Note
Go to the end to download the full example code.
FMU-FMU cosimulation of a heating tank & heater#
This demo illustrates a transient coupled simulation of convection heating of a fluid in a cylindrical tank. The heat source and heating tank are controlled via two FMUs that share temperature and heat flow data with one another.
One FMU is used to model the fluid in the tank.
Another FMU is used to model the controlled heat source at the bottom of the tank.
System Coupling coordinates the simultaneous execution of the solvers and the data transfers between them.
Problem description
The tank is modelled as a uniform-temperature fluid heated by a thermostat and experiencing convective cooling at its top. The temperature of the fluid is available as an output, modelling a sensor in the tank. The FMU has six parameters that can be set:
Height and base radius of the cylindrical tank [m]
Density [kg m-3] and specific heat [W kg-1K-1] of the fluid (by default, set to the properties of water)
Convection heat transfer coefficient between the fluid and its surroundings [W m-2K-1]
Temperature of the tank’s surroundings [K].
The thermostat receives a temperature from the tank sensor and outputs a heat-rate. The FMU uses PID (proprtional-integral-derivative) control to determine the heat output and has five parameters that can be set:
Target temperature [K]
Maximum heat output [W]
Heat scale proportional factor [W/K]
Heat scale integral factor [W K-1s-1]
Heat scale derivative factor [W s K-1]
One coupling interface between the FMUs with two data transfers :
temperature
heat flow
# Tags: FMU, transient
Import modules, download files, launch products#
Setting up this example consists of importing required modules, downloading the input files, and launching the required products.
Perform required imports#
Import ansys-systemcoupling-core
.
import ansys.systemcoupling.core as pysystemcoupling
from ansys.systemcoupling.core import examples
Download the input files#
This example requires the two FMU files to be downloaded.
examples.delete_downloads()
fmu_file_heater = examples.download_file(
"thermostat.fmu", "pysystem-coupling/heating_tank_fmu/FMU"
)
fmu_file_tank = examples.download_file(
"heatingTank.fmu", "pysystem-coupling/heating_tank_fmu_fmu/FMU"
)
Launch System Coupling#
Launch a remote System Coupling instance and return a client object
(a Session
object) that allows you to interact with System Coupling
via an API exposed into the current Python environment.
syc = pysystemcoupling.launch(start_output=True)
Set up the coupled analysis#
System Coupling setup involves adding the two FMU participants, adding coupled interfaces and data transfers, and setting other coupled analysis properties.
Add participants by passing session handles to System Coupling.
heater_part_name = syc.setup.add_participant(input_file=fmu_file_heater)
tank_part_name = syc.setup.add_participant(input_file=fmu_file_tank)
Set FMU settings#
# Access the heater participant object
heater_participant = syc.setup.coupling_participant[heater_part_name]
# Change the "maximum heat output" settings
max_heat_output_param = heater_participant.fmu_parameter["Real_2"]
max_heat_output_param.real_value = 1000
# Change the "target temperature" settings
target_temperature_param = heater_participant.fmu_parameter["Real_3"]
target_temperature_param.real_value = 350
# Change the "heat scale proportional factor" settings
heat_p_factor_param = heater_participant.fmu_parameter["Real_4"]
heat_p_factor_param.real_value = 400
# Change the "heat scale integral factor" settings
heat_i_factor_param = heater_participant.fmu_parameter["Real_5"]
heat_i_factor_param.real_value = 0
# Change the "heat scale derivative factor" settings
heat_d_factor_param = heater_participant.fmu_parameter["Real_6"]
heat_d_factor_param.real_value = 0
# Access the heating tank participant object
tank_participant = syc.setup.coupling_participant[tank_part_name]
# Change the "tank height" settings
tank_height_param = tank_participant.fmu_parameter["Real_2"]
tank_height_param.real_value = 0.14
# Change the "tank radius" settings
tank_radius_param = tank_participant.fmu_parameter["Real_3"]
tank_radius_param.real_value = 0.05
# Change the "fluid density" settings
fluid_density_param = tank_participant.fmu_parameter["Real_4"]
fluid_density_param.real_value = 998.2
# Change the "fluid heat capacity" settings
fluid_heat_capacity_param = tank_participant.fmu_parameter["Real_5"]
fluid_heat_capacity_param.real_value = 4182
# Change the "convection heat transfer coefficient" settings
convection_coeff_param = tank_participant.fmu_parameter["Real_6"]
convection_coeff_param.real_value = 10
# Change the "surrounding temperature" settings
surrounding_temp = tank_participant.fmu_parameter["Real_7"]
surrounding_temp.real_value = 295
Add a coupling interface and data transfers#
# Add a coupling interface for tank <-> heater (sensor, heat source)
fmu_interface_name = syc.setup.add_interface(
side_one_participant=tank_part_name, side_two_participant=heater_part_name
)
# Add the temperature data transfer
temperature_transfer_name = syc.setup.add_data_transfer(
interface=fmu_interface_name,
target_side="Two",
source_variable="Real_0",
target_variable="Real_0",
)
# Add the heat flow data transfer
heatflow_transfer_name = syc.setup.add_data_transfer(
interface=fmu_interface_name,
target_side="One",
source_variable="Real_1",
target_variable="Real_1",
)
Other controls
# Set time step size
syc.setup.solution_control.time_step_size = "4 [s]"
# Set the simulation end time
syc.setup.solution_control.end_time = "400 [s]"
# Set minimum and maximum number of iterations to 1
# to emulate explicit update.
syc.setup.solution_control.minimum_iterations = 1
syc.setup.solution_control.maximum_iterations = 1
# Turn on chart output. This step is necessary
# to chart the data after solving.
syc.setup.output_control.generate_csv_chart_output = True
Solution#
syc.solution.solve()
+=============================================================================+
| Coupling Participants (2) |
+=============================================================================+
| tutorialHeater |
+-----------------------------------------------------------------------------+
| Internal Name : FMU-1 |
| Participant Type : FMU |
| Participant Display Name : tutorialHeater |
| Input Variables : [Real_0] |
| Output Variables : [Real_1] |
| Participant File Loaded : thermostat.fmu |
| Logging On : False |
| Can Serialize Fmu State : True |
| Can Get And Set Fmu State : True |
| Variables (2) |
| Variable : Heat_Rate |
| Internal Name : Real_1 |
| Participant Display Name : Heat Rate |
| Data Type : Real |
| Real Initial Value : 1.00e+03 |
| Real Min : None |
| Real Max : None |
| |
| Variable : Temperature |
| Internal Name : Real_0 |
| Participant Display Name : Temperature |
| Data Type : Real |
| Real Initial Value : 3.00e+02 |
| Real Min : None |
| Real Max : None |
| Update Control |
| Option : ProgramControlled |
| FMU Parameter |
| FMUParameter : Heat_Scale_Derivative_Factor |
| Internal Name : Real_6 |
| Data Type : Real |
| Participant Display Name : Heat Scale Derivative Factor |
| Real Value : 0.00e+00 |
| Real Min : None |
| Real Max : None |
| FMUParameter : Heat_Scale_Integral_Factor |
| Internal Name : Real_5 |
| Data Type : Real |
| Participant Display Name : Heat Scale Integral Factor |
| Real Value : 0.00e+00 |
| Real Min : None |
| Real Max : None |
| FMUParameter : Heat_Scale_Proportional_Factor |
| Internal Name : Real_4 |
| Data Type : Real |
| Participant Display Name : Heat Scale Proportional Factor |
| Real Value : 4.00e+02 |
| Real Min : None |
| Real Max : None |
| FMUParameter : Maximum_Heat_Output |
| Internal Name : Real_2 |
| Data Type : Real |
| Participant Display Name : Maximum Heat Output |
| Real Value : 1.00e+03 |
| Real Min : None |
| Real Max : None |
| FMUParameter : Target_Temperature |
| Internal Name : Real_3 |
| Data Type : Real |
| Participant Display Name : Target Temperature |
| Real Value : 3.50e+02 |
| Real Min : None |
| Real Max : None |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank |
+-----------------------------------------------------------------------------+
| Internal Name : FMU-2 |
| Participant Type : FMU |
| Participant Display Name : tutorialHeatingTank |
| Input Variables : [Real_1] |
| Output Variables : [Real_0] |
| Participant File Loaded : heatingTank.fmu |
| Logging On : False |
| Can Serialize Fmu State : True |
| Can Get And Set Fmu State : True |
| Variables (2) |
| Variable : Heat_Rate |
| Internal Name : Real_1 |
| Participant Display Name : Heat Rate |
| Data Type : Real |
| Real Initial Value : 1.00e+01 |
| Real Min : None |
| Real Max : None |
| |
| Variable : Temperature |
| Internal Name : Real_0 |
| Participant Display Name : Temperature |
| Data Type : Real |
| Real Initial Value : 2.73e+02 |
| Real Min : None |
| Real Max : None |
| Update Control |
| Option : ProgramControlled |
| FMU Parameter |
| FMUParameter : Convection_Coefficient |
| Internal Name : Real_6 |
| Data Type : Real |
| Participant Display Name : Convection Coefficient |
| Real Value : 1.00e+01 |
| Real Min : None |
| Real Max : None |
| FMUParameter : Density |
| Internal Name : Real_4 |
| Data Type : Real |
| Participant Display Name : Density |
| Real Value : 9.98e+02 |
| Real Min : None |
| Real Max : None |
| FMUParameter : Specific_Heat |
| Internal Name : Real_5 |
| Data Type : Real |
| Participant Display Name : Specific Heat |
| Real Value : 4.18e+03 |
| Real Min : None |
| Real Max : None |
| FMUParameter : Surroundings_Temp |
| Internal Name : Real_7 |
| Data Type : Real |
| Participant Display Name : Surroundings Temp |
| Real Value : 2.95e+02 |
| Real Min : None |
| Real Max : None |
| FMUParameter : Tank_Height |
| Internal Name : Real_2 |
| Data Type : Real |
| Participant Display Name : Tank Height |
| Real Value : 1.40e-01 |
| Real Min : None |
| Real Max : None |
| FMUParameter : Tank_Radius |
| Internal Name : Real_3 |
| Data Type : Real |
| Participant Display Name : Tank Radius |
| Real Value : 5.00e-02 |
| Real Min : None |
| Real Max : None |
+=============================================================================+
| Analysis Control |
+=============================================================================+
| Analysis Type : Transient |
| Optimize If One Way : True |
| Allow Simultaneous Update : False |
+=============================================================================+
| Coupling Interfaces (1) |
+=============================================================================+
| Interface-1 |
+-----------------------------------------------------------------------------+
| Internal Name : Interface-1 |
| Side |
| Side: One |
| Coupling Participant : FMU-2 |
| Side: Two |
| Coupling Participant : FMU-1 |
| Data Transfers (2) |
| DataTransfer : Heat_Rate |
| Internal Name : Real_1 |
| Suppress : False |
| Target Side : One |
| Option : UsingVariable |
| Source Variable : Real_1 |
| Target Variable : Real_1 |
| Ramping Option : None |
| Relaxation Factor : 1.00e+00 |
| Convergence Target : 1.00e-02 |
| DataTransfer : Temperature |
| Internal Name : Real_0 |
| Suppress : False |
| Target Side : Two |
| Option : UsingVariable |
| Source Variable : Real_0 |
| Target Variable : Real_0 |
| Ramping Option : None |
| Relaxation Factor : 1.00e+00 |
| Convergence Target : 1.00e-02 |
+=============================================================================+
| Solution Control |
+=============================================================================+
| Duration Option : EndTime |
| End Time : 400 [s] |
| Time Step Size : 4 [s] |
| Minimum Iterations : 1 |
| Maximum Iterations : 1 |
+=============================================================================+
| Output Control |
+=============================================================================+
| Generate CSV Chart Output : True |
| Write Initial Snapshot : True |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warnings were found during data model validation. |
+-----------------------------------------------------------------------------+
| Warning: FMU(s) detected in the Datamodel. FMUs are not currently |
| restartable. A restart can still be performed but the FMU state will be |
| re-initialized based on the Datamodel. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| Execution Information |
+=============================================================================+
| |
| System Coupling |
| Command Line Arguments: |
| -m cosimgui --grpcport=0.0.0.0:35397 |
| Working Directory: |
| /working |
| |
| tutorialHeater |
| thermostat.fmu |
| |
| tutorialHeatingTank |
| heatingTank.fmu |
| |
+=============================================================================+
Awaiting connections from coupling participants... done.
+=============================================================================+
| Build Information |
+-----------------------------------------------------------------------------+
| System Coupling |
| 2025 R2: Build ID: 0f637b7 Build Date: 2025-05-16T16:59 |
| tutorialHeater |
| Author: ANSYS Inc |
| Version: 1.0 |
| Copyright: ANSYS Inc |
| License: None |
| Generation Time: 2019-02-08T09:52:33z |
| Description: Thermostat FMU for Tutorial |
| tutorialHeatingTank |
| Author: ANSYS Inc |
| Version: 1.0 |
| Copyright: ANSYS Inc |
| License: None |
| Description: Heating Tank FMU for Tutorial |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Transfer Diagnostics |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | |
| Value | 3.00E+02 3.00E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | |
| Value | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
===============================================================================
+=============================================================================+
| |
| Analysis Initialization |
| |
+=============================================================================+
===============================================================================
===============================================================================
+=============================================================================+
| |
| Coupled Solution |
| |
+=============================================================================+
===============================================================================
+=============================================================================+
| COUPLING STEP = 1 SIMULATION TIME = 4.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Not yet converged |
| RMS Change | 1.00E+00 1.00E+00 |
| Value | 3.00E+02 3.00E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 1.00E+00 1.00E+00 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 2 SIMULATION TIME = 8.00000E+00 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.90E-03 2.90E-03 |
| Value | 3.01E+02 3.01E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 3 SIMULATION TIME = 1.20000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.89E-03 2.89E-03 |
| Value | 3.02E+02 3.02E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 4 SIMULATION TIME = 1.60000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.88E-03 2.88E-03 |
| Value | 3.03E+02 3.03E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 5 SIMULATION TIME = 2.00000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.87E-03 2.87E-03 |
| Value | 3.03E+02 3.03E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 6 SIMULATION TIME = 2.40000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.86E-03 2.86E-03 |
| Value | 3.04E+02 3.04E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 7 SIMULATION TIME = 2.80000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.85E-03 2.85E-03 |
| Value | 3.05E+02 3.05E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 8 SIMULATION TIME = 3.20000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.84E-03 2.84E-03 |
| Value | 3.06E+02 3.06E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 9 SIMULATION TIME = 3.60000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.84E-03 2.84E-03 |
| Value | 3.07E+02 3.07E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 10 SIMULATION TIME = 4.00000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.83E-03 2.83E-03 |
| Value | 3.08E+02 3.08E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 11 SIMULATION TIME = 4.40000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.82E-03 2.82E-03 |
| Value | 3.09E+02 3.09E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 12 SIMULATION TIME = 4.80000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.81E-03 2.81E-03 |
| Value | 3.10E+02 3.10E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 13 SIMULATION TIME = 5.20000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.80E-03 2.80E-03 |
| Value | 3.10E+02 3.10E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 14 SIMULATION TIME = 5.60000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.80E-03 2.80E-03 |
| Value | 3.11E+02 3.11E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 15 SIMULATION TIME = 6.00000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.79E-03 2.79E-03 |
| Value | 3.12E+02 3.12E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 16 SIMULATION TIME = 6.40000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.78E-03 2.78E-03 |
| Value | 3.13E+02 3.13E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 17 SIMULATION TIME = 6.80000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.77E-03 2.77E-03 |
| Value | 3.14E+02 3.14E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 18 SIMULATION TIME = 7.20000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.76E-03 2.76E-03 |
| Value | 3.15E+02 3.15E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 19 SIMULATION TIME = 7.60000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.76E-03 2.76E-03 |
| Value | 3.16E+02 3.16E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 20 SIMULATION TIME = 8.00000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.75E-03 2.75E-03 |
| Value | 3.17E+02 3.17E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 21 SIMULATION TIME = 8.40000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.74E-03 2.74E-03 |
| Value | 3.17E+02 3.17E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 22 SIMULATION TIME = 8.80000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.73E-03 2.73E-03 |
| Value | 3.18E+02 3.18E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 23 SIMULATION TIME = 9.20000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.73E-03 2.73E-03 |
| Value | 3.19E+02 3.19E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 24 SIMULATION TIME = 9.60000E+01 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.72E-03 2.72E-03 |
| Value | 3.20E+02 3.20E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 25 SIMULATION TIME = 1.00000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.71E-03 2.71E-03 |
| Value | 3.21E+02 3.21E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 26 SIMULATION TIME = 1.04000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.70E-03 2.70E-03 |
| Value | 3.22E+02 3.22E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 27 SIMULATION TIME = 1.08000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.70E-03 2.70E-03 |
| Value | 3.23E+02 3.23E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 28 SIMULATION TIME = 1.12000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.69E-03 2.69E-03 |
| Value | 3.23E+02 3.23E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 29 SIMULATION TIME = 1.16000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.68E-03 2.68E-03 |
| Value | 3.24E+02 3.24E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 30 SIMULATION TIME = 1.20000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.67E-03 2.67E-03 |
| Value | 3.25E+02 3.25E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 31 SIMULATION TIME = 1.24000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.67E-03 2.67E-03 |
| Value | 3.26E+02 3.26E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 32 SIMULATION TIME = 1.28000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.66E-03 2.66E-03 |
| Value | 3.27E+02 3.27E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 33 SIMULATION TIME = 1.32000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.65E-03 2.65E-03 |
| Value | 3.28E+02 3.28E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 34 SIMULATION TIME = 1.36000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.64E-03 2.64E-03 |
| Value | 3.29E+02 3.29E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 35 SIMULATION TIME = 1.40000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.64E-03 2.64E-03 |
| Value | 3.30E+02 3.30E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 36 SIMULATION TIME = 1.44000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.63E-03 2.63E-03 |
| Value | 3.30E+02 3.30E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 37 SIMULATION TIME = 1.48000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.62E-03 2.62E-03 |
| Value | 3.31E+02 3.31E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 38 SIMULATION TIME = 1.52000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.62E-03 2.62E-03 |
| Value | 3.32E+02 3.32E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 39 SIMULATION TIME = 1.56000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.61E-03 2.61E-03 |
| Value | 3.33E+02 3.33E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 40 SIMULATION TIME = 1.60000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.60E-03 2.60E-03 |
| Value | 3.34E+02 3.34E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 41 SIMULATION TIME = 1.64000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.59E-03 2.59E-03 |
| Value | 3.35E+02 3.35E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 42 SIMULATION TIME = 1.68000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.59E-03 2.59E-03 |
| Value | 3.36E+02 3.36E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 43 SIMULATION TIME = 1.72000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.58E-03 2.58E-03 |
| Value | 3.37E+02 3.37E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 44 SIMULATION TIME = 1.76000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.57E-03 2.57E-03 |
| Value | 3.37E+02 3.37E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 45 SIMULATION TIME = 1.80000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.57E-03 2.57E-03 |
| Value | 3.38E+02 3.38E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 46 SIMULATION TIME = 1.84000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.56E-03 2.56E-03 |
| Value | 3.39E+02 3.39E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 47 SIMULATION TIME = 1.88000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.55E-03 2.55E-03 |
| Value | 3.40E+02 3.40E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 48 SIMULATION TIME = 1.92000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.55E-03 2.55E-03 |
| Value | 3.41E+02 3.41E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 49 SIMULATION TIME = 1.96000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.54E-03 2.54E-03 |
| Value | 3.42E+02 3.42E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 50 SIMULATION TIME = 2.00000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.53E-03 2.53E-03 |
| Value | 3.43E+02 3.43E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 51 SIMULATION TIME = 2.04000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.53E-03 2.53E-03 |
| Value | 3.43E+02 3.43E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 52 SIMULATION TIME = 2.08000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.52E-03 2.52E-03 |
| Value | 3.44E+02 3.44E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 53 SIMULATION TIME = 2.12000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.51E-03 2.51E-03 |
| Value | 3.45E+02 3.45E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 54 SIMULATION TIME = 2.16000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.51E-03 2.51E-03 |
| Value | 3.46E+02 3.46E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 55 SIMULATION TIME = 2.20000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.50E-03 2.50E-03 |
| Value | 3.47E+02 3.47E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.00E-14 1.00E-14 |
| Value | 1.00E+03 1.00E+03 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 56 SIMULATION TIME = 2.24000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.50E-03 2.50E-03 |
| Value | 3.48E+02 3.48E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 1.48E-01 1.48E-01 |
| Value | 8.71E+02 8.71E+02 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 57 SIMULATION TIME = 2.28000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.17E-03 2.17E-03 |
| Value | 3.49E+02 3.49E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 5.31E-01 5.31E-01 |
| Value | 5.69E+02 5.69E+02 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 58 SIMULATION TIME = 2.32000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.41E-03 1.41E-03 |
| Value | 3.49E+02 3.49E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 5.29E-01 5.29E-01 |
| Value | 3.72E+02 3.72E+02 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 59 SIMULATION TIME = 2.36000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 9.17E-04 9.17E-04 |
| Value | 3.49E+02 3.49E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 5.26E-01 5.26E-01 |
| Value | 2.44E+02 2.44E+02 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 60 SIMULATION TIME = 2.40000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 5.97E-04 5.97E-04 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 5.21E-01 5.21E-01 |
| Value | 1.60E+02 1.60E+02 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 61 SIMULATION TIME = 2.44000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 3.89E-04 3.89E-04 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 5.13E-01 5.13E-01 |
| Value | 1.06E+02 1.06E+02 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 62 SIMULATION TIME = 2.48000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.53E-04 2.53E-04 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 5.02E-01 5.02E-01 |
| Value | 7.05E+01 7.05E+01 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 63 SIMULATION TIME = 2.52000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.65E-04 1.65E-04 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 4.87E-01 4.87E-01 |
| Value | 4.74E+01 4.74E+01 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 64 SIMULATION TIME = 2.56000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.07E-04 1.07E-04 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 4.64E-01 4.64E-01 |
| Value | 3.24E+01 3.24E+01 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 65 SIMULATION TIME = 2.60000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 6.99E-05 6.99E-05 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 4.33E-01 4.33E-01 |
| Value | 2.26E+01 2.26E+01 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 66 SIMULATION TIME = 2.64000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 4.56E-05 4.56E-05 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 3.93E-01 3.93E-01 |
| Value | 1.62E+01 1.62E+01 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 67 SIMULATION TIME = 2.68000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.97E-05 2.97E-05 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 3.44E-01 3.44E-01 |
| Value | 1.21E+01 1.21E+01 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 68 SIMULATION TIME = 2.72000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.93E-05 1.93E-05 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 2.89E-01 2.89E-01 |
| Value | 9.37E+00 9.37E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 69 SIMULATION TIME = 2.76000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.26E-05 1.26E-05 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 2.32E-01 2.32E-01 |
| Value | 7.61E+00 7.61E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 70 SIMULATION TIME = 2.80000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 8.20E-06 8.20E-06 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 1.78E-01 1.78E-01 |
| Value | 6.46E+00 6.46E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 71 SIMULATION TIME = 2.84000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 5.34E-06 5.34E-06 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 1.31E-01 1.31E-01 |
| Value | 5.72E+00 5.72E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 72 SIMULATION TIME = 2.88000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 3.48E-06 3.48E-06 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 9.31E-02 9.31E-02 |
| Value | 5.23E+00 5.23E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 73 SIMULATION TIME = 2.92000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.27E-06 2.27E-06 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 6.46E-02 6.46E-02 |
| Value | 4.91E+00 4.91E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 74 SIMULATION TIME = 2.96000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.48E-06 1.48E-06 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 4.39E-02 4.39E-02 |
| Value | 4.70E+00 4.70E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 75 SIMULATION TIME = 3.00000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 9.61E-07 9.61E-07 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 2.94E-02 2.94E-02 |
| Value | 4.57E+00 4.57E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 76 SIMULATION TIME = 3.04000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 6.26E-07 6.26E-07 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 1.96E-02 1.96E-02 |
| Value | 4.48E+00 4.48E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 77 SIMULATION TIME = 3.08000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 4.08E-07 4.08E-07 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Not yet converged |
| RMS Change | 1.29E-02 1.29E-02 |
| Value | 4.43E+00 4.43E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warning: Analysis is not fully converged within the current step. Results |
| may not be accurate. |
+-----------------------------------------------------------------------------+
+=============================================================================+
| COUPLING STEP = 78 SIMULATION TIME = 3.12000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.66E-07 2.66E-07 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 8.47E-03 8.47E-03 |
| Value | 4.39E+00 4.39E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 79 SIMULATION TIME = 3.16000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.73E-07 1.73E-07 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 5.55E-03 5.55E-03 |
| Value | 4.36E+00 4.36E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 80 SIMULATION TIME = 3.20000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.13E-07 1.13E-07 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 3.63E-03 3.63E-03 |
| Value | 4.35E+00 4.35E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 81 SIMULATION TIME = 3.24000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 7.34E-08 7.34E-08 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 2.37E-03 2.37E-03 |
| Value | 4.34E+00 4.34E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 82 SIMULATION TIME = 3.28000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 4.78E-08 4.78E-08 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.55E-03 1.55E-03 |
| Value | 4.33E+00 4.33E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 83 SIMULATION TIME = 3.32000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 3.11E-08 3.11E-08 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.01E-03 1.01E-03 |
| Value | 4.33E+00 4.33E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 84 SIMULATION TIME = 3.36000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.03E-08 2.03E-08 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 6.57E-04 6.57E-04 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 85 SIMULATION TIME = 3.40000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.32E-08 1.32E-08 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 4.28E-04 4.28E-04 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 86 SIMULATION TIME = 3.44000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 8.61E-09 8.61E-09 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 2.79E-04 2.79E-04 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 87 SIMULATION TIME = 3.48000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 5.61E-09 5.61E-09 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.82E-04 1.82E-04 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 88 SIMULATION TIME = 3.52000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 3.65E-09 3.65E-09 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.18E-04 1.18E-04 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 89 SIMULATION TIME = 3.56000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.38E-09 2.38E-09 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 7.71E-05 7.71E-05 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 90 SIMULATION TIME = 3.60000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.55E-09 1.55E-09 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 5.02E-05 5.02E-05 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 91 SIMULATION TIME = 3.64000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.01E-09 1.01E-09 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 3.27E-05 3.27E-05 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 92 SIMULATION TIME = 3.68000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 6.57E-10 6.57E-10 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 2.13E-05 2.13E-05 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 93 SIMULATION TIME = 3.72000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 4.28E-10 4.28E-10 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.39E-05 1.39E-05 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 94 SIMULATION TIME = 3.76000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.79E-10 2.79E-10 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 9.04E-06 9.04E-06 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 95 SIMULATION TIME = 3.80000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.82E-10 1.82E-10 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 5.89E-06 5.89E-06 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 96 SIMULATION TIME = 3.84000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 1.18E-10 1.18E-10 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 3.83E-06 3.83E-06 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 97 SIMULATION TIME = 3.88000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 7.71E-11 7.71E-11 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 2.50E-06 2.50E-06 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 98 SIMULATION TIME = 3.92000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 5.02E-11 5.02E-11 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.63E-06 1.63E-06 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 99 SIMULATION TIME = 3.96000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 3.27E-11 3.27E-11 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 1.06E-06 1.06E-06 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 100 SIMULATION TIME = 4.00000E+02 [s] |
+=============================================================================+
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| tutorialHeater | |
| Interface: Interface-1 | |
| Temperature | Converged |
| RMS Change | 2.13E-11 2.13E-11 |
| Value | 3.50E+02 3.50E+02 |
+-----------------------------------------------------------------------------+
| tutorialHeatingTank | |
| Interface: Interface-1 | |
| Heat_Rate | Converged |
| RMS Change | 6.90E-07 6.90E-07 |
| Value | 4.32E+00 4.32E+00 |
+-----------------------------------------------------------------------------+
| Participant solution status | |
| tutorialHeater | Convergence not evaluated |
| tutorialHeatingTank | Convergence not evaluated |
+=============================================================================+
===============================================================================
+=============================================================================+
| |
| Shut Down |
| |
+=============================================================================+
===============================================================================
+=============================================================================+
| Available Restart Points |
+=============================================================================+
| Restart Point | File Name |
+-----------------------------------------------------------------------------+
| Coupling Step 100 | Restart_step100.h5 |
+=============================================================================+
+=============================================================================+
| Timing Summary [s] |
+=============================================================================+
| Total Time : 4.01580E+00 |
| Coupling Participant Time |
| tutorialHeater : 9.46997E-01 |
| tutorialHeatingTank : 8.47463E-01 |
| Total : 1.79446E+00 |
Post-processing#
Plot graphs of temperature and heat rate over time using System Coupling’s charting command.
syc.solution.show_plot(interface_name=fmu_interface_name, show_convergence=False)
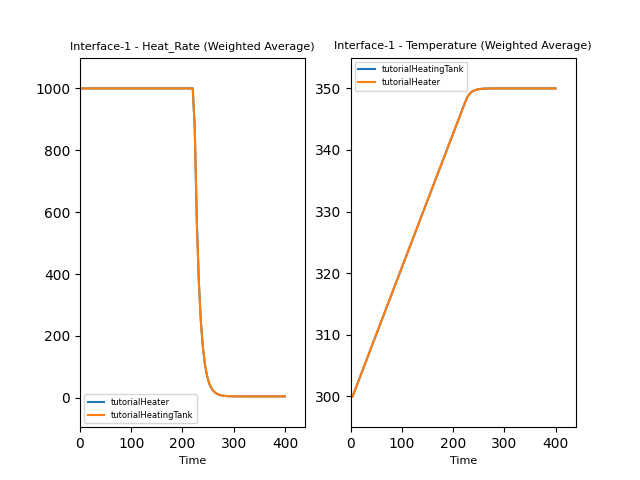
| Solution Control : 2.00932E+00 |
| Mesh Import : 0.00000E+00 |
| Mapping Setup : 3.66926E-04 |
| Mapping : 1.53041E-03 |
| Numerics : 6.32739E-03 |
| Misc. : 2.03789E-01 |
| Total : 2.22134E+00 |
+=============================================================================+
+=============================================================================+
| System coupling run completed successfully. |
+=============================================================================+
<ansys.systemcoupling.core.charts.plotter.Plotter object at 0x7fa244dac290>
Exit#
syc.exit()
Total running time of the script: (0 minutes 39.228 seconds)