z | 1.55E-14 1.56E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.05E-01 8.89E-02 |
| Sum x | 1.02E+00 1.02E+00 |
| Sum y | -4.95E-01 -4.95E-01 |
| Sum z | -2.75E-14 -2.75E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.60E-03 1.69E-03 |
| Weighted Average x | -1.50E-02 -1.50E-02 |
| Weighted Average y | 8.41E-03 8.39E-03 |
| Weighted Average z | 1.51E-14 1.52E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.31E-03 3.51E-03 |
| Sum x | 1.02E+00 1.02E+00 |
| Sum y | -4.93E-01 -4.93E-01 |
| Sum z | -7.47E-14 -7.47E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.07E-05 3.11E-05 |
| Weighted Average x | -1.50E-02 -1.50E-02 |
| Weighted Average y | 8.42E-03 8.39E-03 |
| Weighted Average z | 1.51E-14 1.52E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 46 SIMULATION TIME = 4.60000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.27E-04 1.85E-04 |
| Sum x | 1.02E+00 1.02E+00 |
| Sum y | -4.93E-01 -4.93E-01 |
| Sum z | -7.52E-14 -7.52E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.54E-01 7.70E-01 |
| Weighted Average x | -2.20E-02 -2.20E-02 |
| Weighted Average y | 1.05E-02 1.05E-02 |
| Weighted Average z | -3.87E-15 -4.08E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 8.33E-02 7.08E-02 |
| Sum x | 1.13E+00 1.13E+00 |
| Sum y | -4.61E-01 -4.61E-01 |
| Sum z | -5.16E-14 -5.16E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.18E-03 1.23E-03 |
| Weighted Average x | -2.19E-02 -2.20E-02 |
| Weighted Average y | 1.05E-02 1.05E-02 |
| Weighted Average z | -3.74E-15 -3.99E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.89E-03 3.28E-03 |
| Sum x | 1.12E+00 1.12E+00 |
| Sum y | -4.59E-01 -4.59E-01 |
| Sum z | -6.11E-14 -6.11E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.91E-05 1.93E-05 |
| Weighted Average x | -2.19E-02 -2.20E-02 |
| Weighted Average y | 1.05E-02 1.05E-02 |
| Weighted Average z | -3.69E-15 -3.90E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 47 SIMULATION TIME = 4.70000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.44E-04 1.19E-04 |
| Sum x | 1.12E+00 1.12E+00 |
| Sum y | -4.59E-01 -4.59E-01 |
| Sum z | -6.07E-14 -6.07E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.59E-01 7.73E-01 |
| Weighted Average x | -2.85E-02 -2.85E-02 |
| Weighted Average y | 1.11E-02 1.11E-02 |
| Weighted Average z | -1.99E-14 -2.00E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 8.49E-02 7.28E-02 |
| Sum x | 1.18E+00 1.18E+00 |
| Sum y | -3.69E-01 -3.69E-01 |
| Sum z | -7.44E-14 -7.44E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.07E-03 1.09E-03 |
| Weighted Average x | -2.85E-02 -2.85E-02 |
| Weighted Average y | 1.11E-02 1.10E-02 |
| Weighted Average z | -1.96E-14 -1.97E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.36E-03 3.77E-03 |
| Sum x | 1.18E+00 1.18E+00 |
| Sum y | -3.67E-01 -3.67E-01 |
| Sum z | -1.18E-13 -1.18E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.18E-05 1.16E-05 |
| Weighted Average x | -2.85E-02 -2.85E-02 |
| Weighted Average y | 1.11E-02 1.10E-02 |
| Weighted Average z | -1.96E-14 -1.97E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 48 SIMULATION TIME = 4.80000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.37E-04 1.17E-04 |
| Sum x | 1.18E+00 1.18E+00 |
| Sum y | -3.66E-01 -3.66E-01 |
| Sum z | -1.19E-13 -1.19E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.61E-01 7.75E-01 |
| Weighted Average x | -3.40E-02 -3.41E-02 |
| Weighted Average y | 1.01E-02 1.00E-02 |
| Weighted Average z | -2.41E-14 -2.42E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.08E-01 8.98E-02 |
| Sum x | 1.18E+00 1.18E+00 |
| Sum y | -2.34E-01 -2.34E-01 |
| Sum z | -1.62E-13 -1.62E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.03E-03 1.04E-03 |
| Weighted Average x | -3.40E-02 -3.40E-02 |
| Weighted Average y | 1.00E-02 1.00E-02 |
| Weighted Average z | -2.35E-14 -2.36E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.36E-03 4.48E-03 |
| Sum x | 1.17E+00 1.17E+00 |
| Sum y | -2.32E-01 -2.32E-01 |
| Sum z | -2.71E-13 -2.71E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.09E-05 1.09E-05 |
| Weighted Average x | -3.40E-02 -3.40E-02 |
| Weighted Average y | 1.01E-02 1.00E-02 |
| Weighted Average z | -2.36E-14 -2.37E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 49 SIMULATION TIME = 4.90000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.48E-04 1.22E-04 |
| Sum x | 1.17E+00 1.17E+00 |
| Sum y | -2.32E-01 -2.32E-01 |
| Sum z | -2.68E-13 -2.68E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.74E-01 |
| Weighted Average x | -3.81E-02 -3.81E-02 |
| Weighted Average y | 7.58E-03 7.55E-03 |
| Weighted Average z | -1.27E-14 -1.29E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.34E-01 1.07E-01 |
| Sum x | 1.11E+00 1.11E+00 |
| Sum y | -8.09E-02 -8.09E-02 |
| Sum z | -2.46E-13 -2.46E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.05E-03 1.08E-03 |
| Weighted Average x | -3.81E-02 -3.81E-02 |
| Weighted Average y | 7.56E-03 7.53E-03 |
| Weighted Average z | -1.25E-14 -1.26E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.58E-03 5.23E-03 |
| Sum x | 1.10E+00 1.10E+00 |
| Sum y | -7.99E-02 -7.99E-02 |
| Sum z | -2.67E-13 -2.67E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.75E-06 8.72E-06 |
| Weighted Average x | -3.81E-02 -3.81E-02 |
| Weighted Average y | 7.56E-03 7.53E-03 |
| Weighted Average z | -1.25E-14 -1.26E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 50 SIMULATION TIME = 5.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.22E-04 1.74E-04 |
| Sum x | 1.10E+00 1.10E+00 |
| Sum y | -7.99E-02 -7.99E-02 |
| Sum z | -2.66E-13 -2.66E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.58E-01 7.73E-01 |
| Weighted Average x | -4.05E-02 -4.05E-02 |
| Weighted Average y | 3.97E-03 3.95E-03 |
| Weighted Average z | 6.82E-15 6.85E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.54E-01 1.17E-01 |
| Sum x | 1.01E+00 1.01E+00 |
| Sum y | 6.56E-02 6.56E-02 |
| Sum z | -2.16E-13 -2.16E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.12E-03 1.16E-03 |
| Weighted Average x | -4.04E-02 -4.04E-02 |
| Weighted Average y | 3.97E-03 3.95E-03 |
| Weighted Average z | 6.68E-15 6.72E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.21E-03 4.68E-03 |
| Sum x | 1.00E+00 1.00E+00 |
| Sum y | 6.57E-02 6.57E-02 |
| Sum z | -2.30E-13 -2.30E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.32E-06 9.94E-06 |
| Weighted Average x | -4.04E-02 -4.04E-02 |
| Weighted Average y | 3.97E-03 3.95E-03 |
| Weighted Average z | 6.68E-15 6.74E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 51 SIMULATION TIME = 5.10000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.16E-04 1.60E-04 |
| Sum x | 1.00E+00 1.00E+00 |
| Sum y | 6.56E-02 6.56E-02 |
| Sum z | -2.32E-13 -2.32E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.59E-01 7.73E-01 |
| Weighted Average x | -4.10E-02 -4.10E-02 |
| Weighted Average y | -1.82E-04 -1.81E-04 |
| Weighted Average z | 2.06E-14 2.09E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.69E-01 1.22E-01 |
| Sum x | 8.82E-01 8.82E-01 |
| Sum y | 1.88E-01 1.88E-01 |
| Sum z | -2.28E-13 -2.28E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.12E-03 1.16E-03 |
| Weighted Average x | -4.10E-02 -4.10E-02 |
| Weighted Average y | -1.78E-04 -1.77E-04 |
| Weighted Average z | 2.02E-14 2.04E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.51E-03 5.31E-03 |
| Sum x | 8.76E-01 8.76E-01 |
| Sum y | 1.87E-01 1.87E-01 |
| Sum z | -2.88E-13 -2.88E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.95E-06 9.56E-06 |
| Weighted Average x | -4.10E-02 -4.10E-02 |
| Weighted Average y | -1.78E-04 -1.77E-04 |
| Weighted Average z | 2.02E-14 2.05E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 52 SIMULATION TIME = 5.20000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.05E-04 2.13E-04 |
| Sum x | 8.75E-01 8.75E-01 |
| Sum y | 1.87E-01 1.87E-01 |
| Sum z | -2.91E-13 -2.91E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.74E-01 |
| Weighted Average x | -3.97E-02 -3.97E-02 |
| Weighted Average y | -4.19E-03 -4.17E-03 |
| Weighted Average z | 1.55E-14 1.59E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.98E-01 1.34E-01 |
| Sum x | 7.20E-01 7.20E-01 |
| Sum y | 2.72E-01 2.72E-01 |
| Sum z | -4.69E-13 -4.69E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.06E-03 1.09E-03 |
| Weighted Average x | -3.97E-02 -3.97E-02 |
| Weighted Average y | -4.18E-03 -4.16E-03 |
| Weighted Average z | 1.50E-14 1.53E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.10E-03 6.04E-03 |
| Sum x | 7.13E-01 7.13E-01 |
| Sum y | 2.70E-01 2.70E-01 |
| Sum z | -4.31E-13 -4.31E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.21E-06 8.31E-06 |
| Weighted Average x | -3.97E-02 -3.97E-02 |
| Weighted Average y | -4.18E-03 -4.16E-03 |
| Weighted Average z | 1.50E-14 1.53E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 53 SIMULATION TIME = 5.30000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.71E-04 2.43E-04 |
| Sum x | 7.13E-01 7.13E-01 |
| Sum y | 2.70E-01 2.70E-01 |
| Sum z | -4.29E-13 -4.29E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.75E-01 |
| Weighted Average x | -3.66E-02 -3.66E-02 |
| Weighted Average y | -7.43E-03 -7.40E-03 |
| Weighted Average z | -6.97E-15 -6.90E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.54E-01 1.67E-01 |
| Sum x | 5.15E-01 5.15E-01 |
| Sum y | 3.01E-01 3.01E-01 |
| Sum z | -4.82E-13 -4.82E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.83E-04 9.98E-04 |
| Weighted Average x | -3.65E-02 -3.66E-02 |
| Weighted Average y | -7.42E-03 -7.39E-03 |
| Weighted Average z | -6.96E-15 -6.92E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.04E-02 6.80E-03 |
| Sum x | 5.08E-01 5.08E-01 |
| Sum y | 2.99E-01 2.99E-01 |
| Sum z | -4.40E-13 -4.40E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.71E-06 9.61E-06 |
| Weighted Average x | -3.65E-02 -3.66E-02 |
| Weighted Average y | -7.42E-03 -7.39E-03 |
| Weighted Average z | -6.95E-15 -6.88E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 54 SIMULATION TIME = 5.40000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.01E-04 2.58E-04 |
| Sum x | 5.08E-01 5.08E-01 |
| Sum y | 2.99E-01 2.99E-01 |
| Sum z | -4.36E-13 -4.36E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.74E-01 |
| Weighted Average x | -3.19E-02 -3.19E-02 |
| Weighted Average y | -9.45E-03 -9.42E-03 |
| Weighted Average z | -2.61E-14 -2.64E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.73E-01 2.32E-01 |
| Sum x | 2.83E-01 2.83E-01 |
| Sum y | 2.72E-01 2.72E-01 |
| Sum z | -4.26E-13 -4.26E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.15E-04 9.32E-04 |
| Weighted Average x | -3.18E-02 -3.19E-02 |
| Weighted Average y | -9.44E-03 -9.40E-03 |
| Weighted Average z | -2.55E-14 -2.57E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.23E-02 7.55E-03 |
| Sum x | 2.78E-01 2.78E-01 |
| Sum y | 2.69E-01 2.69E-01 |
| Sum z | -4.23E-13 -4.23E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.19E-05 1.19E-05 |
| Weighted Average x | -3.18E-02 -3.19E-02 |
| Weighted Average y | -9.44E-03 -9.40E-03 |
| Weighted Average z | -2.55E-14 -2.58E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 55 SIMULATION TIME = 5.50000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.85E-04 3.54E-04 |
| Sum x | 2.78E-01 2.78E-01 |
| Sum y | 2.69E-01 2.69E-01 |
| Sum z | -4.24E-13 -4.24E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.57E-01 7.72E-01 |
| Weighted Average x | -2.59E-02 -2.60E-02 |
| Weighted Average y | -1.00E-02 -9.97E-03 |
| Weighted Average z | -2.31E-14 -2.36E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 6.64E-01 3.70E-01 |
| Sum x | 4.96E-02 4.96E-02 |
| Sum y | 1.89E-01 1.89E-01 |
| Sum z | -2.65E-13 -2.65E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.02E-04 9.34E-04 |
| Weighted Average x | -2.59E-02 -2.60E-02 |
| Weighted Average y | -9.99E-03 -9.96E-03 |
| Weighted Average z | -2.26E-14 -2.30E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.59E-02 8.79E-03 |
| Sum x | 4.51E-02 4.51E-02 |
| Sum y | 1.86E-01 1.86E-01 |
| Sum z | -2.48E-13 -2.48E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.62E-05 1.61E-05 |
| Weighted Average x | -2.59E-02 -2.60E-02 |
| Weighted Average y | -9.99E-03 -9.96E-03 |
| Weighted Average z | -2.25E-14 -2.29E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 56 SIMULATION TIME = 5.60000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 8.50E-04 4.62E-04 |
| Sum x | 4.50E-02 4.50E-02 |
| Sum y | 1.86E-01 1.86E-01 |
| Sum z | -2.48E-13 -2.48E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.53E-01 7.69E-01 |
| Weighted Average x | -1.94E-02 -1.94E-02 |
| Weighted Average y | -9.03E-03 -9.00E-03 |
| Weighted Average z | 1.39E-15 1.16E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 9.16E-01 6.44E-01 |
| Sum x | -1.68E-01 -1.68E-01 |
| Sum y | 6.94E-02 6.94E-02 |
| Sum z | -6.99E-14 -6.99E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.52E-04 1.00E-03 |
| Weighted Average x | -1.94E-02 -1.94E-02 |
| Weighted Average y | -9.02E-03 -8.99E-03 |
| Weighted Average z | 1.45E-15 1.23E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.37E-02 1.67E-02 |
| Sum x | -1.71E-01 -1.71E-01 |
| Sum y | 6.66E-02 6.66E-02 |
| Sum z | -2.77E-14 -2.77E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.27E-05 2.29E-05 |
| Weighted Average x | -1.94E-02 -1.94E-02 |
| Weighted Average y | -9.02E-03 -8.99E-03 |
| Weighted Average z | 1.44E-15 1.24E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.97E-03 1.39E-03 |
| Sum x | -1.72E-01 -1.72E-01 |
| Sum y | 6.65E-02 6.65E-02 |
| Sum z | -2.83E-14 -2.83E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.44E-06 1.43E-06 |
| Weighted Average x | -1.94E-02 -1.94E-02 |
| Weighted Average y | -9.02E-03 -8.99E-03 |
| Weighted Average z | 1.51E-15 1.28E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 57 SIMULATION TIME = 5.70000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.00E-04 7.09E-05 |
| Sum x | -1.72E-01 -1.72E-01 |
| Sum y | 6.65E-02 6.65E-02 |
| Sum z | -2.82E-14 -2.82E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.51E-01 7.67E-01 |
| Weighted Average x | -1.26E-02 -1.27E-02 |
| Weighted Average y | -6.69E-03 -6.67E-03 |
| Weighted Average z | 2.82E-14 2.85E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 5.93E-01 4.56E-01 |
| Sum x | -3.62E-01 -3.62E-01 |
| Sum y | -6.93E-02 -6.93E-02 |
| Sum z | 8.86E-14 8.86E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.05E-04 9.54E-04 |
| Weighted Average x | -1.26E-02 -1.27E-02 |
| Weighted Average y | -6.68E-03 -6.66E-03 |
| Weighted Average z | 2.74E-14 2.77E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.30E-03 6.86E-03 |
| Sum x | -3.64E-01 -3.64E-01 |
| Sum y | -7.13E-02 -7.13E-02 |
| Sum z | 1.10E-13 1.10E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.56E-05 2.57E-05 |
| Weighted Average x | -1.26E-02 -1.27E-02 |
| Weighted Average y | -6.68E-03 -6.66E-03 |
| Weighted Average z | 2.76E-14 2.79E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 58 SIMULATION TIME = 5.80000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.10E-03 8.25E-04 |
| Sum x | -3.65E-01 -3.65E-01 |
| Sum y | -7.14E-02 -7.14E-02 |
| Sum z | 1.11E-13 1.11E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.52E-01 7.68E-01 |
| Weighted Average x | -5.90E-03 -5.91E-03 |
| Weighted Average y | -3.33E-03 -3.32E-03 |
| Weighted Average z | 3.28E-14 3.32E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 4.77E-01 3.50E-01 |
| Sum x | -5.39E-01 -5.39E-01 |
| Sum y | -2.10E-01 -2.10E-01 |
| Sum z | 1.09E-13 1.09E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.77E-04 5.75E-04 |
| Weighted Average x | -5.90E-03 -5.92E-03 |
| Weighted Average y | -3.33E-03 -3.32E-03 |
| Weighted Average z | 3.21E-14 3.25E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.71E-03 1.87E-03 |
| Sum x | -5.38E-01 -5.38E-01 |
| Sum y | -2.11E-01 -2.11E-01 |
| Sum z | 1.10E-13 1.10E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.74E-05 2.74E-05 |
| Weighted Average x | -5.90E-03 -5.92E-03 |
| Weighted Average y | -3.33E-03 -3.32E-03 |
| Weighted Average z | 3.21E-14 3.25E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 59 SIMULATION TIME = 5.90000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.46E-04 3.20E-04 |
| Sum x | -5.38E-01 -5.38E-01 |
| Sum y | -2.11E-01 -2.11E-01 |
| Sum z | 1.10E-13 1.10E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.29E-01 7.51E-01 |
| Weighted Average x | 7.93E-04 7.92E-04 |
| Weighted Average y | 5.07E-04 5.04E-04 |
| Weighted Average z | 1.33E-14 1.35E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.20E-01 2.53E-01 |
| Sum x | -7.11E-01 -7.11E-01 |
| Sum y | -3.42E-01 -3.42E-01 |
| Sum z | 1.12E-13 1.12E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.28E-03 9.80E-03 |
| Weighted Average x | 7.86E-04 7.85E-04 |
| Weighted Average y | 5.01E-04 4.98E-04 |
| Weighted Average z | 1.31E-14 1.32E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.32E-03 1.69E-03 |
| Sum x | -7.10E-01 -7.10E-01 |
| Sum y | -3.42E-01 -3.42E-01 |
| Sum z | 1.20E-13 1.20E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.34E-04 1.50E-04 |
| Weighted Average x | 7.86E-04 7.85E-04 |
| Weighted Average y | 5.01E-04 4.98E-04 |
| Weighted Average z | 1.31E-14 1.32E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 60 SIMULATION TIME = 6.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.34E-04 2.58E-04 |
| Sum x | -7.10E-01 -7.10E-01 |
| Sum y | -3.42E-01 -3.42E-01 |
| Sum z | 1.20E-13 1.20E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.50E-01 7.66E-01 |
| Weighted Average x | 7.50E-03 7.52E-03 |
| Weighted Average y | 4.21E-03 4.20E-03 |
| Weighted Average z | -1.12E-14 -1.14E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.86E-01 1.52E-01 |
| Sum x | -8.52E-01 -8.52E-01 |
| Sum y | -4.20E-01 -4.20E-01 |
| Sum z | 2.41E-13 2.41E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.92E-03 2.02E-03 |
| Weighted Average x | 7.50E-03 7.51E-03 |
| Weighted Average y | 4.20E-03 4.19E-03 |
| Weighted Average z | -1.08E-14 -1.10E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.39E-03 1.92E-03 |
| Sum x | -8.51E-01 -8.51E-01 |
| Sum y | -4.19E-01 -4.19E-01 |
| Sum z | 2.41E-13 2.41E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.04E-05 3.17E-05 |
| Weighted Average x | 7.50E-03 7.51E-03 |
| Weighted Average y | 4.20E-03 4.19E-03 |
| Weighted Average z | -1.09E-14 -1.10E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 61 SIMULATION TIME = 6.10000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.11E-04 1.68E-04 |
| Sum x | -8.51E-01 -8.51E-01 |
| Sum y | -4.19E-01 -4.19E-01 |
| Sum z | 2.42E-13 2.42E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.54E-01 7.70E-01 |
| Weighted Average x | 1.42E-02 1.42E-02 |
| Weighted Average y | 7.24E-03 7.22E-03 |
| Weighted Average z | -2.20E-14 -2.23E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.25E-01 9.78E-02 |
| Sum x | -9.69E-01 -9.69E-01 |
| Sum y | -4.45E-01 -4.45E-01 |
| Sum z | 5.21E-13 5.21E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.31E-03 1.36E-03 |
| Weighted Average x | 1.42E-02 1.42E-02 |
| Weighted Average y | 7.23E-03 7.21E-03 |
| Weighted Average z | -2.13E-14 -2.16E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.14E-03 2.47E-03 |
| Sum x | -9.67E-01 -9.67E-01 |
| Sum y | -4.44E-01 -4.44E-01 |
| Sum z | 5.33E-13 5.33E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.97E-05 1.97E-05 |
| Weighted Average x | 1.42E-02 1.42E-02 |
| Weighted Average y | 7.23E-03 7.21E-03 |
| Weighted Average z | -2.18E-14 -2.20E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 62 SIMULATION TIME = 6.20000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.94E-04 1.50E-04 |
| Sum x | -9.67E-01 -9.67E-01 |
| Sum y | -4.43E-01 -4.43E-01 |
| Sum z | 5.32E-13 5.32E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.57E-01 7.72E-01 |
| Weighted Average x | 2.07E-02 2.07E-02 |
| Weighted Average y | 9.19E-03 9.16E-03 |
| Weighted Average z | -1.37E-14 -1.39E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 9.58E-02 7.18E-02 |
| Sum x | -1.05E+00 -1.05E+00 |
| Sum y | -4.12E-01 -4.12E-01 |
| Sum z | 8.02E-13 8.02E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.09E-03 1.12E-03 |
| Weighted Average x | 2.06E-02 2.07E-02 |
| Weighted Average y | 9.17E-03 9.15E-03 |
| Weighted Average z | -1.34E-14 -1.35E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.22E-03 2.47E-03 |
| Sum x | -1.05E+00 -1.05E+00 |
| Sum y | -4.10E-01 -4.10E-01 |
| Sum z | 8.60E-13 8.60E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.51E-05 1.50E-05 |
| Weighted Average x | 2.06E-02 2.07E-02 |
| Weighted Average y | 9.17E-03 9.15E-03 |
| Weighted Average z | -1.34E-14 -1.36E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 63 SIMULATION TIME = 6.30000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.53E-04 1.14E-04 |
| Sum x | -1.05E+00 -1.05E+00 |
| Sum y | -4.10E-01 -4.10E-01 |
| Sum z | 8.64E-13 8.64E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.58E-01 7.73E-01 |
| Weighted Average x | 2.66E-02 2.66E-02 |
| Weighted Average y | 9.79E-03 9.75E-03 |
| Weighted Average z | 7.80E-16 9.30E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 9.95E-02 7.39E-02 |
| Sum x | -1.09E+00 -1.09E+00 |
| Sum y | -3.30E-01 -3.30E-01 |
| Sum z | 6.95E-13 6.95E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.06E-03 1.09E-03 |
| Weighted Average x | 2.66E-02 2.66E-02 |
| Weighted Average y | 9.77E-03 9.74E-03 |
| Weighted Average z | 4.40E-16 5.62E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.96E-03 2.99E-03 |
| Sum x | -1.08E+00 -1.08E+00 |
| Sum y | -3.28E-01 -3.28E-01 |
| Sum z | 7.57E-13 7.57E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.23E-05 1.23E-05 |
| Weighted Average x | 2.66E-02 2.66E-02 |
| Weighted Average y | 9.77E-03 9.74E-03 |
| Weighted Average z | 4.59E-16 5.81E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 64 SIMULATION TIME = 6.40000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.23E-04 9.06E-05 |
| Sum x | -1.08E+00 -1.08E+00 |
| Sum y | -3.28E-01 -3.28E-01 |
| Sum z | 7.60E-13 7.60E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.58E-01 7.73E-01 |
| Weighted Average x | 3.17E-02 3.17E-02 |
| Weighted Average y | 8.95E-03 8.91E-03 |
| Weighted Average z | 5.29E-15 5.59E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.15E-01 8.61E-02 |
| Sum x | -1.08E+00 -1.08E+00 |
| Sum y | -2.13E-01 -2.13E-01 |
| Sum z | 7.37E-13 7.37E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.06E-03 1.09E-03 |
| Weighted Average x | 3.16E-02 3.17E-02 |
| Weighted Average y | 8.93E-03 8.90E-03 |
| Weighted Average z | 5.05E-15 5.32E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.58E-03 3.46E-03 |
| Sum x | -1.08E+00 -1.08E+00 |
| Sum y | -2.12E-01 -2.12E-01 |
| Sum z | 7.21E-13 7.21E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.08E-05 1.09E-05 |
| Weighted Average x | 3.16E-02 3.17E-02 |
| Weighted Average y | 8.93E-03 8.90E-03 |
| Weighted Average z | 5.02E-15 5.30E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 65 SIMULATION TIME = 6.50000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.35E-04 9.95E-05 |
| Sum x | -1.08E+00 -1.08E+00 |
| Sum y | -2.12E-01 -2.12E-01 |
| Sum z | 7.23E-13 7.23E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.58E-01 7.73E-01 |
| Weighted Average x | 3.55E-02 3.56E-02 |
| Weighted Average y | 6.78E-03 6.75E-03 |
| Weighted Average z | 2.89E-15 2.94E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.26E-01 9.65E-02 |
| Sum x | -1.04E+00 -1.04E+00 |
| Sum y | -8.16E-02 -8.16E-02 |
| Sum z | 4.14E-13 4.14E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.07E-03 1.10E-03 |
| Weighted Average x | 3.55E-02 3.55E-02 |
| Weighted Average y | 6.77E-03 6.74E-03 |
| Weighted Average z | 2.83E-15 2.83E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.96E-03 3.79E-03 |
| Sum x | -1.03E+00 -1.03E+00 |
| Sum y | -8.05E-02 -8.05E-02 |
| Sum z | 3.61E-13 3.61E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.29E-06 9.59E-06 |
| Weighted Average x | 3.55E-02 3.55E-02 |
| Weighted Average y | 6.77E-03 6.74E-03 |
| Weighted Average z | 2.85E-15 2.86E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 66 SIMULATION TIME = 6.60000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.89E-04 1.41E-04 |
| Sum x | -1.03E+00 -1.03E+00 |
| Sum y | -8.05E-02 -8.05E-02 |
| Sum z | 3.62E-13 3.62E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.59E-01 7.74E-01 |
| Weighted Average x | 3.79E-02 3.79E-02 |
| Weighted Average y | 3.63E-03 3.61E-03 |
| Weighted Average z | -5.57E-16 -7.19E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.35E-01 1.04E-01 |
| Sum x | -9.54E-01 -9.54E-01 |
| Sum y | 4.76E-02 4.76E-02 |
| Sum z | 1.04E-13 1.04E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.07E-03 1.10E-03 |
| Weighted Average x | 3.79E-02 3.79E-02 |
| Weighted Average y | 3.62E-03 3.61E-03 |
| Weighted Average z | -4.55E-16 -5.43E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.52E-03 4.24E-03 |
| Sum x | -9.48E-01 -9.48E-01 |
| Sum y | 4.79E-02 4.79E-02 |
| Sum z | 1.62E-13 1.62E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 7.34E-06 7.66E-06 |
| Weighted Average x | 3.79E-02 3.79E-02 |
| Weighted Average y | 3.63E-03 3.61E-03 |
| Weighted Average z | -4.61E-16 -5.61E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 67 SIMULATION TIME = 6.70000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.81E-04 1.36E-04 |
| Sum x | -9.48E-01 -9.48E-01 |
| Sum y | 4.79E-02 4.79E-02 |
| Sum z | 1.60E-13 1.60E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.75E-01 |
| Weighted Average x | 3.86E-02 3.86E-02 |
| Weighted Average y | 5.23E-06 5.48E-06 |
| Weighted Average z | -1.94E-15 -1.95E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.48E-01 1.14E-01 |
| Sum x | -8.29E-01 -8.29E-01 |
| Sum y | 1.57E-01 1.57E-01 |
| Sum z | 4.34E-14 4.34E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.03E-03 1.06E-03 |
| Weighted Average x | 3.85E-02 3.85E-02 |
| Weighted Average y | 8.28E-06 8.51E-06 |
| Weighted Average z | -1.87E-15 -1.88E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.11E-03 4.67E-03 |
| Sum x | -8.23E-01 -8.23E-01 |
| Sum y | 1.56E-01 1.56E-01 |
| Sum z | 6.25E-14 6.25E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.06E-06 6.12E-06 |
| Weighted Average x | 3.85E-02 3.85E-02 |
| Weighted Average y | 8.21E-06 8.45E-06 |
| Weighted Average z | -1.88E-15 -1.90E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 68 SIMULATION TIME = 6.80000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.89E-04 1.42E-04 |
| Sum x | -8.22E-01 -8.22E-01 |
| Sum y | 1.56E-01 1.56E-01 |
| Sum z | 6.31E-14 6.31E-14 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.75E-01 |
| Weighted Average x | 3.74E-02 3.74E-02 |
| Weighted Average y | -3.52E-03 -3.51E-03 |
| Weighted Average z | -1.26E-15 -1.23E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.76E-01 1.33E-01 |
| Sum x | -6.63E-01 -6.63E-01 |
| Sum y | 2.31E-01 2.31E-01 |
| Sum z | -2.83E-13 -2.83E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.00E-03 1.02E-03 |
| Weighted Average x | 3.73E-02 3.74E-02 |
| Weighted Average y | -3.51E-03 -3.50E-03 |
| Weighted Average z | -1.31E-15 -1.20E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.03E-03 5.26E-03 |
| Sum x | -6.57E-01 -6.57E-01 |
| Sum y | 2.29E-01 2.29E-01 |
| Sum z | -2.77E-13 -2.77E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 7.08E-06 7.01E-06 |
| Weighted Average x | 3.73E-02 3.74E-02 |
| Weighted Average y | -3.51E-03 -3.50E-03 |
| Weighted Average z | -1.30E-15 -1.23E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 69 SIMULATION TIME = 6.90000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.86E-04 1.37E-04 |
| Sum x | -6.57E-01 -6.57E-01 |
| Sum y | 2.29E-01 2.29E-01 |
| Sum z | -2.78E-13 -2.78E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.59E-01 7.74E-01 |
| Weighted Average x | 3.45E-02 3.45E-02 |
| Weighted Average y | -6.43E-03 -6.40E-03 |
| Weighted Average z | -1.72E-15 -1.75E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.32E-01 1.66E-01 |
| Sum x | -4.67E-01 -4.67E-01 |
| Sum y | 2.58E-01 2.58E-01 |
| Sum z | -5.73E-13 -5.73E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.71E-04 9.93E-04 |
| Weighted Average x | 3.44E-02 3.45E-02 |
| Weighted Average y | -6.42E-03 -6.39E-03 |
| Weighted Average z | -1.74E-15 -1.75E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 8.71E-03 6.18E-03 |
| Sum x | -4.61E-01 -4.61E-01 |
| Sum y | 2.56E-01 2.56E-01 |
| Sum z | -5.63E-13 -5.63E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.16E-06 9.13E-06 |
| Weighted Average x | 3.44E-02 3.45E-02 |
| Weighted Average y | -6.42E-03 -6.39E-03 |
| Weighted Average z | -1.79E-15 -1.78E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 70 SIMULATION TIME = 7.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.89E-04 2.01E-04 |
| Sum x | -4.61E-01 -4.61E-01 |
| Sum y | 2.56E-01 2.56E-01 |
| Sum z | -5.65E-13 -5.65E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.58E-01 7.73E-01 |
| Weighted Average x | 3.01E-02 3.02E-02 |
| Weighted Average y | -8.29E-03 -8.26E-03 |
| Weighted Average z | -1.33E-15 -1.43E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.60E-01 2.28E-01 |
| Sum x | -2.60E-01 -2.60E-01 |
| Sum y | 2.37E-01 2.37E-01 |
| Sum z | -8.95E-13 -8.95E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.45E-04 9.75E-04 |
| Weighted Average x | 3.01E-02 3.01E-02 |
| Weighted Average y | -8.28E-03 -8.24E-03 |
| Weighted Average z | -1.21E-15 -1.29E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.22E-02 7.62E-03 |
| Sum x | -2.55E-01 -2.55E-01 |
| Sum y | 2.35E-01 2.35E-01 |
| Sum z | -8.98E-13 -8.98E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.24E-05 1.25E-05 |
| Weighted Average x | 3.01E-02 3.01E-02 |
| Weighted Average y | -8.28E-03 -8.24E-03 |
| Weighted Average z | -1.31E-15 -1.38E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 71 SIMULATION TIME = 7.10000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.70E-04 2.92E-04 |
| Sum x | -2.55E-01 -2.55E-01 |
| Sum y | 2.35E-01 2.35E-01 |
| Sum z | -9.01E-13 -9.01E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.56E-01 7.71E-01 |
| Weighted Average x | 2.48E-02 2.48E-02 |
| Weighted Average y | -8.85E-03 -8.82E-03 |
| Weighted Average z | 2.43E-15 2.31E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 6.54E-01 3.60E-01 |
| Sum x | -5.38E-02 -5.38E-02 |
| Sum y | 1.71E-01 1.71E-01 |
| Sum z | -1.19E-12 -1.19E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.12E-04 9.49E-04 |
| Weighted Average x | 2.48E-02 2.48E-02 |
| Weighted Average y | -8.83E-03 -8.80E-03 |
| Weighted Average z | 2.48E-15 2.39E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.65E-02 9.05E-03 |
| Sum x | -4.98E-02 -4.98E-02 |
| Sum y | 1.68E-01 1.68E-01 |
| Sum z | -1.19E-12 -1.19E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.62E-05 1.62E-05 |
| Weighted Average x | 2.48E-02 2.48E-02 |
| Weighted Average y | -8.83E-03 -8.80E-03 |
| Weighted Average z | 2.54E-15 2.43E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 72 SIMULATION TIME = 7.20000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 8.14E-04 4.40E-04 |
| Sum x | -4.97E-02 -4.97E-02 |
| Sum y | 1.68E-01 1.68E-01 |
| Sum z | -1.20E-12 -1.20E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.55E-01 7.70E-01 |
| Weighted Average x | 1.88E-02 1.88E-02 |
| Weighted Average y | -8.03E-03 -8.01E-03 |
| Weighted Average z | 6.93E-15 6.96E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.32E+00 6.44E-01 |
| Sum x | 1.45E-01 1.45E-01 |
| Sum y | 6.93E-02 6.93E-02 |
| Sum z | -1.46E-12 -1.46E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.24E-04 8.61E-04 |
| Weighted Average x | 1.88E-02 1.88E-02 |
| Weighted Average y | -8.02E-03 -7.99E-03 |
| Weighted Average z | 6.85E-15 6.88E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.40E-02 1.63E-02 |
| Sum x | 1.49E-01 1.49E-01 |
| Sum y | 6.67E-02 6.67E-02 |
| Sum z | -1.44E-12 -1.44E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.88E-05 1.88E-05 |
| Weighted Average x | 1.88E-02 1.88E-02 |
| Weighted Average y | -8.02E-03 -8.00E-03 |
| Weighted Average z | 6.79E-15 6.81E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.79E-03 8.55E-04 |
| Sum x | 1.49E-01 1.49E-01 |
| Sum y | 6.66E-02 6.66E-02 |
| Sum z | -1.44E-12 -1.44E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.36E-06 1.36E-06 |
| Weighted Average x | 1.88E-02 1.88E-02 |
| Weighted Average y | -8.02E-03 -8.00E-03 |
| Weighted Average z | 6.86E-15 6.89E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 73 SIMULATION TIME = 7.30000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.09E-04 5.21E-05 |
| Sum x | 1.49E-01 1.49E-01 |
| Sum y | 6.66E-02 6.66E-02 |
| Sum z | -1.44E-12 -1.44E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.55E-01 7.70E-01 |
| Weighted Average x | 1.25E-02 1.25E-02 |
| Weighted Average y | -6.01E-03 -5.99E-03 |
| Weighted Average z | 6.09E-15 6.14E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 6.69E-01 4.83E-01 |
| Sum x | 3.33E-01 3.33E-01 |
| Sum y | -5.17E-02 -5.17E-02 |
| Sum z | -1.46E-12 -1.46E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.33E-04 6.58E-04 |
| Weighted Average x | 1.25E-02 1.25E-02 |
| Weighted Average y | -6.00E-03 -5.98E-03 |
| Weighted Average z | 6.17E-15 6.22E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.17E-02 8.22E-03 |
| Sum x | 3.36E-01 3.36E-01 |
| Sum y | -5.41E-02 -5.41E-02 |
| Sum z | -1.46E-12 -1.46E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.93E-05 1.94E-05 |
| Weighted Average x | 1.25E-02 1.25E-02 |
| Weighted Average y | -6.00E-03 -5.98E-03 |
| Weighted Average z | 5.99E-15 6.04E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 74 SIMULATION TIME = 7.40000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.03E-04 2.60E-04 |
| Sum x | 3.36E-01 3.36E-01 |
| Sum y | -5.41E-02 -5.41E-02 |
| Sum z | -1.46E-12 -1.46E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.56E-01 7.71E-01 |
| Weighted Average x | 6.02E-03 6.04E-03 |
| Weighted Average y | -3.10E-03 -3.09E-03 |
| Weighted Average z | -1.63E-15 -1.56E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.93E-01 3.10E-01 |
| Sum x | 5.06E-01 5.06E-01 |
| Sum y | -1.76E-01 -1.76E-01 |
| Sum z | -1.04E-12 -1.04E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.33E-04 3.31E-04 |
| Weighted Average x | 6.03E-03 6.04E-03 |
| Weighted Average y | -3.09E-03 -3.09E-03 |
| Weighted Average z | -1.58E-15 -1.52E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.36E-03 3.33E-03 |
| Sum x | 5.08E-01 5.08E-01 |
| Sum y | -1.77E-01 -1.77E-01 |
| Sum z | -1.01E-12 -1.01E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.10E-05 2.14E-05 |
| Weighted Average x | 6.03E-03 6.04E-03 |
| Weighted Average y | -3.09E-03 -3.09E-03 |
| Weighted Average z | -1.60E-15 -1.54E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 75 SIMULATION TIME = 7.50000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.22E-04 8.85E-05 |
| Sum x | 5.08E-01 5.08E-01 |
| Sum y | -1.77E-01 -1.77E-01 |
| Sum z | -1.01E-12 -1.01E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.46E-01 7.64E-01 |
| Weighted Average x | -4.57E-04 -4.58E-04 |
| Weighted Average y | 2.45E-04 2.44E-04 |
| Weighted Average z | -2.80E-15 -2.76E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.61E-01 2.10E-01 |
| Sum x | 6.72E-01 6.72E-01 |
| Sum y | -2.91E-01 -2.91E-01 |
| Sum z | -7.10E-13 -7.10E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.43E-02 1.48E-02 |
| Weighted Average x | -4.49E-04 -4.50E-04 |
| Weighted Average y | 2.40E-04 2.39E-04 |
| Weighted Average z | -2.86E-15 -2.82E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.52E-03 1.07E-03 |
| Sum x | 6.73E-01 6.73E-01 |
| Sum y | -2.92E-01 -2.92E-01 |
| Sum z | -7.16E-13 -7.16E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.73E-05 1.12E-04 |
| Weighted Average x | -4.49E-04 -4.50E-04 |
| Weighted Average y | 2.40E-04 2.39E-04 |
| Weighted Average z | -2.85E-15 -2.82E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 76 SIMULATION TIME = 7.60000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.01E-04 1.53E-04 |
| Sum x | 6.73E-01 6.73E-01 |
| Sum y | -2.92E-01 -2.92E-01 |
| Sum z | -7.17E-13 -7.17E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.55E-01 7.71E-01 |
| Weighted Average x | -6.90E-03 -6.91E-03 |
| Weighted Average y | 3.52E-03 3.51E-03 |
| Weighted Average z | 1.67E-15 1.73E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.51E-01 1.25E-01 |
| Sum x | 8.02E-01 8.02E-01 |
| Sum y | -3.58E-01 -3.58E-01 |
| Sum z | -4.96E-13 -4.96E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.66E-03 1.72E-03 |
| Weighted Average x | -6.88E-03 -6.90E-03 |
| Weighted Average y | 3.51E-03 3.50E-03 |
| Weighted Average z | 1.46E-15 1.52E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.00E-03 2.39E-03 |
| Sum x | 8.00E-01 8.00E-01 |
| Sum y | -3.57E-01 -3.57E-01 |
| Sum z | -5.15E-13 -5.15E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.90E-05 1.94E-05 |
| Weighted Average x | -6.88E-03 -6.90E-03 |
| Weighted Average y | 3.51E-03 3.50E-03 |
| Weighted Average z | 1.59E-15 1.65E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 77 SIMULATION TIME = 7.70000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.71E-04 1.35E-04 |
| Sum x | 8.00E-01 8.00E-01 |
| Sum y | -3.57E-01 -3.57E-01 |
| Sum z | -5.14E-13 -5.14E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.56E-01 7.71E-01 |
| Weighted Average x | -1.32E-02 -1.32E-02 |
| Weighted Average y | 6.25E-03 6.24E-03 |
| Weighted Average z | -8.37E-15 -8.46E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 9.93E-02 8.34E-02 |
| Sum x | 9.02E-01 9.02E-01 |
| Sum y | -3.79E-01 -3.79E-01 |
| Sum z | -2.94E-13 -2.94E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.25E-03 1.29E-03 |
| Weighted Average x | -1.32E-02 -1.32E-02 |
| Weighted Average y | 6.24E-03 6.22E-03 |
| Weighted Average z | -8.48E-15 -8.54E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.41E-03 3.61E-03 |
| Sum x | 9.00E-01 9.00E-01 |
| Sum y | -3.78E-01 -3.78E-01 |
| Sum z | -2.76E-13 -2.76E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.71E-05 1.71E-05 |
| Weighted Average x | -1.32E-02 -1.32E-02 |
| Weighted Average y | 6.24E-03 6.22E-03 |
| Weighted Average z | -8.39E-15 -8.46E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 78 SIMULATION TIME = 7.80000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.16E-04 1.77E-04 |
| Sum x | 9.00E-01 9.00E-01 |
| Sum y | -3.78E-01 -3.78E-01 |
| Sum z | -2.76E-13 -2.76E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.56E-01 7.71E-01 |
| Weighted Average x | -1.92E-02 -1.93E-02 |
| Weighted Average y | 8.05E-03 8.03E-03 |
| Weighted Average z | -2.38E-14 -2.39E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 7.43E-02 6.29E-02 |
| Sum x | 9.71E-01 9.71E-01 |
| Sum y | -3.51E-01 -3.51E-01 |
| Sum z | -1.63E-13 -1.63E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.10E-03 1.14E-03 |
| Weighted Average x | -1.92E-02 -1.92E-02 |
| Weighted Average y | 8.04E-03 8.01E-03 |
| Weighted Average z | -2.33E-14 -2.34E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.38E-03 3.70E-03 |
| Sum x | 9.68E-01 9.68E-01 |
| Sum y | -3.49E-01 -3.49E-01 |
| Sum z | -1.16E-13 -1.16E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.54E-05 1.55E-05 |
| Weighted Average x | -1.92E-02 -1.92E-02 |
| Weighted Average y | 8.04E-03 8.01E-03 |
| Weighted Average z | -2.33E-14 -2.34E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 79 SIMULATION TIME = 7.90000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.84E-04 1.53E-04 |
| Sum x | 9.67E-01 9.67E-01 |
| Sum y | -3.49E-01 -3.49E-01 |
| Sum z | -1.15E-13 -1.15E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.56E-01 7.72E-01 |
| Weighted Average x | -2.48E-02 -2.48E-02 |
| Weighted Average y | 8.65E-03 8.62E-03 |
| Weighted Average z | -1.50E-14 -1.52E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 7.71E-02 6.57E-02 |
| Sum x | 1.01E+00 1.01E+00 |
| Sum y | -2.81E-01 -2.81E-01 |
| Sum z | 1.47E-13 1.47E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.08E-03 1.11E-03 |
| Weighted Average x | -2.47E-02 -2.48E-02 |
| Weighted Average y | 8.64E-03 8.61E-03 |
| Weighted Average z | -1.45E-14 -1.46E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.97E-03 4.24E-03 |
| Sum x | 1.00E+00 1.00E+00 |
| Sum y | -2.79E-01 -2.79E-01 |
| Sum z | 1.28E-13 1.28E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.33E-05 1.34E-05 |
| Weighted Average x | -2.47E-02 -2.48E-02 |
| Weighted Average y | 8.64E-03 8.61E-03 |
| Weighted Average z | -1.45E-14 -1.47E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 80 SIMULATION TIME = 8.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.34E-04 1.13E-04 |
| Sum x | 1.00E+00 1.00E+00 |
| Sum y | -2.79E-01 -2.79E-01 |
| Sum z | 1.29E-13 1.29E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.57E-01 7.72E-01 |
| Weighted Average x | -2.96E-02 -2.96E-02 |
| Weighted Average y | 7.97E-03 7.93E-03 |
| Weighted Average z | 1.33E-14 1.32E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 9.33E-02 7.72E-02 |
| Sum x | 1.01E+00 1.01E+00 |
| Sum y | -1.81E-01 -1.81E-01 |
| Sum z | 3.76E-13 3.76E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.05E-03 1.08E-03 |
| Weighted Average x | -2.95E-02 -2.95E-02 |
| Weighted Average y | 7.95E-03 7.92E-03 |
| Weighted Average z | 1.32E-14 1.31E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.17E-03 4.26E-03 |
| Sum x | 1.00E+00 1.00E+00 |
| Sum y | -1.80E-01 -1.80E-01 |
| Sum z | 3.61E-13 3.61E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.07E-05 1.08E-05 |
| Weighted Average x | -2.95E-02 -2.95E-02 |
| Weighted Average y | 7.95E-03 7.92E-03 |
| Weighted Average z | 1.32E-14 1.31E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 81 SIMULATION TIME = 8.10000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.45E-04 1.18E-04 |
| Sum x | 1.00E+00 1.00E+00 |
| Sum y | -1.80E-01 -1.80E-01 |
| Sum z | 3.62E-13 3.62E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.59E-01 7.73E-01 |
| Weighted Average x | -3.32E-02 -3.33E-02 |
| Weighted Average y | 6.11E-03 6.08E-03 |
| Weighted Average z | 3.14E-14 3.17E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.13E-01 9.06E-02 |
| Sum x | 9.70E-01 9.70E-01 |
| Sum y | -6.71E-02 -6.71E-02 |
| Sum z | 5.56E-13 5.56E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.03E-03 1.06E-03 |
| Weighted Average x | -3.32E-02 -3.32E-02 |
| Weighted Average y | 6.10E-03 6.07E-03 |
| Weighted Average z | 3.07E-14 3.11E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.56E-03 4.41E-03 |
| Sum x | 9.65E-01 9.65E-01 |
| Sum y | -6.64E-02 -6.64E-02 |
| Sum z | 5.52E-13 5.52E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.04E-06 8.14E-06 |
| Weighted Average x | -3.32E-02 -3.32E-02 |
| Weighted Average y | 6.10E-03 6.07E-03 |
| Weighted Average z | 3.07E-14 3.11E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 82 SIMULATION TIME = 8.20000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.46E-04 1.14E-04 |
| Sum x | 9.65E-01 9.65E-01 |
| Sum y | -6.64E-02 -6.64E-02 |
| Sum z | 5.53E-13 5.53E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.74E-01 |
| Weighted Average x | -3.55E-02 -3.55E-02 |
| Weighted Average y | 3.38E-03 3.36E-03 |
| Weighted Average z | 2.38E-14 2.42E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.37E-01 1.05E-01 |
| Sum x | 8.89E-01 8.89E-01 |
| Sum y | 4.56E-02 4.56E-02 |
| Sum z | 6.53E-13 6.53E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.02E-03 1.04E-03 |
| Weighted Average x | -3.55E-02 -3.55E-02 |
| Weighted Average y | 3.37E-03 3.36E-03 |
| Weighted Average z | 2.31E-14 2.35E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.17E-03 4.67E-03 |
| Sum x | 8.83E-01 8.83E-01 |
| Sum y | 4.58E-02 4.58E-02 |
| Sum z | 6.62E-13 6.62E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.28E-06 6.34E-06 |
| Weighted Average x | -3.55E-02 -3.55E-02 |
| Weighted Average y | 3.37E-03 3.36E-03 |
| Weighted Average z | 2.32E-14 2.35E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 83 SIMULATION TIME = 8.30000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.78E-04 1.33E-04 |
| Sum x | 8.83E-01 8.83E-01 |
| Sum y | 4.58E-02 4.58E-02 |
| Sum z | 6.63E-13 6.63E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.75E-01 |
| Weighted Average x | -3.62E-02 -3.62E-02 |
| Weighted Average y | 2.05E-04 2.04E-04 |
| Weighted Average z | -4.03E-15 -4.09E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.66E-01 1.20E-01 |
| Sum x | 7.66E-01 7.66E-01 |
| Sum y | 1.41E-01 1.41E-01 |
| Sum z | 5.95E-13 5.95E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.69E-04 9.83E-04 |
| Weighted Average x | -3.61E-02 -3.61E-02 |
| Weighted Average y | 2.07E-04 2.06E-04 |
| Weighted Average z | -4.35E-15 -4.39E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 7.54E-03 5.36E-03 |
| Sum x | 7.60E-01 7.60E-01 |
| Sum y | 1.40E-01 1.40E-01 |
| Sum z | 5.94E-13 5.94E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.97E-06 6.03E-06 |
| Weighted Average x | -3.61E-02 -3.61E-02 |
| Weighted Average y | 2.07E-04 2.06E-04 |
| Weighted Average z | -4.31E-15 -4.35E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 84 SIMULATION TIME = 8.40000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.65E-04 1.86E-04 |
| Sum x | 7.60E-01 7.60E-01 |
| Sum y | 1.40E-01 1.40E-01 |
| Sum z | 5.91E-13 5.91E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.74E-01 |
| Weighted Average x | -3.52E-02 -3.52E-02 |
| Weighted Average y | -2.93E-03 -2.91E-03 |
| Weighted Average z | -2.76E-14 -2.80E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.07E-01 1.39E-01 |
| Sum x | 6.11E-01 6.11E-01 |
| Sum y | 2.04E-01 2.04E-01 |
| Sum z | 4.09E-13 4.09E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.85E-04 1.01E-03 |
| Weighted Average x | -3.51E-02 -3.51E-02 |
| Weighted Average y | -2.92E-03 -2.91E-03 |
| Weighted Average z | -2.68E-14 -2.72E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 8.95E-03 5.91E-03 |
| Sum x | 6.06E-01 6.06E-01 |
| Sum y | 2.03E-01 2.03E-01 |
| Sum z | 2.34E-13 2.34E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.84E-06 6.89E-06 |
| Weighted Average x | -3.51E-02 -3.51E-02 |
| Weighted Average y | -2.92E-03 -2.91E-03 |
| Weighted Average z | -2.69E-14 -2.73E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 85 SIMULATION TIME = 8.50000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.51E-04 2.29E-04 |
| Sum x | 6.05E-01 6.05E-01 |
| Sum y | 2.03E-01 2.03E-01 |
| Sum z | 2.31E-13 2.31E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.59E-01 7.73E-01 |
| Weighted Average x | -3.26E-02 -3.26E-02 |
| Weighted Average y | -5.54E-03 -5.52E-03 |
| Weighted Average z | -2.16E-14 -2.21E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.56E-01 1.66E-01 |
| Sum x | 4.35E-01 4.35E-01 |
| Sum y | 2.29E-01 2.29E-01 |
| Sum z | -2.60E-13 -2.60E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.61E-04 9.87E-04 |
| Weighted Average x | -3.25E-02 -3.26E-02 |
| Weighted Average y | -5.53E-03 -5.50E-03 |
| Weighted Average z | -2.10E-14 -2.13E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.03E-02 6.61E-03 |
| Sum x | 4.30E-01 4.30E-01 |
| Sum y | 2.27E-01 2.27E-01 |
| Sum z | -2.33E-13 -2.33E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.05E-06 9.12E-06 |
| Weighted Average x | -3.25E-02 -3.26E-02 |
| Weighted Average y | -5.53E-03 -5.50E-03 |
| Weighted Average z | -2.10E-14 -2.14E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 86 SIMULATION TIME = 8.60000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.03E-04 2.56E-04 |
| Sum x | 4.29E-01 4.29E-01 |
| Sum y | 2.27E-01 2.27E-01 |
| Sum z | -2.33E-13 -2.33E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.58E-01 7.73E-01 |
| Weighted Average x | -2.87E-02 -2.87E-02 |
| Weighted Average y | -7.24E-03 -7.22E-03 |
| Weighted Average z | 4.93E-15 4.92E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.55E-01 2.18E-01 |
| Sum x | 2.48E-01 2.48E-01 |
| Sum y | 2.11E-01 2.11E-01 |
| Sum z | -6.93E-13 -6.93E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.21E-04 9.49E-04 |
| Weighted Average x | -2.87E-02 -2.87E-02 |
| Weighted Average y | -7.23E-03 -7.20E-03 |
| Weighted Average z | 4.79E-15 4.77E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.24E-02 7.48E-03 |
| Sum x | 2.44E-01 2.44E-01 |
| Sum y | 2.08E-01 2.08E-01 |
| Sum z | -6.48E-13 -6.48E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.16E-05 1.17E-05 |
| Weighted Average x | -2.87E-02 -2.87E-02 |
| Weighted Average y | -7.23E-03 -7.20E-03 |
| Weighted Average z | 4.74E-15 4.74E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 87 SIMULATION TIME = 8.70000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.52E-04 3.30E-04 |
| Sum x | 2.43E-01 2.43E-01 |
| Sum y | 2.08E-01 2.08E-01 |
| Sum z | -6.48E-13 -6.48E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.57E-01 7.72E-01 |
| Weighted Average x | -2.38E-02 -2.38E-02 |
| Weighted Average y | -7.80E-03 -7.78E-03 |
| Weighted Average z | 2.47E-14 2.51E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 6.29E-01 3.50E-01 |
| Sum x | 5.65E-02 5.65E-02 |
| Sum y | 1.52E-01 1.52E-01 |
| Sum z | -7.52E-13 -7.52E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.52E-04 8.81E-04 |
| Weighted Average x | -2.38E-02 -2.38E-02 |
| Weighted Average y | -7.79E-03 -7.76E-03 |
| Weighted Average z | 2.41E-14 2.45E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.69E-02 9.28E-03 |
| Sum x | 5.26E-02 5.26E-02 |
| Sum y | 1.50E-01 1.50E-01 |
| Sum z | -7.14E-13 -7.14E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.40E-05 1.40E-05 |
| Weighted Average x | -2.38E-02 -2.38E-02 |
| Weighted Average y | -7.79E-03 -7.76E-03 |
| Weighted Average z | 2.41E-14 2.45E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 88 SIMULATION TIME = 8.80000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.14E-04 4.96E-04 |
| Sum x | 5.26E-02 5.26E-02 |
| Sum y | 1.50E-01 1.50E-01 |
| Sum z | -7.13E-13 -7.13E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.56E-01 7.71E-01 |
| Weighted Average x | -1.82E-02 -1.82E-02 |
| Weighted Average y | -7.16E-03 -7.14E-03 |
| Weighted Average z | 2.12E-14 2.16E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 9.51E-01 6.49E-01 |
| Sum x | -1.33E-01 -1.33E-01 |
| Sum y | 6.07E-02 6.07E-02 |
| Sum z | -6.35E-13 -6.35E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 7.52E-04 7.79E-04 |
| Weighted Average x | -1.82E-02 -1.82E-02 |
| Weighted Average y | -7.15E-03 -7.12E-03 |
| Weighted Average z | 2.06E-14 2.09E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.45E-02 1.68E-02 |
| Sum x | -1.37E-01 -1.37E-01 |
| Sum y | 5.85E-02 5.85E-02 |
| Sum z | -6.04E-13 -6.04E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.61E-05 1.60E-05 |
| Weighted Average x | -1.82E-02 -1.82E-02 |
| Weighted Average y | -7.15E-03 -7.12E-03 |
| Weighted Average z | 2.06E-14 2.10E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.93E-03 1.33E-03 |
| Sum x | -1.37E-01 -1.37E-01 |
| Sum y | 5.84E-02 5.84E-02 |
| Sum z | -6.02E-13 -6.02E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.27E-06 1.27E-06 |
| Weighted Average x | -1.82E-02 -1.82E-02 |
| Weighted Average y | -7.15E-03 -7.12E-03 |
| Weighted Average z | 2.06E-14 2.10E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 89 SIMULATION TIME = 8.90000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.59E-05 6.58E-05 |
| Sum x | -1.37E-01 -1.37E-01 |
| Sum y | 5.84E-02 5.84E-02 |
| Sum z | -6.02E-13 -6.02E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.55E-01 7.71E-01 |
| Weighted Average x | -1.22E-02 -1.23E-02 |
| Weighted Average y | -5.44E-03 -5.42E-03 |
| Weighted Average z | 2.00E-15 2.01E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 5.94E-01 4.54E-01 |
| Sum x | -3.13E-01 -3.13E-01 |
| Sum y | -4.76E-02 -4.76E-02 |
| Sum z | -2.07E-13 -2.07E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.11E-04 6.35E-04 |
| Weighted Average x | -1.22E-02 -1.23E-02 |
| Weighted Average y | -5.43E-03 -5.42E-03 |
| Weighted Average z | 2.06E-15 2.06E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.03E-02 7.49E-03 |
| Sum x | -3.16E-01 -3.16E-01 |
| Sum y | -4.94E-02 -4.94E-02 |
| Sum z | -1.77E-13 -1.77E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.73E-05 1.74E-05 |
| Weighted Average x | -1.22E-02 -1.23E-02 |
| Weighted Average y | -5.43E-03 -5.42E-03 |
| Weighted Average z | 2.01E-15 2.01E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 90 SIMULATION TIME = 9.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.17E-03 8.62E-04 |
| Sum x | -3.16E-01 -3.16E-01 |
| Sum y | -4.95E-02 -4.95E-02 |
| Sum z | -1.76E-13 -1.76E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.54E-01 7.70E-01 |
| Weighted Average x | -6.10E-03 -6.12E-03 |
| Weighted Average y | -2.92E-03 -2.91E-03 |
| Weighted Average z | -1.05E-14 -1.10E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 4.54E-01 3.35E-01 |
| Sum x | -4.77E-01 -4.77E-01 |
| Sum y | -1.58E-01 -1.58E-01 |
| Sum z | 3.20E-13 3.20E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 4.24E-04 4.28E-04 |
| Weighted Average x | -6.11E-03 -6.12E-03 |
| Weighted Average y | -2.92E-03 -2.91E-03 |
| Weighted Average z | -1.00E-14 -1.05E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.32E-03 1.60E-03 |
| Sum x | -4.77E-01 -4.77E-01 |
| Sum y | -1.58E-01 -1.58E-01 |
| Sum z | 3.44E-13 3.44E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.12E-05 2.12E-05 |
| Weighted Average x | -6.11E-03 -6.12E-03 |
| Weighted Average y | -2.92E-03 -2.91E-03 |
| Weighted Average z | -1.01E-14 -1.05E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 91 SIMULATION TIME = 9.10000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.38E-04 3.16E-04 |
| Sum x | -4.77E-01 -4.77E-01 |
| Sum y | -1.58E-01 -1.58E-01 |
| Sum z | 3.44E-13 3.44E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.42E-01 8.48E-01 |
| Weighted Average x | 6.93E-05 7.04E-05 |
| Weighted Average y | 1.37E-05 1.41E-05 |
| Weighted Average z | -1.01E-14 -1.03E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.23E-01 2.51E-01 |
| Sum x | -6.30E-01 -6.30E-01 |
| Sum y | -2.59E-01 -2.59E-01 |
| Sum z | 7.54E-13 7.54E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.31E-01 1.36E-01 |
| Weighted Average x | 6.13E-05 6.23E-05 |
| Weighted Average y | 1.03E-05 1.07E-05 |
| Weighted Average z | -9.88E-15 -1.01E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.24E-03 2.31E-03 |
| Sum x | -6.29E-01 -6.29E-01 |
| Sum y | -2.59E-01 -2.59E-01 |
| Sum z | 8.00E-13 8.00E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.11E-03 1.20E-03 |
| Weighted Average x | 6.13E-05 6.23E-05 |
| Weighted Average y | 1.03E-05 1.07E-05 |
| Weighted Average z | -9.87E-15 -1.01E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 92 SIMULATION TIME = 9.20000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.52E-04 2.66E-04 |
| Sum x | -6.29E-01 -6.29E-01 |
| Sum y | -2.59E-01 -2.59E-01 |
| Sum z | 8.03E-13 8.03E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.56E-01 7.71E-01 |
| Weighted Average x | 6.17E-03 6.19E-03 |
| Weighted Average y | 2.93E-03 2.92E-03 |
| Weighted Average z | -4.99E-15 -4.74E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.86E-01 1.50E-01 |
| Sum x | -7.47E-01 -7.47E-01 |
| Sum y | -3.19E-01 -3.19E-01 |
| Sum z | 9.55E-13 9.55E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.66E-03 1.72E-03 |
| Weighted Average x | 6.16E-03 6.18E-03 |
| Weighted Average y | 2.92E-03 2.91E-03 |
| Weighted Average z | -5.09E-15 -4.86E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.89E-03 1.51E-03 |
| Sum x | -7.46E-01 -7.46E-01 |
| Sum y | -3.18E-01 -3.18E-01 |
| Sum z | 1.00E-12 1.00E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.74E-05 1.77E-05 |
| Weighted Average x | 6.16E-03 6.18E-03 |
| Weighted Average y | 2.92E-03 2.91E-03 |
| Weighted Average z | -5.07E-15 -4.83E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 93 SIMULATION TIME = 9.30000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.02E-04 1.60E-04 |
| Sum x | -7.46E-01 -7.46E-01 |
| Sum y | -3.18E-01 -3.18E-01 |
| Sum z | 1.00E-12 1.00E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.55E-01 7.71E-01 |
| Weighted Average x | 1.21E-02 1.22E-02 |
| Weighted Average y | 5.39E-03 5.38E-03 |
| Weighted Average z | -1.11E-15 -8.21E-16 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.24E-01 9.58E-02 |
| Sum x | -8.40E-01 -8.40E-01 |
| Sum y | -3.40E-01 -3.40E-01 |
| Sum z | 7.73E-13 7.73E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.27E-03 1.32E-03 |
| Weighted Average x | 1.21E-02 1.21E-02 |
| Weighted Average y | 5.38E-03 5.37E-03 |
| Weighted Average z | -1.30E-15 -1.01E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.56E-03 1.96E-03 |
| Sum x | -8.39E-01 -8.39E-01 |
| Sum y | -3.39E-01 -3.39E-01 |
| Sum z | 1.06E-12 1.06E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.74E-05 1.74E-05 |
| Weighted Average x | 1.21E-02 1.21E-02 |
| Weighted Average y | 5.38E-03 5.37E-03 |
| Weighted Average z | -1.36E-15 -1.06E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 94 SIMULATION TIME = 9.40000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.77E-04 1.36E-04 |
| Sum x | -8.39E-01 -8.39E-01 |
| Sum y | -3.39E-01 -3.39E-01 |
| Sum z | 1.06E-12 1.06E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.56E-01 7.71E-01 |
| Weighted Average x | 1.78E-02 1.79E-02 |
| Weighted Average y | 7.04E-03 7.02E-03 |
| Weighted Average z | -2.99E-15 -2.94E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 9.14E-02 6.91E-02 |
| Sum x | -9.06E-01 -9.06E-01 |
| Sum y | -3.17E-01 -3.17E-01 |
| Sum z | 1.04E-12 1.04E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.12E-03 1.16E-03 |
| Weighted Average x | 1.78E-02 1.79E-02 |
| Weighted Average y | 7.03E-03 7.00E-03 |
| Weighted Average z | -3.06E-15 -3.02E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.99E-03 2.30E-03 |
| Sum x | -9.04E-01 -9.04E-01 |
| Sum y | -3.16E-01 -3.16E-01 |
| Sum z | 1.06E-12 1.06E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.53E-05 1.54E-05 |
| Weighted Average x | 1.78E-02 1.79E-02 |
| Weighted Average y | 7.03E-03 7.00E-03 |
| Weighted Average z | -3.06E-15 -3.01E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 95 SIMULATION TIME = 9.50000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.27E-04 9.51E-05 |
| Sum x | -9.04E-01 -9.04E-01 |
| Sum y | -3.16E-01 -3.16E-01 |
| Sum z | 1.05E-12 1.05E-12 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.57E-01 7.72E-01 |
| Weighted Average x | 2.31E-02 2.31E-02 |
| Weighted Average y | 7.63E-03 7.61E-03 |
| Weighted Average z | -7.96E-15 -8.20E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 8.86E-02 6.65E-02 |
| Sum x | -9.42E-01 -9.42E-01 |
| Sum y | -2.59E-01 -2.59E-01 |
| Sum z | 9.40E-13 9.40E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.07E-03 1.10E-03 |
| Weighted Average x | 2.31E-02 2.31E-02 |
| Weighted Average y | 7.62E-03 7.59E-03 |
| Weighted Average z | -7.66E-15 -7.91E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.49E-03 2.65E-03 |
| Sum x | -9.39E-01 -9.39E-01 |
| Sum y | -2.57E-01 -2.57E-01 |
| Sum z | 9.55E-13 9.55E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.26E-05 1.27E-05 |
| Weighted Average x | 2.31E-02 2.31E-02 |
| Weighted Average y | 7.62E-03 7.59E-03 |
| Weighted Average z | -7.60E-15 -7.86E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 96 SIMULATION TIME = 9.60000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.13E-04 8.39E-05 |
| Sum x | -9.39E-01 -9.39E-01 |
| Sum y | -2.57E-01 -2.57E-01 |
| Sum z | 9.54E-13 9.54E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.58E-01 7.73E-01 |
| Weighted Average x | 2.76E-02 2.76E-02 |
| Weighted Average y | 7.10E-03 7.07E-03 |
| Weighted Average z | -3.89E-15 -4.13E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 9.82E-02 7.46E-02 |
| Sum x | -9.44E-01 -9.44E-01 |
| Sum y | -1.72E-01 -1.72E-01 |
| Sum z | 8.57E-13 8.57E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.02E-03 1.05E-03 |
| Weighted Average x | 2.76E-02 2.76E-02 |
| Weighted Average y | 7.08E-03 7.06E-03 |
| Weighted Average z | -3.58E-15 -3.82E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.28E-03 3.26E-03 |
| Sum x | -9.39E-01 -9.39E-01 |
| Sum y | -1.71E-01 -1.71E-01 |
| Sum z | 9.19E-13 9.19E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.77E-06 9.82E-06 |
| Weighted Average x | 2.76E-02 2.76E-02 |
| Weighted Average y | 7.08E-03 7.06E-03 |
| Weighted Average z | -3.59E-15 -3.82E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 97 SIMULATION TIME = 9.70000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.22E-04 9.10E-05 |
| Sum x | -9.39E-01 -9.39E-01 |
| Sum y | -1.71E-01 -1.71E-01 |
| Sum z | 9.19E-13 9.19E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.59E-01 7.74E-01 |
| Weighted Average x | 3.11E-02 3.11E-02 |
| Weighted Average y | 5.52E-03 5.50E-03 |
| Weighted Average z | 9.65E-15 9.71E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.11E-01 8.59E-02 |
| Sum x | -9.06E-01 -9.06E-01 |
| Sum y | -7.14E-02 -7.14E-02 |
| Sum z | 6.96E-13 6.96E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.00E-03 1.03E-03 |
| Weighted Average x | 3.11E-02 3.11E-02 |
| Weighted Average y | 5.51E-03 5.49E-03 |
| Weighted Average z | 9.48E-15 9.54E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.80E-03 3.68E-03 |
| Sum x | -9.00E-01 -9.00E-01 |
| Sum y | -7.06E-02 -7.06E-02 |
| Sum z | 7.35E-13 7.35E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 7.54E-06 7.57E-06 |
| Weighted Average x | 3.11E-02 3.11E-02 |
| Weighted Average y | 5.51E-03 5.49E-03 |
| Weighted Average z | 9.55E-15 9.63E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 98 SIMULATION TIME = 9.80000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.16E-04 8.70E-05 |
| Sum x | -9.00E-01 -9.00E-01 |
| Sum y | -7.05E-02 -7.05E-02 |
| Sum z | 7.35E-13 7.35E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.74E-01 |
| Weighted Average x | 3.33E-02 3.33E-02 |
| Weighted Average y | 3.15E-03 3.14E-03 |
| Weighted Average z | 1.60E-14 1.63E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.27E-01 9.79E-02 |
| Sum x | -8.27E-01 -8.27E-01 |
| Sum y | 2.90E-02 2.90E-02 |
| Sum z | 5.10E-13 5.10E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.94E-04 1.01E-03 |
| Weighted Average x | 3.32E-02 3.32E-02 |
| Weighted Average y | 3.15E-03 3.14E-03 |
| Weighted Average z | 1.54E-14 1.57E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.23E-03 4.01E-03 |
| Sum x | -8.22E-01 -8.22E-01 |
| Sum y | 2.93E-02 2.93E-02 |
| Sum z | 5.45E-13 5.45E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.10E-06 6.16E-06 |
| Weighted Average x | 3.32E-02 3.32E-02 |
| Weighted Average y | 3.15E-03 3.14E-03 |
| Weighted Average z | 1.54E-14 1.58E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 99 SIMULATION TIME = 9.90000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.05E-04 7.86E-05 |
| Sum x | -8.22E-01 -8.22E-01 |
| Sum y | 2.93E-02 2.93E-02 |
| Sum z | 5.46E-13 5.46E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.60E-01 7.75E-01 |
| Weighted Average x | 3.40E-02 3.40E-02 |
| Weighted Average y | 3.66E-04 3.64E-04 |
| Weighted Average z | 5.40E-15 5.55E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.44E-01 1.11E-01 |
| Sum x | -7.15E-01 -7.15E-01 |
| Sum y | 1.14E-01 1.14E-01 |
| Sum z | 2.49E-13 2.49E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.61E-04 9.79E-04 |
| Weighted Average x | 3.39E-02 3.39E-02 |
| Weighted Average y | 3.67E-04 3.66E-04 |
| Weighted Average z | 4.93E-15 5.06E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 5.83E-03 4.44E-03 |
| Sum x | -7.09E-01 -7.09E-01 |
| Sum y | 1.14E-01 1.14E-01 |
| Sum z | 2.30E-13 2.30E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.47E-06 5.51E-06 |
| Weighted Average x | 3.39E-02 3.39E-02 |
| Weighted Average y | 3.67E-04 3.66E-04 |
| Weighted Average z | 4.98E-15 5.12E-15 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 100 SIMULATION TIME = 1.00000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.75E-04 1.31E-04 |
| Sum x | -7.09E-01 -7.09E-01 |
| Sum y | 1.14E-01 1.14E-01 |
| Sum z | 2.30E-13 2.30E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.59E-01 7.74E-01 |
| Weighted Average x | 3.31E-02 3.31E-02 |
| Weighted Average y | -2.42E-03 -2.41E-03 |
| Weighted Average z | -1.02E-14 -1.04E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.71E-01 1.29E-01 |
| Sum x | -5.74E-01 -5.74E-01 |
| Sum y | 1.73E-01 1.73E-01 |
| Sum z | -1.26E-13 -1.26E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.69E-04 9.92E-04 |
| Weighted Average x | 3.31E-02 3.31E-02 |
| Weighted Average y | -2.41E-03 -2.40E-03 |
| Weighted Average z | -9.85E-15 -1.01E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Not yet converged |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| Solid | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 6.67E-03 4.96E-03 |
| Sum x | -5.69E-01 -5.69E-01 |
| Sum y | 1.73E-01 1.73E-01 |
| Sum z | -1.03E-13 -1.03E-13 |
+-----------------------------------------------------------------------------+
| Fluid | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.61E-06 6.72E-06 |
| Weighted Average x | 3.31E-02 3.31E-02 |
| Weighted Average y | -2.41E-03 -2.40E-03 |
| Weighted Average z | -9.87E-15 -1.01E-14 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| Solid | Converged |
| Fluid | Converged |
+=============================================================================+
===============================================================================
+=============================================================================+
| |
| Shut Down |
| |
+=============================================================================+
===============================================================================
+=============================================================================+
| Available Restart Points |
+=============================================================================+
| Restart Point | File Name |
+-----------------------------------------------------------------------------+
| Coupling Step 1 | Restart_step1.h5 |
| Coupling Step 2 | Restart_step2.h5 |
| Coupling Step 3 | Restart_step3.h5 |
| Coupling Step 4 | Restart_step4.h5 |
| Coupling Step 5 | Restart_step5.h5 |
| Coupling Step 6 | Restart_step6.h5 |
| Coupling Step 7 | Restart_step7.h5 |
| Coupling Step 8 | Restart_step8.h5 |
| Coupling Step 9 | Restart_step9.h5 |
| Coupling Step 10 | Restart_step10.h5 |
| Coupling Step 11 | Restart_step11.h5 |
| Coupling Step 12 | Restart_step12.h5 |
| Coupling Step 13 | Restart_step13.h5 |
| Coupling Step 14 | Restart_step14.h5 |
| Coupling Step 15 | Restart_step15.h5 |
| Coupling Step 16 | Restart_step16.h5 |
| Coupling Step 17 | Restart_step17.h5 |
| Coupling Step 18 | Restart_step18.h5 |
| Coupling Step 19 | Restart_step19.h5 |
| Coupling Step 20 | Restart_step20.h5 |
| Coupling Step 21 | Restart_step21.h5 |
| Coupling Step 22 | Restart_step22.h5 |
| Coupling Step 23 | Restart_step23.h5 |
| Coupling Step 24 | Restart_step24.h5 |
| Coupling Step 25 | Restart_step25.h5 |
| Coupling Step 26 | Restart_step26.h5 |
| Coupling Step 27 | Restart_step27.h5 |
| Coupling Step 28 | Restart_step28.h5 |
| Coupling Step 29 | Restart_step29.h5 |
| Coupling Step 30 | Restart_step30.h5 |
| Coupling Step 31 | Restart_step31.h5 |
| Coupling Step 32 | Restart_step32.h5 |
| Coupling Step 33 | Restart_step33.h5 |
| Coupling Step 34 | Restart_step34.h5 |
| Coupling Step 35 | Restart_step35.h5 |
| Coupling Step 36 | Restart_step36.h5 |
| Coupling Step 37 | Restart_step37.h5 |
| Coupling Step 38 | Restart_step38.h5 |
| Coupling Step 39 | Restart_step39.h5 |
| Coupling Step 40 | Restart_step40.h5 |
| Coupling Step 41 | Restart_step41.h5 |
| Coupling Step 42 | Restart_step42.h5 |
| Coupling Step 43 | Restart_step43.h5 |
| Coupling Step 44 | Restart_step44.h5 |
| Coupling Step 45 | Restart_step45.h5 |
| Coupling Step 46 | Restart_step46.h5 |
| Coupling Step 47 | Restart_step47.h5 |
| Coupling Step 48 | Restart_step48.h5 |
| Coupling Step 49 | Restart_step49.h5 |
| Coupling Step 50 | Restart_step50.h5 |
| Coupling Step 51 | Restart_step51.h5 |
| Coupling Step 52 | Restart_step52.h5 |
| Coupling Step 53 | Restart_step53.h5 |
| Coupling Step 54 | Restart_step54.h5 |
| Coupling Step 55 | Restart_step55.h5 |
| Coupling Step 56 | Restart_step56.h5 |
| Coupling Step 57 | Restart_step57.h5 |
| Coupling Step 58 | Restart_step58.h5 |
| Coupling Step 59 | Restart_step59.h5 |
| Coupling Step 60 | Restart_step60.h5 |
| Coupling Step 61 | Restart_step61.h5 |
| Coupling Step 62 | Restart_step62.h5 |
| Coupling Step 63 | Restart_step63.h5 |
| Coupling Step 64 | Restart_step64.h5 |
| Coupling Step 65 | Restart_step65.h5 |
| Coupling Step 66 | Restart_step66.h5 |
| Coupling Step 67 | Restart_step67.h5 |
| Coupling Step 68 | Restart_step68.h5 |
| Coupling Step 69 | Restart_step69.h5 |
| Coupling Step 70 | Restart_step70.h5 |
| Coupling Step 71 | Restart_step71.h5 |
| Coupling Step 72 | Restart_step72.h5 |
| Coupling Step 73 | Restart_step73.h5 |
| Coupling Step 74 | Restart_step74.h5 |
| Coupling Step 75 | Restart_step75.h5 |
| Coupling Step 76 | Restart_step76.h5 |
| Coupling Step 77 | Restart_step77.h5 |
| Coupling Step 78 | Restart_step78.h5 |
| Coupling Step 79 | Restart_step79.h5 |
| Coupling Step 80 | Restart_step80.h5 |
| Coupling Step 81 | Restart_step81.h5 |
| Coupling Step 82 | Restart_step82.h5 |
| Coupling Step 83 | Restart_step83.h5 |
| Coupling Step 84 | Restart_step84.h5 |
| Coupling Step 85 | Restart_step85.h5 |
| Coupling Step 86 | Restart_step86.h5 |
| Coupling Step 87 | Restart_step87.h5 |
| Coupling Step 88 | Restart_step88.h5 |
| Coupling Step 89 | Restart_step89.h5 |
| Coupling Step 90 | Restart_step90.h5 |
| Coupling Step 91 | Restart_step91.h5 |
| Coupling Step 92 | Restart_step92.h5 |
| Coupling Step 93 | Restart_step93.h5 |
| Coupling Step 94 | Restart_step94.h5 |
| Coupling Step 95 | Restart_step95.h5 |
| Coupling Step 96 | Restart_step96.h5 |
| Coupling Step 97 | Restart_step97.h5 |
| Coupling Step 98 | Restart_step98.h5 |
| Coupling Step 99 | Restart_step99.h5 |
| Coupling Step 100 | Restart_step100.h5 |
+=============================================================================+
+=============================================================================+
| Timing Summary [s] |
+=============================================================================+
| Total Time : 3.72963E+02 |
| Coupling Participant Time |
| Fluid : 2.47391E+02 |
| Solid : 8.20414E+01 |
| Total : 3.29432E+02 |
| Coupling Engine Time |
| Solution Control : 2.34206E+01 |
| Mesh Import : 1.50125E-02 |
| Mapping Setup : 9.70316E-03 |
| Mapping : 3.50602E-02 |
| Numerics : 1.37417E-01 |
| Misc. : 1.99128E+01 |
| Total : 4.35306E+01 |
+=============================================================================+
+=============================================================================+
| System coupling run completed successfully. |
+=============================================================================+
Post-processing#
Post-process the structural results
mapdl.finish()
mapdl.post1()
node_ids, node_coords = mapdl.result.nodal_displacement(0)
max_dx = max([value[0] for value in node_coords])
print(f"There are {len(node_ids)} nodes. Maximum x-displacement is {max_dx}")
There are 2374 nodes. Maximum x-displacement is 0.11232059694684142
Post-process the fluids results
# use_window_resolution option not active inside containers or Ansys Lab environment
if fluent.results.graphics.picture.use_window_resolution.is_active():
fluent.results.graphics.picture.use_window_resolution = False
fluent.results.graphics.picture.x_resolution = 1920
fluent.results.graphics.picture.y_resolution = 1440
fluent.results.graphics.contour["contour_static_pressure"] = {}
contour = fluent.results.graphics.contour["contour_static_pressure"]
contour.coloring.option = "banded"
contour.field = "pressure"
contour.filled = True
contour.surfaces_list = ["symmetry1", "wall_deforming"]
contour.display()
fluent.results.graphics.views.restore_view(view_name="front")
fluent.results.graphics.views.auto_scale()
fluent.results.graphics.picture.save_picture(file_name="oscplate_pressure_contour.png")
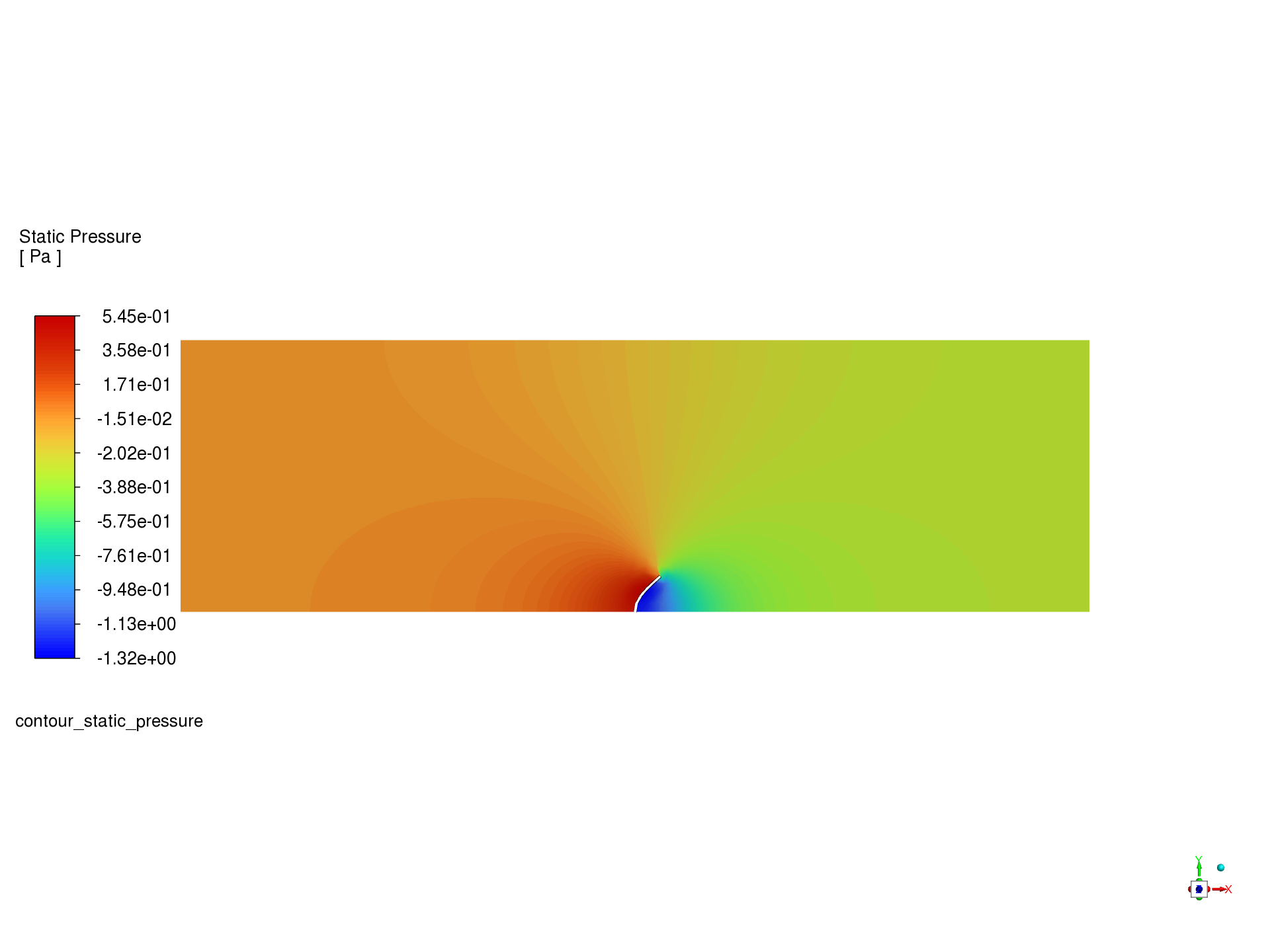
Post-process the System Coupling results - display the charts showing displacement and force values during the simulation
syc.solution.show_plot(show_convergence=False)
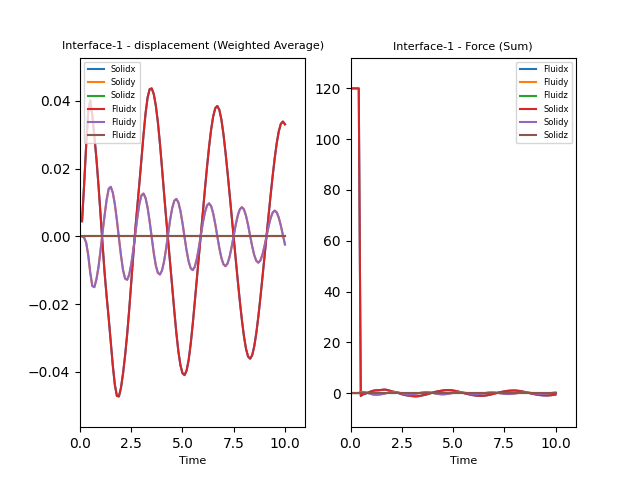
<ansys.systemcoupling.core.charts.plotter.Plotter object at 0x7f8e85ef7890>
Exit#
syc.exit()
fluent.exit()
mapdl.exit()
Total running time of the script: (7 minutes 41.940 seconds)