Note
Go to the end to download the full example code.
Turek-Hron FSI2 Benchmark Example#
This example is a version of the Turek-Hron FSI2 case that is often used as a benchmark case for System Coupling. This two-way, fluid-structure interaction (FSI) case is based on co-simulation of a transient oscillating beam with surface data transfers.
Ansys Mechanical APDL (MAPDL) is used to perform a transient structural analysis.
Ansys Fluent is used to perform a transient fluid-flow analysis.
System Coupling coordinates the coupled solution involving the above products to solve the multiphysics problem via co-simulation.
Problem description
An elastic beam structure is attached to a rigid cylinder. The system resides within a fluid filled channel:
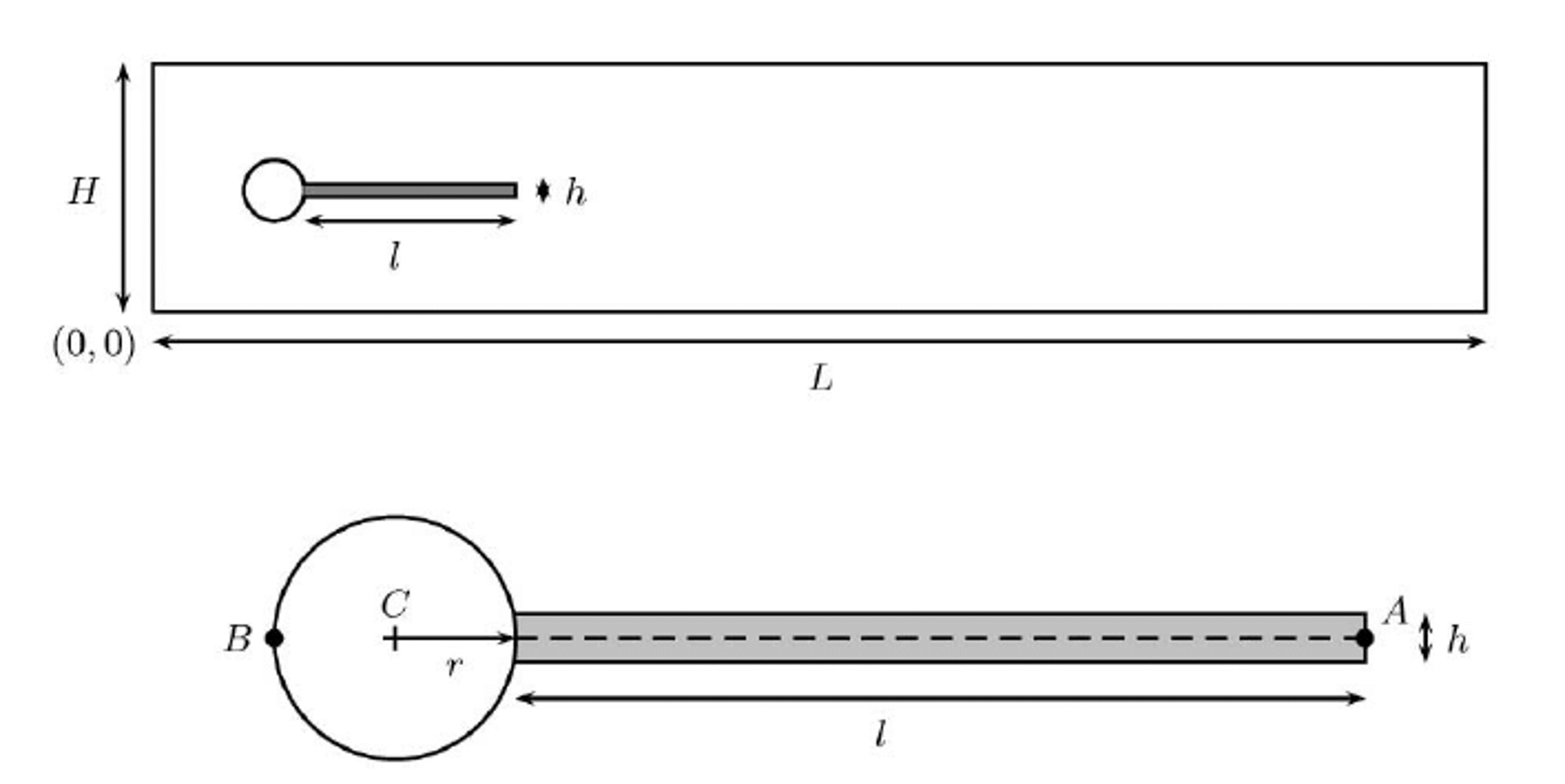
The flow is laminar with a Reynolds number of \(Re = 100\). The inlet velocity has a parabolic profile with a maximum value of \(1.5 \cdot \bar{U}\), where \(\bar{U}\) is the average inlet velocity. The cylinder sits at an offset of \(0.05~m\) to the incoming flow, causing an imbalance of surface forces on the elastic beam. The beam and the surrounding fluid are simulated for a few time setps to allow an examination of the motion of the beam as it starts vibrating due to vortices shedded by the rigid cylinder.
Import modules, download files, launch products#
Setting up this example consists of performing imports, downloading the input file, and launching the required products.
Perform required imports#
Import ansys-systemcoupling-core
, ansys-fluent-core
and
ansys-mapdl-core
and other required packages.
import ansys.fluent.core as pyfluent
import ansys.mapdl.core as pymapdl
import matplotlib.pyplot as plt
import ansys.systemcoupling.core as pysyc
from ansys.systemcoupling.core import examples
Download the input file#
This example uses one pre-created file - a Fluent mesh file that contains the fluids mesh and named zones.
fluent_msh_file = examples.download_file(
"turek_hron_fluid.msh", "pysystem-coupling/turek-horn-benchmark"
)
mapdl_cdb_file = examples.download_file(
"turek_hron_solid_model.cdb", "pysystem-coupling/turek-horn-benchmark"
)
Launch products#
Launch instances of the Mechanical APDL, Fluent, and System Coupling and return client (session) objects that allow you to interact with these products via APIs exposed into the current Python environment.
mapdl = pymapdl.launch_mapdl()
fluent = pyfluent.launch_fluent()
syc = pysyc.launch(start_output=True)
Setup Mechanical APDL, Fluent, and System Coupling analyses#
The setup consists of setting up the structural analysis, the fluids analysis, and the coupled analysis.
Define constants#
CYLINDER_DIA = 0.1 # Diameter of the cylinder
RE = 100 # Reynolds number
U_BAR = 1 # Average velocity
FLUID_DENS = 1000 # Fluid density
SOLID_DENS = 10000 # Solid density
NU = 0.4 # Poisson's ratio
G = 500000 # Shear modulus
E = 2 * G * (1 + NU) # Youngs modulus
Clear cache#
mapdl.clear()
Enter Mechancal APDL setup#
mapdl.prep7()
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 24.2 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 10:11:18 OCT 28, 2024 CP= 1.379
***** MAPDL ANALYSIS DEFINITION (PREP7) *****
Read the CDB file#
mapdl.cdread(option="DB", fname=mapdl_cdb_file)
Define material properties.#
mapdl.mp("DENS", 1, SOLID_DENS) # density
mapdl.mp("EX", 1, E) # Young's modulus
mapdl.mp("NUXY", 1, NU) # Poisson's ratio
mapdl.mp("GXY", 1, G) # Shear modulus
MATERIAL 1 GXY = 500000.0
Mechanical solver setup#
mapdl.slashsolu()
mapdl.antype(4)
mapdl.nlgeom("on")
mapdl.kbc(1)
mapdl.eqslv("sparse")
mapdl.run("rstsuppress,none") # don't suppress anything due to presence of FSI loading
mapdl.dmpoption("emat", "no")
mapdl.dmpoption("esav", "no")
mapdl.cmwrite() # Export components due to presence of FSI loading
mapdl.trnopt(tintopt="hht")
mapdl.tintp("mosp") # No such option in docs
mapdl.nldiag("cont", "iter")
mapdl.scopt("NO")
mapdl.autots("on") # User turned on automatic time stepping
mapdl.nsubst(1, 1, 1, "OFF")
mapdl.time(20.0) # Max time set to any high value; will be controlled by syc
mapdl.timint("on")
mapdl.outres("all", "all")
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
Set up the fluid analysis#
Read the pre-created mesh file
fluent.file.read_mesh(file_name=fluent_msh_file)
fluent.mesh.check()
Reading "turek_hron_fluid.msh"...
Buffering for file scan...
13932 nodes, binary.
13218 quadrilateral interior faces, zone 1, binary.
36 quadrilateral velocity-inlet faces, zone 5, binary.
60 quadrilateral pressure-outlet faces, zone 6, binary.
196 quadrilateral wall faces, zone 7, binary.
100 quadrilateral wall faces, zone 8, binary.
84 quadrilateral wall faces, zone 9, binary.
6728 quadrilateral symmetry faces, zone 10, binary.
6728 quadrilateral symmetry faces, zone 11, binary.
6728 hexahedral cells, zone 2, binary.
Building...
mesh
materials,
interface,
domains,
zones,
symmetry_top
symmetry_bot
fsi
cylinder
wall
outlet
inlet
d8b0bb73-1d68-4795-9b6f-6b2467eb5a7b_fluid
interior-d8b0bb73-1d68-4795-9b6f-6b2467eb5a7b_fluid
parallel,
Done.
Domain Extents:
x-coordinate: min (m) = -2.000000e-01, max (m) = 2.300000e+00
y-coordinate: min (m) = -2.000000e-01, max (m) = 2.100000e-01
z-coordinate: min (m) = 0.000000e+00, max (m) = 1.000000e-02
Volume statistics:
minimum volume (m3): 3.748429e-08
maximum volume (m3): 1.290568e-05
total volume (m3): 1.009166e-02
Face area statistics:
minimum face area (m2): 3.748429e-06
maximum face area (m2): 1.290568e-03
Checking mesh............................
Done.
Define fluids general solver settings#
fluent.setup.general.solver.type = "pressure-based"
fluent.solution.methods.high_order_term_relaxation.enable = True
Define the fluid and update the material properties#
viscosity = (FLUID_DENS * U_BAR * CYLINDER_DIA) / RE
fluent.setup.models.viscous.model = "laminar"
fluent.setup.materials.fluid["water"] = {
"density": {"option": "constant", "value": FLUID_DENS},
"viscosity": {"option": "constant", "value": viscosity},
}
fluent.setup.cell_zone_conditions.fluid["*fluid*"].general.material = "water"
fluent.setup.materials.print_state()
database :
database_type : fluent-database
fluid :
air :
name : air
chemical_formula :
density :
option : constant
value : 1.225
viscosity :
option : constant
value : 1.7894e-05
water :
name : water
chemical_formula :
density :
option : constant
value : 1000
viscosity :
option : constant
value : 1.0
solid :
aluminum :
name : aluminum
chemical_formula : al
density :
option : constant
value : 2719
Create the parabolic inlet profile as a named expression#
fluent.setup.named_expressions["u_bar"] = { # average velocity
"definition": f"{U_BAR} [m/s]"
}
fluent.setup.named_expressions["t_bar"] = {"definition": "1.0 [s]"}
fluent.setup.named_expressions["y_bar"] = {"definition": "1.0 [m]"}
fluent.setup.named_expressions["u_y"] = {
"definition": f"(6 * u_bar / ( ( 4.1 * {CYLINDER_DIA} ) ** 2 )) \
* ( y/y_bar + 0.2 ) * ( 0.21 - y/y_bar )"
}
Update the inlet field#
inlet_fluid = fluent.setup.boundary_conditions.velocity_inlet["inlet"]
inlet_fluid.momentum.initial_gauge_pressure.value = 0
inlet_fluid.momentum.velocity.value = "u_y"
fluent.setup.named_expressions.print_state()
t_bar :
name : t_bar
definition : 1.0 [s]
description :
parameterid :
parametername :
unit :
input_parameter : False
output_parameter : False
u_bar :
name : u_bar
definition : 1 [m/s]
description :
parameterid :
parametername :
unit :
input_parameter : False
output_parameter : False
u_y :
name : u_y
definition : (6 * u_bar / ( ( 4.1 * 0.1 ) ** 2 )) * ( y/y_bar + 0.2 ) * ( 0.21 - y/y_bar )
description :
parameterid :
parametername :
unit :
input_parameter : False
output_parameter : False
y_bar :
name : y_bar
definition : 1.0 [m]
description :
parameterid :
parametername :
unit :
input_parameter : False
output_parameter : False
Setup any relevant solution controls#
fluent.solution.methods.discretization_scheme = {
"mom": "second-order-upwind",
"pressure": "second-order",
}
Initialize the flow field & run a steady simulation#
First, a steady simulation is conducted to initialize the flow field with the parabolic inlet flow.
fluent.solution.initialization.hybrid_initialize()
fluent.solution.run_calculation.iterate(iter_count=500)
Initialize using the hybrid initialization method.
Checking case topology...
-This case has both inlets & outlets
-Pressure information is not available at the boundaries.
Case will be initialized with constant pressure
iter scalar-0
1 1.000000e+00
2 1.213050e-05
3 2.572431e-06
4 1.282160e-06
5 4.130318e-07
6 1.825524e-07
7 6.718438e-08
8 2.651539e-08
9 1.019930e-08
10 4.000471e-09
Hybrid initialization is done.
iter continuity x-velocity y-velocity z-velocity time/iter
1 1.0000e+00 1.2988e-02 5.4052e-03 0.0000e+00 0:00:21 499
2 6.0422e-01 9.2900e-03 3.0441e-03 0.0000e+00 0:00:22 498
3 4.6533e-01 7.3624e-03 1.8828e-03 0.0000e+00 0:00:22 497
4 3.7461e-01 6.1863e-03 1.2895e-03 0.0000e+00 0:00:22 496
5 3.1013e-01 5.3575e-03 9.6644e-04 0.0000e+00 0:00:21 495
6 2.7349e-01 4.6957e-03 7.5868e-04 6.8550e-14 0:00:21 494
7 2.6472e-01 4.1352e-03 6.2159e-04 3.9989e-14 0:00:21 493
8 2.4880e-01 3.6612e-03 5.2709e-04 2.5735e-14 0:00:21 492
9 2.2863e-01 3.2835e-03 4.5395e-04 1.8040e-14 0:00:21 491
10 2.0993e-01 2.9670e-03 3.9798e-04 1.4244e-14 0:00:22 490
11 1.9688e-01 2.6976e-03 3.5848e-04 1.1597e-14 0:00:22 489
iter continuity x-velocity y-velocity z-velocity time/iter
12 1.8175e-01 2.4688e-03 3.2553e-04 9.7888e-15 0:00:21 488
13 1.6791e-01 2.2698e-03 2.9865e-04 8.6386e-15 0:00:21 487
14 1.5669e-01 2.1003e-03 2.7747e-04 7.5366e-15 0:00:21 486
15 1.4811e-01 1.9591e-03 2.5991e-04 6.9611e-15 0:00:21 485
16 1.3957e-01 1.8384e-03 2.4618e-04 6.6191e-15 0:00:21 484
17 1.3280e-01 1.7359e-03 2.3505e-04 6.5294e-15 0:00:21 483
18 1.2520e-01 1.6469e-03 2.2550e-04 6.0518e-15 0:00:21 482
19 1.2116e-01 1.5643e-03 2.1738e-04 5.2223e-15 0:00:21 481
20 1.1603e-01 1.4905e-03 2.0910e-04 4.3215e-15 0:00:21 480
21 1.1417e-01 1.4232e-03 2.0288e-04 3.4610e-15 0:00:21 479
22 1.1095e-01 1.3627e-03 1.9792e-04 2.7499e-15 0:00:21 478
iter continuity x-velocity y-velocity z-velocity time/iter
23 1.0499e-01 1.3055e-03 1.9180e-04 2.4630e-15 0:00:21 477
24 9.9785e-02 1.2505e-03 1.8552e-04 2.2110e-15 0:00:21 476
25 9.6403e-02 1.1982e-03 1.8127e-04 1.6772e-15 0:00:21 475
26 9.1879e-02 1.1469e-03 1.7664e-04 1.2652e-15 0:00:21 474
27 8.7518e-02 1.1000e-03 1.7114e-04 1.2256e-15 0:00:21 473
28 8.5723e-02 1.0550e-03 1.6596e-04 1.2129e-15 0:00:21 472
29 8.3369e-02 1.0111e-03 1.6193e-04 9.8516e-16 0:00:29 471
30 7.9603e-02 9.7007e-04 1.5688e-04 8.1901e-16 0:00:27 470
31 7.5947e-02 9.3081e-04 1.5170e-04 7.5297e-16 0:00:25 469
32 7.1970e-02 8.9229e-04 1.4721e-04 7.1759e-16 0:00:53 468
33 6.7985e-02 8.5609e-04 1.4275e-04 6.8166e-16 0:00:46 467
iter continuity x-velocity y-velocity z-velocity time/iter
34 6.4003e-02 8.2155e-04 1.3704e-04 6.6138e-16 0:00:41 466
35 6.0994e-02 7.8842e-04 1.3197e-04 5.9408e-16 0:00:36 465
36 5.7711e-02 7.5643e-04 1.2724e-04 5.1519e-16 0:00:33 464
37 5.5130e-02 7.2551e-04 1.2289e-04 4.3624e-16 0:00:30 463
38 5.1997e-02 6.9534e-04 1.1839e-04 3.6017e-16 0:00:28 462
39 4.7873e-02 6.6618e-04 1.1301e-04 2.9290e-16 0:00:26 461
40 4.4954e-02 6.3833e-04 1.0829e-04 2.4966e-16 0:00:25 460
41 4.2367e-02 6.1138e-04 1.0362e-04 2.0739e-16 0:00:23 459
42 3.9912e-02 5.8557e-04 9.8739e-05 1.6815e-16 0:00:23 458
43 3.7625e-02 5.6107e-04 9.3923e-05 1.5285e-16 0:00:22 457
44 3.5052e-02 5.3745e-04 8.9587e-05 1.4216e-16 0:00:22 456
iter continuity x-velocity y-velocity z-velocity time/iter
45 3.2460e-02 5.1463e-04 8.5139e-05 1.2985e-16 0:00:22 455
46 3.0356e-02 4.9301e-04 8.0768e-05 1.2434e-16 0:00:21 454
47 2.8422e-02 4.7221e-04 7.6797e-05 1.2014e-16 0:00:21 453
48 2.6773e-02 4.5243e-04 7.2965e-05 1.1196e-16 0:00:21 452
49 2.5304e-02 4.3376e-04 6.9326e-05 1.0090e-16 0:00:21 451
50 2.4067e-02 4.1610e-04 6.5745e-05 8.7713e-17 0:00:20 450
51 2.2653e-02 3.9914e-04 6.2516e-05 7.3759e-17 0:00:21 449
52 2.1299e-02 3.8305e-04 5.9328e-05 6.0714e-17 0:00:21 448
53 2.0085e-02 3.6774e-04 5.6452e-05 5.2110e-17 0:00:21 447
54 1.8929e-02 3.5320e-04 5.3861e-05 4.8589e-17 0:00:20 446
55 1.7787e-02 3.3943e-04 5.1415e-05 4.7539e-17 0:00:20 445
iter continuity x-velocity y-velocity z-velocity time/iter
56 1.6691e-02 3.2636e-04 4.9004e-05 4.5934e-17 0:00:20 444
57 1.5670e-02 3.1389e-04 4.6870e-05 4.4163e-17 0:00:20 443
58 1.4790e-02 3.0214e-04 4.4692e-05 4.2252e-17 0:00:19 442
59 1.3872e-02 2.9089e-04 4.2674e-05 3.9374e-17 0:00:19 441
60 1.3103e-02 2.8015e-04 4.0804e-05 3.6262e-17 0:00:19 440
61 1.2101e-02 2.6986e-04 3.9199e-05 3.2635e-17 0:00:19 439
62 1.1389e-02 2.6028e-04 3.7633e-05 2.9172e-17 0:00:19 438
63 1.0649e-02 2.5119e-04 3.6171e-05 2.6318e-17 0:00:19 437
64 1.0136e-02 2.4256e-04 3.4865e-05 2.3399e-17 0:00:20 436
65 9.7014e-03 2.3436e-04 3.3554e-05 2.0301e-17 0:00:20 435
66 9.3621e-03 2.2654e-04 3.2344e-05 1.7432e-17 0:00:20 434
iter continuity x-velocity y-velocity z-velocity time/iter
67 8.9332e-03 2.1913e-04 3.1124e-05 1.5878e-17 0:00:19 433
68 8.7320e-03 2.1220e-04 2.9963e-05 1.5523e-17 0:00:19 432
69 8.4962e-03 2.0547e-04 2.8936e-05 1.4920e-17 0:00:19 431
70 8.2318e-03 1.9906e-04 2.7896e-05 1.4236e-17 0:00:19 430
71 7.9459e-03 1.9296e-04 2.6956e-05 1.3569e-17 0:00:19 429
72 7.6883e-03 1.8715e-04 2.6011e-05 1.2874e-17 0:00:19 428
73 7.3386e-03 1.8163e-04 2.5161e-05 1.2180e-17 0:00:19 427
74 7.0831e-03 1.7639e-04 2.4318e-05 1.1387e-17 0:00:19 426
75 6.8238e-03 1.7138e-04 2.3474e-05 1.0446e-17 0:00:19 425
76 6.6377e-03 1.6664e-04 2.2715e-05 9.5202e-18 0:00:19 424
77 6.4309e-03 1.6212e-04 2.1949e-05 8.6096e-18 0:00:19 423
iter continuity x-velocity y-velocity z-velocity time/iter
78 6.2116e-03 1.5783e-04 2.1209e-05 7.7720e-18 0:00:19 422
79 5.9921e-03 1.5372e-04 2.0516e-05 7.1087e-18 0:00:18 421
80 5.7797e-03 1.4974e-04 1.9798e-05 6.6990e-18 0:00:18 420
81 5.5621e-03 1.4589e-04 1.9161e-05 6.4146e-18 0:00:18 419
82 5.4070e-03 1.4221e-04 1.8527e-05 6.0928e-18 0:00:19 418
83 5.2226e-03 1.3861e-04 1.7895e-05 5.7269e-18 0:00:18 417
84 5.0626e-03 1.3512e-04 1.7327e-05 5.4528e-18 0:00:18 416
85 4.9197e-03 1.3167e-04 1.6716e-05 5.1340e-18 0:00:18 415
86 4.8011e-03 1.2834e-04 1.6162e-05 4.8662e-18 0:00:18 414
87 4.6879e-03 1.2504e-04 1.5608e-05 4.5552e-18 0:00:18 413
88 4.6217e-03 1.2182e-04 1.5064e-05 4.2674e-18 0:00:19 412
iter continuity x-velocity y-velocity z-velocity time/iter
89 4.5177e-03 1.1862e-04 1.4555e-05 4.0339e-18 0:00:18 411
90 4.3746e-03 1.1548e-04 1.4045e-05 3.7697e-18 0:00:18 410
91 4.2680e-03 1.1240e-04 1.3567e-05 3.5219e-18 0:00:18 409
92 4.1332e-03 1.0933e-04 1.3088e-05 3.3104e-18 0:00:18 408
93 4.0106e-03 1.0629e-04 1.2632e-05 3.2128e-18 0:00:18 407
94 3.8901e-03 1.0332e-04 1.2184e-05 3.0416e-18 0:00:18 406
95 3.7946e-03 1.0036e-04 1.1740e-05 2.9067e-18 0:00:18 405
96 3.6299e-03 9.7405e-05 1.1332e-05 2.9273e-18 0:00:18 404
97 3.5088e-03 9.4469e-05 1.0915e-05 2.7864e-18 0:00:18 403
98 3.4069e-03 9.1589e-05 1.0519e-05 2.6590e-18 0:00:18 402
99 3.3017e-03 8.8718e-05 1.0137e-05 2.5889e-18 0:00:18 401
iter continuity x-velocity y-velocity z-velocity time/iter
100 3.2234e-03 8.5874e-05 9.7524e-06 2.5039e-18 0:00:18 400
101 3.1318e-03 8.3059e-05 9.3978e-06 2.4294e-18 0:00:18 399
102 3.0388e-03 8.0270e-05 9.0454e-06 2.2807e-18 0:00:18 398
103 2.9645e-03 7.7498e-05 8.7086e-06 2.1629e-18 0:00:18 397
104 2.8871e-03 7.4772e-05 8.3906e-06 2.1431e-18 0:00:17 396
105 2.7557e-03 7.2058e-05 8.0724e-06 2.0565e-18 0:00:17 395
106 2.7106e-03 6.9384e-05 7.7753e-06 2.0258e-18 0:00:17 394
107 2.5775e-03 6.6744e-05 7.4853e-06 1.9239e-18 0:00:17 393
108 2.5162e-03 6.4114e-05 7.2042e-06 1.8495e-18 0:00:17 392
109 2.3944e-03 6.1554e-05 6.9437e-06 1.7764e-18 0:00:16 391
110 2.3326e-03 5.8997e-05 6.6953e-06 1.7524e-18 0:00:16 390
iter continuity x-velocity y-velocity z-velocity time/iter
111 2.2450e-03 5.6505e-05 6.4518e-06 1.7314e-18 0:00:16 389
112 2.1744e-03 5.4041e-05 6.2257e-06 1.6777e-18 0:00:16 388
113 2.0907e-03 5.1618e-05 6.0124e-06 1.6380e-18 0:00:16 387
114 2.0469e-03 4.9259e-05 5.7993e-06 1.5357e-18 0:00:16 386
115 1.9372e-03 4.6928e-05 5.6059e-06 1.6163e-18 0:00:16 385
116 1.8911e-03 4.4667e-05 5.4178e-06 1.5830e-18 0:00:16 384
117 1.7974e-03 4.2448e-05 5.2287e-06 1.5211e-18 0:00:16 383
118 1.7491e-03 4.0285e-05 5.0466e-06 1.5344e-18 0:00:16 382
119 1.7080e-03 3.8189e-05 4.8812e-06 1.5602e-18 0:00:16 381
120 1.6350e-03 3.6137e-05 4.7189e-06 1.5397e-18 0:00:15 380
121 1.5985e-03 3.4159e-05 4.5624e-06 1.4721e-18 0:00:16 379
iter continuity x-velocity y-velocity z-velocity time/iter
122 1.5597e-03 3.2238e-05 4.4162e-06 1.4710e-18 0:00:16 378
123 1.5048e-03 3.0386e-05 4.2735e-06 1.4020e-18 0:00:16 377
124 1.4703e-03 2.8600e-05 4.1350e-06 1.4129e-18 0:00:16 376
125 1.4261e-03 2.6873e-05 4.0005e-06 1.3829e-18 0:00:16 375
126 1.3661e-03 2.5230e-05 3.8620e-06 1.3825e-18 0:00:16 374
127 1.3241e-03 2.3648e-05 3.7216e-06 1.4373e-18 0:00:16 373
128 1.2704e-03 2.2131e-05 3.5806e-06 1.3735e-18 0:00:16 372
129 1.2094e-03 2.0697e-05 3.4406e-06 1.2980e-18 0:00:16 371
130 1.1573e-03 1.9326e-05 3.3062e-06 1.3048e-18 0:00:16 370
131 1.0769e-03 1.8023e-05 3.1763e-06 1.3072e-18 0:00:16 369
132 1.0543e-03 1.6800e-05 3.0454e-06 1.3447e-18 0:00:16 368
iter continuity x-velocity y-velocity z-velocity time/iter
133 1.0377e-03 1.5639e-05 2.9168e-06 1.2842e-18 0:00:16 367
134 9.9983e-04 1.4547e-05 2.7861e-06 1.3548e-18 0:00:16 366
! 134 solution is converged
Switch to transient mode and prepare for coupling#
fluent.setup.general.solver.time = "unsteady-1st-order"
Pressure-Velocity Coupling scheme is set to SIMPLE
Define dynamic meshing#
Define dynamic meshing for deforming symmetry planes. Currently, dynamic_mesh is not exposed to the fluent root session directly. We need to use the tui framework to create dynamic zones.
fluent.tui.define.dynamic_mesh.dynamic_mesh("yes", "no", "no", "no", "no")
fluent.tui.define.dynamic_mesh.zones.create("fsi", "system-coupling")
fluent.tui.define.dynamic_mesh.zones.create(
"symmetry_bot",
"deforming",
"plane",
"0.",
"0.",
"0.00",
"0",
"0",
"1",
"no",
"yes",
"yes",
"yes",
"no",
"yes",
"no",
"yes",
)
fluent.tui.define.dynamic_mesh.zones.create(
"symmetry_top",
"deforming",
"plane",
"0.",
"0.",
"0.01",
"0",
"0",
"1",
"no",
"yes",
"yes",
"yes",
"no",
"yes",
"no",
"yes",
)
Results and output controls#
Define number of sub-steps fluent iterates for each coupling step. Maximum integration time and total steps are controlled by system coupling.
fluent.solution.run_calculation.transient_controls.max_iter_per_time_step = 20
fluent.file.auto_save.save_data_file_every.frequency_type = "time-step"
fluent.file.auto_save.data_frequency = 10
fluent.file.auto_save.root_name = "turek_hron_fluid_resolved"
Set up the coupled analysis#
System Coupling setup involves adding the structural and fluid participants, adding coupled interfaces and data transfers, and setting other coupled analysis properties.
Add participants#
Add participants by passing session handles to System Coupling.
Writing System Coupling file...done.
Writing System Coupling file...done.
Writing System Coupling file...done.
Writing System Coupling file...done.
Setup the interface and data transfers#
Add a coupling interface and data transfers.
interface_name = syc.setup.add_interface(
side_one_participant=fluid,
side_one_regions=["fsi"],
side_two_participant=solid,
side_two_regions=["FSIN_1"],
)
# set up 2-way FSI coupling - add force & displacement data transfers
data_transfer = syc.setup.add_fsi_data_transfers(interface=interface_name)
force_transfer = syc.setup.coupling_interface[interface_name].data_transfer["FORC"]
force_transfer.relaxation_factor = 0.5
Time step size, end time, output controls
syc.setup.solution_control.time_step_size = "0.01 [s]" # time step is 0.01 [s]
# To generate similar results shown in this example documentation, increase an end_time
# parameter to 15 [s].
syc.setup.solution_control.end_time = "0.1 [s]" # end time
syc.setup.output_control.option = "StepInterval"
syc.setup.output_control.output_frequency = 250
# print(syc.setup.get_setup_summary())
Solve the coupled system#
syc.solution.solve()
+=============================================================================+
| Coupling Participants (2) |
+=============================================================================+
| FLUENT-1 |
+-----------------------------------------------------------------------------+
| Internal Name : FLUENT-1 |
| Participant Type : FLUENT |
| Participant Display Name : FLUENT-1 |
| Dimension : 3D |
| Input Parameters : [] |
| Output Parameters : [] |
| Participant Analysis Type : Transient |
| Use New APIs : True |
| Restarts Supported : True |
| Variables (3) |
| Variable : Lorentz_Force |
| Internal Name : lorentz-force |
| Quantity Type : Force |
| Participant Display Name : Lorentz Force |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : True |
| |
| Variable : displacement |
| Internal Name : displacement |
| Quantity Type : Incremental Displacement |
| Participant Display Name : displacement |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : False |
| |
| Variable : force |
| Internal Name : force |
| Quantity Type : Force |
| Participant Display Name : force |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : True |
| Regions (6) |
| Region : cylinder |
| Internal Name : cylinder |
| Topology : Surface |
| Input Variables : [] |
| Output Variables : [force] |
| Region Discretization Type : Mesh Region |
| |
| Region : d8b0bb73-1d68-4795-9b6f-6b2467eb5a7b_fluid |
| Internal Name : d8b0bb73-1d68-4795-9b6f-6b2467eb5a7b_fluid |
| Topology : Volume |
| Input Variables : [] |
| Output Variables : [] |
| Region Discretization Type : Mesh Region |
| |
| Region : fsi |
| Internal Name : fsi |
| Topology : Surface |
| Input Variables : [displacement] |
| Output Variables : [force] |
| Region Discretization Type : Mesh Region |
| |
| Region : inlet |
| Internal Name : inlet |
| Topology : Surface |
| Input Variables : [] |
| Output Variables : [] |
| Region Discretization Type : Mesh Region |
| |
| Region : outlet |
| Internal Name : outlet |
| Topology : Surface |
| Input Variables : [] |
| Output Variables : [] |
| Region Discretization Type : Mesh Region |
| |
| Region : wall |
| Internal Name : wall |
| Topology : Surface |
| Input Variables : [] |
| Output Variables : [force] |
| Region Discretization Type : Mesh Region |
| Properties |
| Accepts New Inputs : False |
| Time Integration : Implicit |
| Update Control |
| Option : ProgramControlled |
| Execution Control |
| Option : ExternallyManaged |
+-----------------------------------------------------------------------------+
| MAPDL-2 |
+-----------------------------------------------------------------------------+
| Internal Name : MAPDL-2 |
| Participant Type : MAPDL |
| Participant Display Name : MAPDL-2 |
| Dimension : 3D |
| Input Parameters : [] |
| Output Parameters : [] |
| Participant Analysis Type : Transient |
| Restarts Supported : True |
| Variables (3) |
| Variable : Force |
| Internal Name : FORC |
| Quantity Type : Force |
| Location : Node |
| Participant Display Name : Force |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : True |
| |
| Variable : Force_Density |
| Internal Name : FDNS |
| Quantity Type : Force |
| Location : Element |
| Participant Display Name : Force Density |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : False |
| |
| Variable : Incremental_Displacement |
| Internal Name : INCD |
| Quantity Type : Incremental Displacement |
| Location : Node |
| Participant Display Name : Incremental Displacement |
| Data Type : Real |
| Tensor Type : Vector |
| Is Extensive : False |
| Regions (3) |
| Region : System Coupling (Surface) Region 0 |
| Internal Name : FSIN_1 |
| Topology : Surface |
| Input Variables : [FORC, FDNS] |
| Output Variables : [INCD] |
| Region Discretization Type : Mesh Region |
| |
| Region : System Coupling (Surface) Region 1 |
| Internal Name : _FIXEDSU |
| Topology : Surface |
| Input Variables : [FORC, FDNS] |
| Output Variables : [INCD] |
| Region Discretization Type : Mesh Region |
| |
| Region : System Coupling (Surface) Region 2 |
| Internal Name : _FRICSUZ |
| Topology : Surface |
| Input Variables : [FORC, FDNS] |
| Output Variables : [INCD] |
| Region Discretization Type : Mesh Region |
| Properties |
| Accepts New Inputs : False |
| Time Integration : Implicit |
| Update Control |
| Option : ProgramControlled |
| Execution Control |
| Option : ExternallyManaged |
+=============================================================================+
| Analysis Control |
+=============================================================================+
| Analysis Type : Transient |
| Optimize If One Way : True |
| Allow Simultaneous Update : False |
| Partitioning Algorithm : SharedAllocateMachines |
| Global Stabilization |
| Option : None |
+=============================================================================+
| Coupling Interfaces (1) |
+=============================================================================+
| Interface-1 |
+-----------------------------------------------------------------------------+
| Internal Name : Interface-1 |
| Side |
| Side: One |
| Coupling Participant : FLUENT-1 |
| Region List : [fsi] |
| Reference Frame : GlobalReferenceFrame |
| Instancing : None |
| Side: Two |
| Coupling Participant : MAPDL-2 |
| Region List : [FSIN_1] |
| Reference Frame : GlobalReferenceFrame |
| Instancing : None |
| Data Transfers (2) |
| DataTransfer : Force |
| Internal Name : FORC |
| Suppress : False |
| Target Side : Two |
| Option : UsingVariable |
| Source Variable : force |
| Target Variable : FORC |
| Ramping Option : None |
| Relaxation Factor : 5.00e-01 |
| Convergence Target : 1.00e-02 |
| Mapping Type : Conservative |
| DataTransfer : displacement |
| Internal Name : displacement |
| Suppress : False |
| Target Side : One |
| Option : UsingVariable |
| Source Variable : INCD |
| Target Variable : displacement |
| Ramping Option : None |
| Relaxation Factor : 1.00e+00 |
| Convergence Target : 1.00e-02 |
| Mapping Type : ProfilePreserving |
| Unmapped Value Option : ProgramControlled |
| Mapping Control |
| Stop If Poor Intersection : True |
| Poor Intersection Threshold : 5.00e-01 |
| Face Alignment : ProgramControlled |
| Absolute Gap Tolerance : 0.0 [m] |
| Relative Gap Tolerance : 1.00e+00 |
+=============================================================================+
| Solution Control |
+=============================================================================+
| Duration Option : EndTime |
| End Time : 0.1 [s] |
| Time Step Size : 0.01 [s] |
| Minimum Iterations : 1 |
| Maximum Iterations : 5 |
| Use IP Address When Possible : True |
+=============================================================================+
| Output Control |
+=============================================================================+
| Option : StepInterval |
| Generate CSV Chart Output : False |
| Write Initial Snapshot : True |
| Output Frequency : 250 |
| Results |
| Option : ProgramControlled |
| Include Instances : ProgramControlled |
| Type |
| Option : EnsightGold |
+=============================================================================+
+-----------------------------------------------------------------------------+
| Warnings were found during data model validation. |
+-----------------------------------------------------------------------------+
| Warning: Participant MAPDL-2 (CouplingParticipant:MAPDL-2) has the |
| ExecutionControl 'Option' set to 'ExternallyManaged'. System Coupling |
| will not control the startup/shutdown behavior of this participant. |
| Warning: Participant FLUENT-1 (CouplingParticipant:FLUENT-1) has the |
| ExecutionControl 'Option' set to 'ExternallyManaged'. System Coupling |
| will not control the startup/shutdown behavior of this participant. |
| Warning: Unused input variables ['Force_Density'] (FDNS) on region FSIN_1 |
| for MAPDL-2 (CouplingParticipant:MAPDL-2). |
| Warning: Unused input variables ['Force', 'Force_Density'] (FORC, FDNS) on |
| region _FRICSUZ for MAPDL-2 (CouplingParticipant:MAPDL-2). |
| Warning: Unused input variables ['Force', 'Force_Density'] (FORC, FDNS) on |
| region _FIXEDSU for MAPDL-2 (CouplingParticipant:MAPDL-2). |
+-----------------------------------------------------------------------------+
+=============================================================================+
| Execution Information |
+=============================================================================+
| |
| System Coupling |
| Command Line Arguments: |
| -m cosimgui --grpcport=0.0.0.0:55131 |
| Working Directory: |
| /working |
| |
| FLUENT-1 |
| Not started by System Coupling |
| |
| MAPDL-2 |
| Not started by System Coupling |
| |
+=============================================================================+
Successfully connected to System Coupling
Awaiting connections from coupling participants... done.
+=============================================================================+
| Build Information |
+-----------------------------------------------------------------------------+
| System Coupling |
| 2024 R2: Build ID: 48974af Build Date: 2024-05-13T13:51 |
| FLUENT-1 |
| ANSYS Fluent 24.0 2.0 0.0 Build Time: May 13 2024 11:01:41 EDT Build Id: |
| 10192 |
| MAPDL-2 |
| Mechanical APDL Release Build 24.2 UP20240603 |
| DISTRIBUTED LINUX x64 Version |
+=============================================================================+
===============================================================================
+=============================================================================+
| |
| Analysis Initialization |
| |
+=============================================================================+
===============================================================================
+-----------------------------------------------------------------------------+
| MESH STATISTICS |
+-----------------------------------------------------------------------------+
| Participant: FLUENT-1 |
| Number of face regions 1 |
| Number of faces 84 |
| Quadrilateral 84 |
| Area (m2) 8.200e-03 |
| Bounding Box (m) |
| Minimum [ 5.000e-02 -1.000e-02 0.000e+00] |
| Maximum [ 4.500e-01 1.000e-02 1.000e-02] |
| |
| Participant: MAPDL-2 |
| Number of face regions 1 |
| Number of faces 104 |
| Quadrilateral8 104 |
| Area (m2) 8.220e-03 |
| Bounding Box (m) |
| Minimum [ 4.899e-02 -1.000e-02 0.000e+00] |
| Maximum [ 4.500e-01 1.000e-02 1.000e-02] |
| |
| Total |
| Number of cells 0 |
| Number of faces 188 |
| Number of nodes 693 |
+-----------------------------------------------------------------------------+
+-----------------------------------------------------------------------------+
| MAPPING SUMMARY |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| Interface-1 | |
| Force | |
| Mapped Area [%] | 100 100 |
| Mapped Elements [%] | 100 100 |
| Mapped Nodes [%] | 100 100 |
| displacement | |
| Mapped Area [%] | 100 100 |
| Mapped Elements [%] | 100 100 |
| Mapped Nodes [%] | 100 100 |
+-----------------------------------------------------------------------------+
+-----------------------------------------------------------------------------+
| Transfer Diagnostics |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | |
| Sum x | -3.78E-14 -3.78E-14 |
| Sum y | -4.42E-29 -5.61E-28 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | |
| Weighted Average x | 0.00E+00 0.00E+00 |
| Weighted Average y | 0.00E+00 0.00E+00 |
| Weighted Average z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
===============================================================================
+=============================================================================+
| |
| Coupled Solution |
| |
+=============================================================================+
===============================================================================
+=============================================================================+
| COUPLING STEP = 1 SIMULATION TIME = 1.00000E-02 [s] |
+=============================================================================+
NOTE: System Coupling analysis settings will override Fluent calculation settings
COUPLING STEP = 1 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.00E+00 1.00E+00 |
| Sum x | -3.78E-14 -3.78E-14 |
| Sum y | -4.42E-29 -5.61E-28 |
| Sum z | 0.00E+00 0.00E+00 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.00E+00 1.00E+00 |
| Weighted Average x | -5.47E-19 -5.47E-19 |
| Weighted Average y | 1.77E-31 1.78E-31 |
| Weighted Average z | 3.80E-34 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
1 1.0000e+00 4.9048e-03 1.4517e-04 0.0000e+00 0:00:01 19
2 1.0000e+00 9.1003e-03 7.8288e-03 0.0000e+00 0:00:01 18
3 4.6252e-01 3.9585e-03 2.9783e-03 0.0000e+00 0:00:01 17
4 2.5246e-01 2.2003e-03 1.2802e-03 0.0000e+00 0:00:01 16
5 1.6992e-01 1.4894e-03 6.2937e-04 0.0000e+00 0:00:01 15
6 1.2482e-01 1.0146e-03 3.6791e-04 8.2650e-15 0:00:01 14
7 9.3311e-02 7.5039e-04 2.5012e-04 6.9633e-15 0:00:00 13
8 7.2581e-02 5.5055e-04 1.8278e-04 5.1688e-15 0:00:00 12
9 5.7295e-02 4.1527e-04 1.3735e-04 3.5978e-15 0:00:00 11
10 4.4910e-02 3.2724e-04 1.0546e-04 2.3513e-15 0:00:00 10
11 3.5425e-02 2.6834e-04 7.8464e-05 1.7795e-15 0:00:00 9
iter continuity x-velocity y-velocity z-velocity time/iter
12 2.8258e-02 2.3050e-04 5.9950e-05 1.3607e-15 0:00:00 8
13 2.2452e-02 2.0420e-04 4.5911e-05 1.0958e-15 0:00:00 7
14 1.8038e-02 1.8550e-04 3.5619e-05 8.4633e-16 0:00:00 6
15 1.4437e-02 1.7051e-04 2.7875e-05 6.6764e-16 0:00:00 5
16 1.1594e-02 1.6217e-04 2.2027e-05 5.3094e-16 0:00:00 4
17 9.3596e-03 1.5499e-04 1.7488e-05 3.9742e-16 0:00:00 3
18 7.5035e-03 1.5092e-04 1.3952e-05 3.0056e-16 0:00:00 2
19 6.0272e-03 1.4779e-04 1.1137e-05 2.2384e-16 0:00:00 1
20 4.8696e-03 1.4539e-04 8.9986e-06 1.6958e-16 0:00:00 0
Flow time = 0.01s, time step = 1
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Not yet converged |
COUPLING STEP = 1 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.04E+00 9.39E-01 |
| Sum x | 2.45E+00 1.22E+00 |
| Sum y | -2.56E-01 -1.28E-01 |
| Sum z | -3.66E-16 -1.83E-16 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.55E-01 7.39E-01 |
| Weighted Average x | 4.82E-05 4.83E-05 |
| Weighted Average y | -4.49E-06 -4.50E-06 |
| Weighted Average z | 1.50E-21 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
20 4.8696e-03 1.4539e-04 8.9986e-06 1.6958e-16 0:00:01 20
Updating mesh at iteration... done.
21 7.4702e-03 1.5155e-04 9.5654e-06 5.8720e-13 0:00:04 19
22 7.2550e-03 2.2751e-04 2.1755e-05 5.4446e-13 0:00:03 18
23 4.1580e-03 1.8510e-04 1.0651e-05 4.5307e-13 0:00:02 17
24 2.9373e-03 1.6373e-04 7.1331e-06 3.6596e-13 0:00:02 16
25 2.2644e-03 1.5234e-04 5.0978e-06 2.9019e-13 0:00:02 15
26 1.7612e-03 1.4700e-04 3.6852e-06 2.2776e-13 0:00:01 14
27 1.3682e-03 1.4364e-04 2.7631e-06 1.7847e-13 0:00:01 13
28 1.0886e-03 1.4122e-04 2.1766e-06 1.3932e-13 0:00:01 12
29 8.9400e-04 1.3961e-04 1.7247e-06 1.0840e-13 0:00:01 11
! 29 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.01s, time step = 1
COUPLING STEP = 1 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 6.24E-02 4.40E-01 |
| Sum x | 2.45E+00 1.84E+00 |
| Sum y | -1.40E-01 -1.34E-01 |
| Sum z | 5.55E-11 2.78E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 2.49E-01 2.44E-01 |
| Weighted Average x | 7.20E-05 7.22E-05 |
| Weighted Average y | -4.69E-06 -4.70E-06 |
| Weighted Average z | 3.11E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
29 8.9400e-04 1.3961e-04 1.7247e-06 1.0840e-13 0:00:01 20
! 29 solution is converged
Updating mesh at iteration... done.
30 2.4894e-03 1.4234e-04 2.3768e-06 4.2897e-13 0:00:04 19
31 2.5226e-03 1.7476e-04 8.1508e-06 3.7695e-13 0:00:03 18
32 1.1808e-03 1.5620e-04 2.7835e-06 3.0641e-13 0:00:03 17
33 7.3031e-04 1.4660e-04 1.7947e-06 2.4375e-13 0:00:02 16
! 33 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.01s, time step = 1
COUPLING STEP = 1 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.65E-02 2.02E-01 |
| Sum x | 2.45E+00 2.15E+00 |
| Sum y | -1.38E-01 -1.36E-01 |
| Sum z | 7.07E-11 4.92E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.06E-01 1.04E-01 |
| Weighted Average x | 8.38E-05 8.40E-05 |
| Weighted Average y | -4.75E-06 -4.76E-06 |
| Weighted Average z | 5.51E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
33 7.3031e-04 1.4660e-04 1.7947e-06 2.4375e-13 0:00:03 20
Updating mesh at iteration... done.
34 1.2604e-03 1.4459e-04 1.6260e-06 3.5553e-13 0:00:05 19
35 1.2512e-03 1.5537e-04 3.8135e-06 2.9843e-13 0:00:04 18
36 5.9332e-04 1.4625e-04 1.3552e-06 2.3842e-13 0:00:03 17
! 36 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.01s, time step = 1
COUPLING STEP = 1 COUPLING ITERATION = 5
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 5 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.16E-02 9.21E-02 |
| Sum x | 2.45E+00 2.30E+00 |
| Sum y | -1.37E-01 -1.36E-01 |
| Sum z | 7.40E-11 6.16E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 4.89E-02 4.79E-02 |
| Weighted Average x | 8.97E-05 8.99E-05 |
| Weighted Average y | -4.77E-06 -4.78E-06 |
| Weighted Average z | 6.90E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
36 5.9332e-04 1.4625e-04 1.3552e-06 2.3842e-13 0:00:04 20
Updating mesh at iteration... done.
37 6.7688e-04 1.4295e-04 1.1142e-06 2.7047e-13 0:00:06 19
! 37 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 2 SIMULATION TIME = 2.00000E-02 [s] |
+=============================================================================+
Flow time = 0.01s, time step = 1
COUPLING STEP = 2 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 5.28E-03 4.18E-02 |
| Sum x | 2.45E+00 2.38E+00 |
| Sum y | -1.36E-01 -1.36E-01 |
| Sum z | 7.25E-11 6.70E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.07E-01 7.87E-01 |
| Weighted Average x | 2.97E-04 2.98E-04 |
| Weighted Average y | -1.69E-05 -1.69E-05 |
| Weighted Average z | 7.35E-19 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
37 6.7688e-04 1.4295e-04 1.1142e-06 2.7047e-13 0:00:06 20
38 4.8132e-01 1.1981e-02 7.8543e-03 5.3051e-12 0:00:05 19
39 3.6458e-01 5.5233e-03 3.6745e-03 6.1884e-12 0:00:04 18
40 2.6527e-01 2.8371e-03 1.8738e-03 4.7735e-12 0:00:03 17
41 1.8315e-01 1.4565e-03 9.9338e-04 3.3054e-12 0:00:02 16
42 1.3035e-01 7.9492e-04 5.6664e-04 2.3912e-12 0:00:02 15
43 9.4627e-02 5.2744e-04 3.4442e-04 1.6477e-12 0:00:02 14
44 6.9776e-02 3.9874e-04 2.2392e-04 1.2218e-12 0:00:01 13
45 5.0605e-02 2.8844e-04 1.4592e-04 8.2784e-13 0:00:01 12
46 3.7687e-02 2.1548e-04 1.0004e-04 6.1974e-13 0:00:01 11
47 2.8419e-02 1.6385e-04 7.2671e-05 4.7917e-13 0:00:01 10
iter continuity x-velocity y-velocity z-velocity time/iter
48 2.1766e-02 1.2880e-04 5.6652e-05 3.8111e-13 0:00:01 9
49 1.6979e-02 1.0100e-04 4.5374e-05 2.9797e-13 0:00:00 8
50 1.3418e-02 8.0203e-05 3.6006e-05 2.2728e-13 0:00:00 7
51 1.0863e-02 6.2262e-05 2.9034e-05 1.8079e-13 0:00:00 6
52 8.8196e-03 5.0599e-05 2.2541e-05 1.3832e-13 0:00:00 5
53 7.2939e-03 4.0355e-05 1.7810e-05 1.0822e-13 0:00:00 4
54 6.1277e-03 3.3014e-05 1.4124e-05 8.3066e-14 0:00:00 3
55 5.1085e-03 2.7609e-05 1.1186e-05 6.3665e-14 0:00:00 2
56 4.2543e-03 2.3512e-05 8.9437e-06 4.9244e-14 0:00:00 1
57 3.5571e-03 2.0557e-05 7.2210e-06 3.8272e-14 0:00:00 0
Flow time = 0.02s, time step = 2
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Not yet converged |
COUPLING STEP = 2 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 5.60E-01 4.64E-01 |
| Sum x | 1.21E+00 1.79E+00 |
| Sum y | 3.05E-01 8.44E-02 |
| Sum z | 2.07E-11 4.39E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.29E-02 8.25E-02 |
| Weighted Average x | 2.73E-04 2.73E-04 |
| Weighted Average y | -9.12E-06 -9.13E-06 |
| Weighted Average z | -2.94E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
57 3.5571e-03 2.0557e-05 7.2210e-06 3.8272e-14 0:00:01 20
Updating mesh at iteration... done.
58 5.8264e-03 2.2146e-05 8.0823e-06 3.8972e-13 0:00:04 19
59 5.4443e-03 3.9460e-05 2.0416e-05 3.3194e-13 0:00:03 18
60 3.5062e-03 2.8662e-05 1.2697e-05 2.2464e-13 0:00:02 17
61 2.3951e-03 2.1309e-05 7.6976e-06 1.6143e-13 0:00:02 16
62 1.7634e-03 1.7150e-05 4.9826e-06 1.2042e-13 0:00:02 15
63 1.3401e-03 1.5003e-05 3.4340e-06 9.1008e-14 0:00:01 14
64 1.0632e-03 1.3278e-05 2.4970e-06 6.9281e-14 0:00:01 13
65 8.4554e-04 1.2333e-05 1.9130e-06 5.3086e-14 0:00:01 12
! 65 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.02s, time step = 2
COUPLING STEP = 2 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.40E-02 2.25E-01 |
| Sum x | 1.21E+00 1.50E+00 |
| Sum y | 9.72E-02 9.08E-02 |
| Sum z | -1.57E-11 1.41E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 3.62E-02 3.60E-02 |
| Weighted Average x | 2.62E-04 2.63E-04 |
| Weighted Average y | -8.92E-06 -8.93E-06 |
| Weighted Average z | -6.43E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
65 8.4554e-04 1.2333e-05 1.9130e-06 5.3086e-14 0:00:01 20
Updating mesh at iteration... done.
66 1.4606e-03 1.3225e-05 1.7748e-06 1.5499e-13 0:00:04 19
67 1.3137e-03 1.6736e-05 3.7148e-06 1.2775e-13 0:00:03 18
68 7.2088e-04 1.2941e-05 1.5864e-06 8.9208e-14 0:00:03 17
! 68 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.02s, time step = 2
COUPLING STEP = 2 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 4.46E-03 1.09E-01 |
| Sum x | 1.22E+00 1.36E+00 |
| Sum y | 9.60E-02 9.34E-02 |
| Sum z | -2.15E-11 -3.73E-12 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.84E-02 1.83E-02 |
| Weighted Average x | 2.57E-04 2.57E-04 |
| Weighted Average y | -8.82E-06 -8.84E-06 |
| Weighted Average z | -8.30E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
68 7.2088e-04 1.2941e-05 1.5864e-06 8.9208e-14 0:00:03 20
Updating mesh at iteration... done.
69 7.9593e-04 1.2470e-05 1.3917e-06 1.2710e-13 0:00:06 19
! 69 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.02s, time step = 2
COUPLING STEP = 2 COUPLING ITERATION = 5
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 5 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.79E-03 5.24E-02 |
| Sum x | 1.22E+00 1.29E+00 |
| Sum y | 9.31E-02 9.32E-02 |
| Sum z | -2.12E-11 -1.25E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.08E-03 9.03E-03 |
| Weighted Average x | 2.54E-04 2.55E-04 |
| Weighted Average y | -8.83E-06 -8.84E-06 |
| Weighted Average z | -9.18E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
69 7.9593e-04 1.2470e-05 1.3917e-06 1.2710e-13 0:00:06 20
Updating mesh at iteration... done.
70 6.0794e-04 1.2971e-05 1.7309e-06 1.2982e-13 0:00:08 19
! 70 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 3 SIMULATION TIME = 3.00000E-02 [s] |
+=============================================================================+
Flow time = 0.02s, time step = 2
COUPLING STEP = 3 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 5.10E-04 2.58E-02 |
| Sum x | 1.22E+00 1.26E+00 |
| Sum y | 9.37E-02 9.35E-02 |
| Sum z | -2.32E-11 -1.78E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.45E-01 8.23E-01 |
| Weighted Average x | 3.51E-04 3.51E-04 |
| Weighted Average y | -2.82E-06 -2.82E-06 |
| Weighted Average z | -7.12E-19 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
70 6.0794e-04 1.2971e-05 1.7309e-06 1.2982e-13 0:00:08 20
71 7.9825e-02 4.4370e-03 9.3662e-04 4.2889e-12 0:00:06 19
72 6.4467e-02 2.0722e-03 5.1783e-04 2.8394e-12 0:00:05 18
73 5.1163e-02 1.0555e-03 3.0670e-04 2.0085e-12 0:00:04 17
74 3.8758e-02 5.7604e-04 1.9346e-04 1.4069e-12 0:00:03 16
75 2.9151e-02 3.5626e-04 1.3103e-04 1.0513e-12 0:00:02 15
76 2.2221e-02 2.3073e-04 9.4020e-05 7.8291e-13 0:00:02 14
77 1.7175e-02 1.5645e-04 6.9948e-05 5.6678e-13 0:00:01 13
78 1.3311e-02 1.1099e-04 5.3073e-05 4.2838e-13 0:00:01 12
79 1.0339e-02 8.0285e-05 4.1048e-05 3.1514e-13 0:00:01 11
80 8.1394e-03 6.0871e-05 3.2163e-05 2.4489e-13 0:00:01 10
iter continuity x-velocity y-velocity z-velocity time/iter
81 6.4593e-03 4.9099e-05 2.5384e-05 1.8607e-13 0:00:01 9
82 5.1668e-03 3.7234e-05 1.9966e-05 1.3741e-13 0:00:00 8
83 4.0830e-03 3.1796e-05 1.6015e-05 1.0767e-13 0:00:00 7
84 3.2997e-03 2.5837e-05 1.2779e-05 8.1508e-14 0:00:00 6
85 2.6709e-03 2.2091e-05 1.0289e-05 6.2836e-14 0:00:00 5
86 2.1721e-03 1.9021e-05 8.3148e-06 4.8450e-14 0:00:00 4
87 1.7704e-03 1.6532e-05 6.7451e-06 3.7370e-14 0:00:00 3
88 1.4529e-03 1.4623e-05 5.4916e-06 2.8913e-14 0:00:00 2
89 1.2012e-03 1.3105e-05 4.4786e-06 2.2421e-14 0:00:00 1
90 9.9570e-04 1.1899e-05 3.6563e-06 1.7435e-14 0:00:00 0
! 90 solution is converged
Flow time = 0.03s, time step = 3
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
COUPLING STEP = 3 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 4.72E-01 4.33E-01 |
| Sum x | 9.07E-01 1.08E+00 |
| Sum y | -1.64E-01 -3.51E-02 |
| Sum z | -2.32E-10 -1.25E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 2.60E-02 2.55E-02 |
| Weighted Average x | 3.41E-04 3.42E-04 |
| Weighted Average y | -7.35E-06 -7.36E-06 |
| Weighted Average z | -1.38E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
90 9.9570e-04 1.1899e-05 3.6563e-06 1.7435e-14 0:00:02 20
Updating mesh at iteration... done.
91 2.0529e-03 1.2546e-05 4.2589e-06 3.5061e-14 0:00:04 19
92 2.2842e-03 3.3049e-05 1.1663e-05 9.7476e-14 0:00:04 18
93 1.4471e-03 2.3315e-05 7.5181e-06 5.2694e-14 0:00:03 17
94 9.5847e-04 1.7087e-05 4.4687e-06 3.1098e-14 0:00:02 16
! 94 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.03s, time step = 3
COUPLING STEP = 3 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.58E-02 1.87E-01 |
| Sum x | 9.05E-01 9.93E-01 |
| Sum y | -3.27E-02 -3.39E-02 |
| Sum z | -2.36E-10 -1.81E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 8.37E-03 8.25E-03 |
| Weighted Average x | 3.38E-04 3.38E-04 |
| Weighted Average y | -7.30E-06 -7.31E-06 |
| Weighted Average z | -1.91E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
94 9.5847e-04 1.7087e-05 4.4687e-06 3.1098e-14 0:00:03 20
Updating mesh at iteration... done.
95 7.9779e-04 1.3567e-05 2.8743e-06 5.2829e-14 0:00:05 19
! 95 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.03s, time step = 3
COUPLING STEP = 3 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.00E-02 8.37E-02 |
| Sum x | 9.05E-01 9.49E-01 |
| Sum y | -4.06E-02 -3.73E-02 |
| Sum z | -2.35E-10 -2.08E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 4.15E-03 4.09E-03 |
| Weighted Average x | 3.36E-04 3.37E-04 |
| Weighted Average y | -7.42E-06 -7.43E-06 |
| Weighted Average z | -2.19E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
95 7.9779e-04 1.3567e-05 2.8743e-06 5.2829e-14 0:00:06 20
Updating mesh at iteration... done.
96 6.4359e-04 1.6986e-05 2.5248e-06 7.1611e-14 0:00:07 19
! 96 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.03s, time step = 3
COUPLING STEP = 3 COUPLING ITERATION = 5
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 5 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 5.08E-03 3.73E-02 |
| Sum x | 9.05E-01 9.27E-01 |
| Sum y | -3.84E-02 -3.79E-02 |
| Sum z | -2.36E-10 -2.22E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.06E-03 2.03E-03 |
| Weighted Average x | 3.35E-04 3.36E-04 |
| Weighted Average y | -7.44E-06 -7.45E-06 |
| Weighted Average z | -2.33E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
96 6.4359e-04 1.6986e-05 2.5248e-06 7.1611e-14 0:00:08 20
Updating mesh at iteration... done.
97 4.8419e-04 1.5526e-05 1.8376e-06 6.0134e-14 0:00:09 19
! 97 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 4 SIMULATION TIME = 4.00000E-02 [s] |
+=============================================================================+
Flow time = 0.03s, time step = 3
COUPLING STEP = 4 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.77E-03 1.62E-02 |
| Sum x | 9.04E-01 9.15E-01 |
| Sum y | -3.88E-02 -3.83E-02 |
| Sum z | -2.37E-10 -2.29E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.14E-01 7.92E-01 |
| Weighted Average x | 2.89E-04 2.90E-04 |
| Weighted Average y | -8.40E-06 -8.41E-06 |
| Weighted Average z | -6.55E-19 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
97 4.8419e-04 1.5526e-05 1.8376e-06 6.0134e-14 0:00:10 20
98 3.9582e-02 3.5918e-03 8.2252e-04 4.4025e-12 0:00:08 19
99 3.0993e-02 1.6662e-03 4.6343e-04 2.6277e-12 0:00:06 18
100 2.5199e-02 8.4363e-04 2.7986e-04 1.6751e-12 0:00:05 17
101 2.0294e-02 4.6740e-04 1.7933e-04 1.1319e-12 0:00:04 16
102 1.5752e-02 2.7936e-04 1.2235e-04 8.0179e-13 0:00:03 15
103 1.2811e-02 1.8184e-04 8.7150e-05 5.9384e-13 0:00:02 14
104 1.0251e-02 1.2527e-04 6.4317e-05 4.3828e-13 0:00:02 13
105 8.2670e-03 9.1418e-05 4.8744e-05 3.3373e-13 0:00:01 12
106 6.5928e-03 6.8935e-05 3.7353e-05 2.5694e-13 0:00:01 11
107 5.1994e-03 5.1736e-05 2.8925e-05 1.9385e-13 0:00:01 10
iter continuity x-velocity y-velocity z-velocity time/iter
108 4.1431e-03 4.0757e-05 2.2549e-05 1.4646e-13 0:00:01 9
109 3.2887e-03 3.2929e-05 1.7689e-05 1.1028e-13 0:00:01 8
110 2.6203e-03 2.7455e-05 1.4021e-05 8.6183e-14 0:00:00 7
111 2.0717e-03 2.2674e-05 1.1145e-05 6.4840e-14 0:00:00 6
112 1.6594e-03 1.9055e-05 8.9157e-06 4.9933e-14 0:00:00 5
113 1.3389e-03 1.6295e-05 7.1320e-06 3.8081e-14 0:00:00 4
114 1.0931e-03 1.4209e-05 5.7251e-06 2.9523e-14 0:00:00 3
115 8.9487e-04 1.2564e-05 4.5936e-06 2.3216e-14 0:00:00 2
! 115 solution is converged
Flow time = 0.04s, time step = 4
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
COUPLING STEP = 4 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.23E-01 2.09E-01 |
| Sum x | 7.72E-01 8.44E-01 |
| Sum y | 1.70E-02 -1.07E-02 |
| Sum z | -4.69E-10 -3.49E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.38E-02 1.35E-02 |
| Weighted Average x | 2.84E-04 2.85E-04 |
| Weighted Average y | -7.43E-06 -7.44E-06 |
| Weighted Average z | -1.52E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
115 8.9487e-04 1.2564e-05 4.5936e-06 2.3216e-14 0:00:01 20
Updating mesh at iteration... done.
116 1.1328e-03 1.2178e-05 4.1444e-06 3.9370e-14 0:00:04 19
117 1.0191e-03 1.8887e-05 4.8401e-06 7.1240e-14 0:00:03 18
118 6.8268e-04 1.3768e-05 3.3945e-06 3.3818e-14 0:00:02 17
! 118 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.04s, time step = 4
COUPLING STEP = 4 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.60E-02 8.99E-02 |
| Sum x | 7.71E-01 8.08E-01 |
| Sum y | -1.20E-02 -1.13E-02 |
| Sum z | -4.74E-10 -4.12E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.69E-03 3.65E-03 |
| Weighted Average x | 2.83E-04 2.84E-04 |
| Weighted Average y | -7.46E-06 -7.47E-06 |
| Weighted Average z | -2.11E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
118 6.8268e-04 1.3768e-05 3.3945e-06 3.3818e-14 0:00:03 20
Updating mesh at iteration... done.
119 5.5297e-04 1.1557e-05 2.5747e-06 3.0785e-14 0:00:05 19
! 119 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.04s, time step = 4
COUPLING STEP = 4 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 4.65E-03 4.07E-02 |
| Sum x | 7.71E-01 7.89E-01 |
| Sum y | -9.61E-03 -1.05E-02 |
| Sum z | -4.73E-10 -4.42E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.85E-03 1.83E-03 |
| Weighted Average x | 2.82E-04 2.83E-04 |
| Weighted Average y | -7.43E-06 -7.44E-06 |
| Weighted Average z | -2.42E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
119 5.5297e-04 1.1557e-05 2.5747e-06 3.0785e-14 0:00:06 20
Updating mesh at iteration... done.
120 4.3902e-04 1.2264e-05 1.9689e-06 3.4481e-14 0:00:07 19
! 120 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.04s, time step = 4
COUPLING STEP = 4 COUPLING ITERATION = 5
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 5 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.26E-03 1.83E-02 |
| Sum x | 7.71E-01 7.80E-01 |
| Sum y | -9.57E-03 -1.00E-02 |
| Sum z | -4.74E-10 -4.58E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 9.21E-04 9.12E-04 |
| Weighted Average x | 2.82E-04 2.83E-04 |
| Weighted Average y | -7.41E-06 -7.43E-06 |
| Weighted Average z | -2.58E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
120 4.3902e-04 1.2264e-05 1.9689e-06 3.4481e-14 0:00:08 20
Updating mesh at iteration... done.
121 3.6476e-04 1.0998e-05 1.5905e-06 2.8588e-14 0:00:09 19
! 121 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 5 SIMULATION TIME = 5.00000E-02 [s] |
+=============================================================================+
Flow time = 0.04s, time step = 4
COUPLING STEP = 5 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.66E-03 7.71E-03 |
| Sum x | 7.71E-01 7.76E-01 |
| Sum y | -9.63E-03 -9.82E-03 |
| Sum z | -4.74E-10 -4.66E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.67E-01 7.47E-01 |
| Weighted Average x | 1.50E-04 1.50E-04 |
| Weighted Average y | -8.89E-06 -8.91E-06 |
| Weighted Average z | -9.39E-19 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
121 3.6476e-04 1.0998e-05 1.5905e-06 2.8588e-14 0:00:10 20
122 3.0829e-02 3.2261e-03 7.6479e-04 3.7708e-12 0:00:07 19
123 2.2309e-02 1.4956e-03 4.4404e-04 2.0985e-12 0:00:06 18
124 1.8289e-02 7.5482e-04 2.7198e-04 1.2268e-12 0:00:04 17
125 1.4531e-02 4.1743e-04 1.7515e-04 7.9661e-13 0:00:03 16
126 1.1927e-02 2.5104e-04 1.1941e-04 5.6177e-13 0:00:03 15
127 9.8226e-03 1.6339e-04 8.4681e-05 4.2027e-13 0:00:02 14
128 7.9807e-03 1.1360e-04 6.2091e-05 3.1955e-13 0:00:02 13
129 6.3957e-03 8.2273e-05 4.6540e-05 2.4401e-13 0:00:01 12
130 5.0441e-03 6.1154e-05 3.5181e-05 1.8473e-13 0:00:01 11
131 4.0107e-03 4.6625e-05 2.6838e-05 1.4168e-13 0:00:01 10
iter continuity x-velocity y-velocity z-velocity time/iter
132 3.1907e-03 3.5981e-05 2.0686e-05 1.0580e-13 0:00:01 9
133 2.5456e-03 2.8410e-05 1.6077e-05 8.0217e-14 0:00:01 8
134 2.0467e-03 2.3206e-05 1.2583e-05 6.0736e-14 0:00:00 7
135 1.6541e-03 1.9312e-05 9.9391e-06 4.7917e-14 0:00:00 6
136 1.3403e-03 1.6109e-05 7.8549e-06 3.6043e-14 0:00:00 5
137 1.0894e-03 1.3647e-05 6.2422e-06 2.7685e-14 0:00:00 4
138 8.9695e-04 1.1885e-05 4.9720e-06 2.1884e-14 0:00:00 3
! 138 solution is converged
Flow time = 0.05s, time step = 5
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
COUPLING STEP = 5 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 7.36E-02 7.08E-02 |
| Sum x | 7.02E-01 7.39E-01 |
| Sum y | 3.01E-02 1.01E-02 |
| Sum z | -6.76E-10 -5.71E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.30E-02 1.30E-02 |
| Weighted Average x | 1.48E-04 1.48E-04 |
| Weighted Average y | -8.19E-06 -8.21E-06 |
| Weighted Average z | -1.36E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
138 8.9695e-04 1.1885e-05 4.9720e-06 2.1884e-14 0:00:01 20
Updating mesh at iteration... done.
139 1.0197e-03 1.0940e-05 4.3506e-06 3.7402e-14 0:00:04 19
140 8.3944e-04 1.1125e-05 4.3009e-06 4.3975e-14 0:00:03 18
! 140 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.05s, time step = 5
COUPLING STEP = 5 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 4.64E-03 3.24E-02 |
| Sum x | 7.02E-01 7.20E-01 |
| Sum y | 9.00E-03 9.56E-03 |
| Sum z | -6.78E-10 -6.25E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.00E-03 3.01E-03 |
| Weighted Average x | 1.47E-04 1.47E-04 |
| Weighted Average y | -8.21E-06 -8.23E-06 |
| Weighted Average z | -1.87E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
140 8.3944e-04 1.1125e-05 4.3009e-06 4.3975e-14 0:00:03 20
Updating mesh at iteration... done.
141 6.5455e-04 9.4664e-06 3.2812e-06 2.9362e-14 0:00:06 19
! 141 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.05s, time step = 5
COUPLING STEP = 5 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.16E-03 1.52E-02 |
| Sum x | 7.02E-01 7.11E-01 |
| Sum y | 9.66E-03 9.61E-03 |
| Sum z | -6.79E-10 -6.52E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.53E-03 1.55E-03 |
| Weighted Average x | 1.47E-04 1.47E-04 |
| Weighted Average y | -8.21E-06 -8.23E-06 |
| Weighted Average z | -2.15E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
141 6.5455e-04 9.4664e-06 3.2812e-06 2.9362e-14 0:00:06 20
Updating mesh at iteration... done.
142 5.1411e-04 8.9371e-06 2.4707e-06 2.4176e-14 0:00:08 19
! 142 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.05s, time step = 5
COUPLING STEP = 5 COUPLING ITERATION = 5
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 5 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 9.02E-04 6.84E-03 |
| Sum x | 7.02E-01 7.07E-01 |
| Sum y | 1.04E-02 9.99E-03 |
| Sum z | -6.79E-10 -6.65E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 7.73E-04 7.81E-04 |
| Weighted Average x | 1.46E-04 1.47E-04 |
| Weighted Average y | -8.20E-06 -8.22E-06 |
| Weighted Average z | -2.28E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
142 5.1411e-04 8.9371e-06 2.4707e-06 2.4176e-14 0:00:08 20
Updating mesh at iteration... done.
143 4.0666e-04 8.1103e-06 1.8891e-06 1.9948e-14 0:00:09 19
! 143 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 6 SIMULATION TIME = 6.00000E-02 [s] |
+=============================================================================+
Flow time = 0.05s, time step = 5
COUPLING STEP = 6 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 4.83E-04 3.03E-03 |
| Sum x | 7.02E-01 7.04E-01 |
| Sum y | 1.07E-02 1.03E-02 |
| Sum z | -6.79E-10 -6.72E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.31E-01 8.09E-01 |
| Weighted Average x | -2.61E-05 -2.61E-05 |
| Weighted Average y | -7.14E-06 -7.16E-06 |
| Weighted Average z | -6.26E-19 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
143 4.0666e-04 8.1103e-06 1.8891e-06 1.9948e-14 0:00:10 20
144 2.7844e-02 3.0242e-03 7.2566e-04 2.7218e-12 0:00:08 19
145 1.9345e-02 1.4107e-03 4.3072e-04 1.5130e-12 0:00:06 18
146 1.5385e-02 7.1530e-04 2.6747e-04 7.9213e-13 0:00:05 17
147 1.2598e-02 3.9810e-04 1.7311e-04 4.9272e-13 0:00:04 16
148 1.0317e-02 2.4131e-04 1.1748e-04 3.8050e-13 0:00:03 15
149 8.6726e-03 1.5588e-04 8.2759e-05 2.6832e-13 0:00:02 14
150 7.0342e-03 1.0766e-04 6.0090e-05 2.0252e-13 0:00:02 13
151 5.6347e-03 7.7696e-05 4.4593e-05 1.5760e-13 0:00:01 12
152 4.4852e-03 5.7462e-05 3.3497e-05 1.2292e-13 0:00:01 11
153 3.5876e-03 4.3359e-05 2.5380e-05 9.4858e-14 0:00:01 10
iter continuity x-velocity y-velocity z-velocity time/iter
154 2.8600e-03 3.3136e-05 1.9366e-05 7.1728e-14 0:00:01 9
155 2.2932e-03 2.5914e-05 1.4908e-05 5.4094e-14 0:00:01 8
156 1.8405e-03 2.0761e-05 1.1573e-05 4.1353e-14 0:00:00 7
157 1.4856e-03 1.6999e-05 9.0384e-06 3.2430e-14 0:00:00 6
158 1.2125e-03 1.4067e-05 7.0825e-06 2.5029e-14 0:00:00 5
159 1.0014e-03 1.1631e-05 5.6121e-06 2.0241e-14 0:00:00 4
160 8.2080e-04 9.9767e-06 4.4358e-06 1.6030e-14 0:00:00 3
! 160 solution is converged
Flow time = 0.06s, time step = 6
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
COUPLING STEP = 6 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.40E-01 1.29E-01 |
| Sum x | 6.72E-01 6.88E-01 |
| Sum y | -3.65E-02 -1.31E-02 |
| Sum z | -7.85E-10 -7.28E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 3.54E-02 3.71E-02 |
| Weighted Average x | -2.65E-05 -2.66E-05 |
| Weighted Average y | -7.97E-06 -7.99E-06 |
| Weighted Average z | -7.38E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
160 8.2080e-04 9.9767e-06 4.4358e-06 1.6030e-14 0:00:01 20
Updating mesh at iteration... done.
161 8.8209e-04 8.8190e-06 3.9277e-06 3.2729e-14 0:00:04 19
! 161 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.06s, time step = 6
COUPLING STEP = 6 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 8.86E-03 5.72E-02 |
| Sum x | 6.72E-01 6.80E-01 |
| Sum y | -1.16E-02 -1.24E-02 |
| Sum z | -7.85E-10 -7.57E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 1.22E-02 1.28E-02 |
| Weighted Average x | -2.68E-05 -2.69E-05 |
| Weighted Average y | -7.95E-06 -7.96E-06 |
| Weighted Average z | -1.01E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
161 8.8209e-04 8.8190e-06 3.9277e-06 3.2729e-14 0:00:04 20
Updating mesh at iteration... done.
162 8.0070e-04 1.2311e-05 4.2314e-06 4.5531e-14 0:00:06 19
! 162 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.06s, time step = 6
COUPLING STEP = 6 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 3.29E-03 2.56E-02 |
| Sum x | 6.71E-01 6.76E-01 |
| Sum y | -1.17E-02 -1.21E-02 |
| Sum z | -7.86E-10 -7.72E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.07E-03 6.39E-03 |
| Weighted Average x | -2.70E-05 -2.71E-05 |
| Weighted Average y | -7.93E-06 -7.95E-06 |
| Weighted Average z | -1.16E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
162 8.0070e-04 1.2311e-05 4.2314e-06 4.5531e-14 0:00:07 20
Updating mesh at iteration... done.
163 6.2792e-04 1.0429e-05 3.1240e-06 3.0445e-14 0:00:08 19
! 163 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.06s, time step = 6
COUPLING STEP = 6 COUPLING ITERATION = 5
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 5 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.55E-03 9.62E-03 |
| Sum x | 6.71E-01 6.73E-01 |
| Sum y | -1.31E-02 -1.26E-02 |
| Sum z | -7.86E-10 -7.79E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.08E-03 3.24E-03 |
| Weighted Average x | -2.71E-05 -2.71E-05 |
| Weighted Average y | -7.95E-06 -7.97E-06 |
| Weighted Average z | -1.23E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
163 6.2792e-04 1.0429e-05 3.1240e-06 3.0445e-14 0:00:09 20
Updating mesh at iteration... done.
164 4.6671e-04 9.4137e-06 2.2310e-06 2.7089e-14 0:00:10 19
! 164 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 7 SIMULATION TIME = 7.00000E-02 [s] |
+=============================================================================+
Flow time = 0.06s, time step = 6
COUPLING STEP = 7 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.49E-03 3.52E-03 |
| Sum x | 6.71E-01 6.72E-01 |
| Sum y | -1.33E-02 -1.29E-02 |
| Sum z | -7.86E-10 -7.83E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 7.93E-01 7.72E-01 |
| Weighted Average x | -1.94E-04 -1.94E-04 |
| Weighted Average y | -8.60E-06 -8.62E-06 |
| Weighted Average z | -4.90E-19 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
164 4.6671e-04 9.4137e-06 2.2310e-06 2.7089e-14 0:00:10 20
165 2.5955e-02 2.8177e-03 6.9351e-04 1.5743e-12 0:00:08 19
166 1.7449e-02 1.3156e-03 4.2040e-04 8.9463e-13 0:00:06 18
167 1.3815e-02 6.7143e-04 2.6352e-04 6.3549e-13 0:00:05 17
168 1.1545e-02 3.7439e-04 1.7085e-04 4.7733e-13 0:00:04 16
169 9.7534e-03 2.2615e-04 1.1568e-04 3.6813e-13 0:00:03 15
170 8.2315e-03 1.4660e-04 8.1064e-05 2.8890e-13 0:00:03 14
171 6.7326e-03 1.0101e-04 5.8423e-05 2.2997e-13 0:00:02 13
172 5.4176e-03 7.2834e-05 4.3011e-05 1.8336e-13 0:00:02 12
173 4.2941e-03 5.3218e-05 3.2015e-05 1.4631e-13 0:00:01 11
174 3.4367e-03 3.9795e-05 2.4067e-05 1.1638e-13 0:00:01 10
iter continuity x-velocity y-velocity z-velocity time/iter
175 2.7705e-03 3.0303e-05 1.8255e-05 9.2512e-14 0:00:01 9
176 2.2524e-03 2.3377e-05 1.3965e-05 7.3482e-14 0:00:01 8
177 1.8260e-03 1.8530e-05 1.0750e-05 5.8002e-14 0:00:01 7
178 1.4941e-03 1.4993e-05 8.3492e-06 4.5223e-14 0:00:00 6
179 1.2157e-03 1.2084e-05 6.5261e-06 3.5615e-14 0:00:00 5
180 9.9621e-04 1.0180e-05 5.1132e-06 2.8362e-14 0:00:00 4
! 180 solution is converged
Flow time = 0.07000000000000001s, time step = 7
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
COUPLING STEP = 7 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 4.54E-02 4.38E-02 |
| Sum x | 6.32E-01 6.52E-01 |
| Sum y | 7.38E-03 -2.77E-03 |
| Sum z | -7.21E-10 -7.52E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.28E-03 5.25E-03 |
| Weighted Average x | -1.93E-04 -1.93E-04 |
| Weighted Average y | -8.25E-06 -8.27E-06 |
| Weighted Average z | 3.42E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
180 9.9621e-04 1.0180e-05 5.1132e-06 2.8362e-14 0:00:01 20
Updating mesh at iteration... done.
181 9.9536e-04 8.8718e-06 4.3351e-06 2.3167e-14 0:00:04 19
! 181 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.07s, time step = 7
COUPLING STEP = 7 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.69E-03 2.12E-02 |
| Sum x | 6.32E-01 6.42E-01 |
| Sum y | -1.28E-03 -2.03E-03 |
| Sum z | -7.20E-10 -7.36E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.59E-03 1.65E-03 |
| Weighted Average x | -1.93E-04 -1.94E-04 |
| Weighted Average y | -8.23E-06 -8.24E-06 |
| Weighted Average z | 4.96E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
181 9.9536e-04 8.8718e-06 4.3351e-06 2.3167e-14 0:00:04 20
Updating mesh at iteration... done.
182 8.3560e-04 8.3187e-06 3.7617e-06 2.3414e-14 0:00:06 19
! 182 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.07s, time step = 7
COUPLING STEP = 7 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.47E-03 9.48E-03 |
| Sum x | 6.32E-01 6.37E-01 |
| Sum y | -3.66E-03 -2.84E-03 |
| Sum z | -7.19E-10 -7.28E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 7.52E-04 7.81E-04 |
| Weighted Average x | -1.93E-04 -1.94E-04 |
| Weighted Average y | -8.25E-06 -8.27E-06 |
| Weighted Average z | 5.85E-18 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
182 8.3560e-04 8.3187e-06 3.7617e-06 2.3414e-14 0:00:07 20
Updating mesh at iteration... done.
183 6.6574e-04 7.3133e-06 2.9514e-06 2.0332e-14 0:00:08 19
! 183 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 8 SIMULATION TIME = 8.00000E-02 [s] |
+=============================================================================+
Flow time = 0.07s, time step = 7
COUPLING STEP = 8 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.09E-03 3.92E-03 |
| Sum x | 6.32E-01 6.34E-01 |
| Sum y | -3.35E-03 -3.10E-03 |
| Sum z | -7.19E-10 -7.23E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.13E-01 7.91E-01 |
| Weighted Average x | -3.14E-04 -3.15E-04 |
| Weighted Average y | -8.56E-06 -8.58E-06 |
| Weighted Average z | 5.50E-19 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
183 6.6574e-04 7.3133e-06 2.9514e-06 2.0332e-14 0:00:09 20
184 2.4118e-02 2.6756e-03 6.6009e-04 2.2700e-12 0:00:07 19
185 1.5972e-02 1.2548e-03 4.0506e-04 1.4895e-12 0:00:05 18
186 1.2789e-02 6.4427e-04 2.5650e-04 1.0848e-12 0:00:04 17
187 1.0851e-02 3.6113e-04 1.6703e-04 8.0550e-13 0:00:03 16
188 9.5568e-03 2.1921e-04 1.1313e-04 6.0291e-13 0:00:03 15
189 7.9534e-03 1.4331e-04 7.8960e-05 4.5461e-13 0:00:02 14
190 6.3665e-03 9.7530e-05 5.6625e-05 3.4956e-13 0:00:02 13
191 5.2124e-03 7.0234e-05 4.1352e-05 2.6861e-13 0:00:01 12
192 4.1771e-03 5.0900e-05 3.0503e-05 2.1001e-13 0:00:01 11
193 3.3906e-03 3.7933e-05 2.2810e-05 1.6446e-13 0:00:01 10
iter continuity x-velocity y-velocity z-velocity time/iter
194 2.7509e-03 2.8551e-05 1.7241e-05 1.2951e-13 0:00:01 9
195 2.2415e-03 2.1894e-05 1.3150e-05 1.0166e-13 0:00:01 8
196 1.8293e-03 1.7165e-05 1.0075e-05 7.9826e-14 0:00:00 7
197 1.4932e-03 1.3429e-05 7.7783e-06 6.2498e-14 0:00:00 6
198 1.2317e-03 1.1114e-05 6.0229e-06 4.8853e-14 0:00:00 5
199 1.0097e-03 9.0543e-06 4.7168e-06 3.8120e-14 0:00:00 4
200 8.3603e-04 7.7450e-06 3.7000e-06 2.9807e-14 0:00:00 3
! 200 solution is converged
Flow time = 0.08s, time step = 8
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
COUPLING STEP = 8 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 6.95E-02 6.49E-02 |
| Sum x | 5.87E-01 6.11E-01 |
| Sum y | -1.92E-03 -2.51E-03 |
| Sum z | -5.24E-10 -6.24E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 4.51E-03 4.45E-03 |
| Weighted Average x | -3.13E-04 -3.14E-04 |
| Weighted Average y | -8.54E-06 -8.56E-06 |
| Weighted Average z | 1.27E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
200 8.3603e-04 7.7450e-06 3.7000e-06 2.9807e-14 0:00:01 20
Updating mesh at iteration... done.
201 8.4162e-04 6.8235e-06 3.0897e-06 3.3635e-14 0:00:04 19
! 201 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.08s, time step = 8
COUPLING STEP = 8 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.84E-03 3.01E-02 |
| Sum x | 5.86E-01 5.98E-01 |
| Sum y | -2.48E-03 -2.49E-03 |
| Sum z | -5.24E-10 -5.74E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.20E-03 1.23E-03 |
| Weighted Average x | -3.14E-04 -3.14E-04 |
| Weighted Average y | -8.55E-06 -8.57E-06 |
| Weighted Average z | 1.75E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
201 8.4162e-04 6.8235e-06 3.0897e-06 3.3635e-14 0:00:04 20
Updating mesh at iteration... done.
202 7.2044e-04 7.7014e-06 2.7308e-06 3.1682e-14 0:00:06 19
! 202 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.08s, time step = 8
COUPLING STEP = 8 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.11E-03 1.33E-02 |
| Sum x | 5.86E-01 5.92E-01 |
| Sum y | -2.43E-03 -2.46E-03 |
| Sum z | -5.23E-10 -5.48E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 5.83E-04 5.99E-04 |
| Weighted Average x | -3.14E-04 -3.15E-04 |
| Weighted Average y | -8.54E-06 -8.56E-06 |
| Weighted Average z | 2.01E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
202 7.2044e-04 7.7014e-06 2.7308e-06 3.1682e-14 0:00:07 20
Updating mesh at iteration... done.
203 5.5811e-04 6.2700e-06 2.0953e-06 2.6161e-14 0:00:08 19
! 203 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.08s, time step = 8
COUPLING STEP = 8 COUPLING ITERATION = 5
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 5 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.23E-03 4.90E-03 |
| Sum x | 5.86E-01 5.89E-01 |
| Sum y | -2.29E-03 -2.37E-03 |
| Sum z | -5.22E-10 -5.35E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 2.87E-04 2.95E-04 |
| Weighted Average x | -3.14E-04 -3.15E-04 |
| Weighted Average y | -8.54E-06 -8.56E-06 |
| Weighted Average z | 2.14E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
203 5.5811e-04 6.2700e-06 2.0953e-06 2.6161e-14 0:00:09 20
Updating mesh at iteration... done.
204 4.5832e-04 5.8413e-06 1.6637e-06 2.1931e-14 0:00:10 19
! 204 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 9 SIMULATION TIME = 9.00000E-02 [s] |
+=============================================================================+
Flow time = 0.08s, time step = 8
COUPLING STEP = 9 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.18E-03 1.68E-03 |
| Sum x | 5.86E-01 5.88E-01 |
| Sum y | -2.31E-03 -2.34E-03 |
| Sum z | -5.22E-10 -5.29E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.28E-01 8.06E-01 |
| Weighted Average x | -3.67E-04 -3.68E-04 |
| Weighted Average y | -8.62E-06 -8.64E-06 |
| Weighted Average z | 6.05E-19 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
204 4.5832e-04 5.8413e-06 1.6637e-06 2.1931e-14 0:00:10 20
205 2.3430e-02 2.5310e-03 6.2365e-04 3.6121e-12 0:00:08 19
206 1.5487e-02 1.1873e-03 3.8638e-04 2.2409e-12 0:00:06 18
207 1.2271e-02 6.1389e-04 2.4656e-04 1.4969e-12 0:00:05 17
208 1.0832e-02 3.4622e-04 1.6225e-04 1.0447e-12 0:00:04 16
209 9.3797e-03 2.1048e-04 1.0979e-04 7.4951e-13 0:00:03 15
210 7.9449e-03 1.3638e-04 7.6203e-05 5.4888e-13 0:00:02 14
211 6.5311e-03 9.3415e-05 5.4515e-05 4.0862e-13 0:00:02 13
212 5.2282e-03 6.6969e-05 3.9711e-05 3.0833e-13 0:00:01 12
213 4.1704e-03 4.9454e-05 2.9331e-05 2.3452e-13 0:00:01 11
214 3.3282e-03 3.6412e-05 2.1826e-05 1.7999e-13 0:00:01 10
iter continuity x-velocity y-velocity z-velocity time/iter
215 2.6528e-03 2.7424e-05 1.6423e-05 1.3830e-13 0:00:01 9
216 2.1245e-03 2.0649e-05 1.2399e-05 1.0663e-13 0:00:01 8
217 1.7253e-03 1.6249e-05 9.4336e-06 8.2412e-14 0:00:00 7
218 1.4032e-03 1.2767e-05 7.2701e-06 6.3920e-14 0:00:00 6
219 1.1499e-03 1.0350e-05 5.6146e-06 4.9672e-14 0:00:00 5
220 9.4821e-04 8.3917e-06 4.3867e-06 3.8630e-14 0:00:00 4
! 220 solution is converged
Flow time = 0.09s, time step = 9
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
COUPLING STEP = 9 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.61E-02 2.47E-02 |
| Sum x | 5.23E-01 5.56E-01 |
| Sum y | -7.73E-03 -5.03E-03 |
| Sum z | -2.67E-10 -3.98E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.85E-03 3.71E-03 |
| Weighted Average x | -3.65E-04 -3.66E-04 |
| Weighted Average y | -8.71E-06 -8.73E-06 |
| Weighted Average z | 1.65E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
220 9.4821e-04 8.3917e-06 4.3867e-06 3.8630e-14 0:00:01 20
Updating mesh at iteration... done.
221 9.0470e-04 7.6178e-06 3.6394e-06 4.3829e-14 0:00:04 19
! 221 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.09s, time step = 9
COUPLING STEP = 9 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.24E-03 1.25E-02 |
| Sum x | 5.23E-01 5.39E-01 |
| Sum y | -5.61E-03 -5.32E-03 |
| Sum z | -2.65E-10 -3.31E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.32E-03 1.33E-03 |
| Weighted Average x | -3.66E-04 -3.67E-04 |
| Weighted Average y | -8.73E-06 -8.75E-06 |
| Weighted Average z | 2.29E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
221 9.0470e-04 7.6178e-06 3.6394e-06 4.3829e-14 0:00:04 20
Updating mesh at iteration... done.
222 7.7546e-04 6.4021e-06 3.0497e-06 3.7308e-14 0:00:06 19
! 222 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.09s, time step = 9
COUPLING STEP = 9 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 3.06E-04 6.17E-03 |
| Sum x | 5.23E-01 5.31E-01 |
| Sum y | -4.63E-03 -4.97E-03 |
| Sum z | -2.64E-10 -2.98E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 6.29E-04 6.35E-04 |
| Weighted Average x | -3.66E-04 -3.67E-04 |
| Weighted Average y | -8.71E-06 -8.73E-06 |
| Weighted Average z | 2.63E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
222 7.7546e-04 6.4021e-06 3.0497e-06 3.7308e-14 0:00:07 20
Updating mesh at iteration... done.
223 6.0162e-04 5.5743e-06 2.3710e-06 3.0807e-14 0:00:08 19
! 223 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
+=============================================================================+
| COUPLING STEP = 10 SIMULATION TIME = 1.00000E-01 [s] |
+=============================================================================+
Flow time = 0.09s, time step = 9
COUPLING STEP = 10 COUPLING ITERATION = 1
+=============================================================================+
| COUPLING ITERATIONS |
+-----------------------------------------------------------------------------+
| | Source Target |
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 1 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 2.26E-04 3.02E-03 |
| Sum x | 5.23E-01 5.27E-01 |
| Sum y | -4.46E-03 -4.71E-03 |
| Sum z | -2.63E-10 -2.80E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Not yet converged |
| RMS Change | 8.26E-01 8.05E-01 |
| Weighted Average x | -3.36E-04 -3.37E-04 |
| Weighted Average y | -9.07E-06 -9.09E-06 |
| Weighted Average z | 1.85E-18 0.00E+00 |
Updating solution at time level N...
done.
Updating mesh at time level N... done.
iter continuity x-velocity y-velocity z-velocity time/iter
223 6.0162e-04 5.5743e-06 2.3710e-06 3.0807e-14 0:00:09 20
224 2.2981e-02 2.4249e-03 5.8414e-04 4.4048e-12 0:00:07 19
225 1.4529e-02 1.1458e-03 3.6850e-04 2.5871e-12 0:00:05 18
226 1.1800e-02 5.9504e-04 2.3652e-04 1.6400e-12 0:00:04 17
227 1.0619e-02 3.3739e-04 1.5590e-04 1.1011e-12 0:00:03 16
228 9.0651e-03 2.0554e-04 1.0537e-04 7.7264e-13 0:00:03 15
229 7.5923e-03 1.3382e-04 7.3245e-05 5.5969e-13 0:00:02 14
230 6.0904e-03 9.1166e-05 5.2334e-05 4.1226e-13 0:00:02 13
231 4.9379e-03 6.5381e-05 3.7943e-05 3.0846e-13 0:00:01 12
232 3.9054e-03 4.6714e-05 2.7860e-05 2.3277e-13 0:00:01 11
233 3.1144e-03 3.4235e-05 2.0674e-05 1.7814e-13 0:00:01 10
iter continuity x-velocity y-velocity z-velocity time/iter
234 2.4932e-03 2.5879e-05 1.5488e-05 1.3707e-13 0:00:01 9
235 1.9813e-03 1.9516e-05 1.1707e-05 1.0573e-13 0:00:01 8
236 1.5947e-03 1.5278e-05 8.9188e-06 8.1770e-14 0:00:00 7
237 1.3080e-03 1.2225e-05 6.8464e-06 6.3311e-14 0:00:00 6
238 1.0777e-03 9.6452e-06 5.3162e-06 4.9048e-14 0:00:00 5
239 8.9233e-04 8.1141e-06 4.1222e-06 3.7938e-14 0:00:00 4
! 239 solution is converged
Flow time = 0.09999999999999999s, time step = 10
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
COUPLING STEP = 10 COUPLING ITERATION = 2
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 2 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 6.46E-02 5.97E-02 |
| Sum x | 4.59E-01 4.93E-01 |
| Sum y | 2.49E-04 -2.23E-03 |
| Sum z | 1.71E-11 -1.32E-10 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.05E-03 2.94E-03 |
| Weighted Average x | -3.35E-04 -3.36E-04 |
| Weighted Average y | -8.99E-06 -9.01E-06 |
| Weighted Average z | 1.99E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
239 8.9233e-04 8.1141e-06 4.1222e-06 3.7938e-14 0:00:01 20
Updating mesh at iteration... done.
240 8.2429e-04 7.0932e-06 3.4274e-06 3.1389e-14 0:00:04 19
! 240 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.1s, time step = 10
COUPLING STEP = 10 COUPLING ITERATION = 3
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 3 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 1.67E-03 2.89E-02 |
| Sum x | 4.58E-01 4.76E-01 |
| Sum y | -1.89E-03 -2.06E-03 |
| Sum z | 1.82E-11 -5.67E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 1.68E-03 1.68E-03 |
| Weighted Average x | -3.36E-04 -3.37E-04 |
| Weighted Average y | -8.98E-06 -9.00E-06 |
| Weighted Average z | 2.71E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
240 8.2429e-04 7.0932e-06 3.4274e-06 3.1389e-14 0:00:04 20
Updating mesh at iteration... done.
241 7.2722e-04 6.6301e-06 2.8241e-06 2.7580e-14 0:00:06 19
! 241 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.1s, time step = 10
COUPLING STEP = 10 COUPLING ITERATION = 4
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 4 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Not yet converged |
| RMS Change | 2.53E-03 1.25E-02 |
| Sum x | 4.59E-01 4.67E-01 |
| Sum y | -1.84E-03 -1.95E-03 |
| Sum z | 1.89E-11 -1.89E-11 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 7.86E-04 7.87E-04 |
| Weighted Average x | -3.36E-04 -3.37E-04 |
| Weighted Average y | -8.98E-06 -9.00E-06 |
| Weighted Average z | 3.09E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
241 7.2722e-04 6.6301e-06 2.8241e-06 2.7580e-14 0:00:07 20
Updating mesh at iteration... done.
242 5.9093e-04 6.1421e-06 2.2522e-06 2.2174e-14 0:00:08 19
! 242 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
Flow time = 0.1s, time step = 10
COUPLING STEP = 10 COUPLING ITERATION = 5
+-----------------------------------------------------------------------------+
| COUPLING ITERATION = 5 |
+-----------------------------------------------------------------------------+
| MAPDL-2 | |
| Interface: Interface-1 | |
| Force | Converged |
| RMS Change | 1.37E-03 5.32E-03 |
| Sum x | 4.59E-01 4.63E-01 |
| Sum y | -2.03E-03 -1.99E-03 |
| Sum z | 1.98E-11 4.59E-13 |
+-----------------------------------------------------------------------------+
| FLUENT-1 | |
| Interface: Interface-1 | |
| displacement | Converged |
| RMS Change | 3.86E-04 3.87E-04 |
| Weighted Average x | -3.36E-04 -3.37E-04 |
| Weighted Average y | -8.98E-06 -9.00E-06 |
| Weighted Average z | 3.29E-17 0.00E+00 |
iter continuity x-velocity y-velocity z-velocity time/iter
242 5.9093e-04 6.1421e-06 2.2522e-06 2.2174e-14 0:00:09 20
Updating mesh at iteration... done.
243 4.7522e-04 5.3433e-06 1.7620e-06 1.7590e-14 0:00:10 19
! 243 solution is converged
+-----------------------------------------------------------------------------+
| Participant solution status | |
| MAPDL-2 | Converged |
| FLUENT-1 | Converged |
+=============================================================================+
===============================================================================
+=============================================================================+
| |
| Shut Down |
| |
+=============================================================================+
===============================================================================
+=============================================================================+
| Available Restart Points |
+=============================================================================+
| Restart Point | File Name |
+-----------------------------------------------------------------------------+
| Coupling Step 10 | Restart_step10.h5 |
+=============================================================================+
Fast-loading "/ansys_inc/v242/fluent/fluent24.2.0/addons/afd/lib/hdfio.bin"
Done.
Writing to 2e13acf0c0b2:"/mnt/pyfluent/.//turek_hron_fluid-0-00010.cas.h5" in NODE0 mode and compression level 1 ...
Grouping cells for Laplace smoothing ...
6728 cells, 1 zone ...
27150 faces, 8 zones ...
13932 nodes, 1 zone ...
Done.
Done.
Writing to 2e13acf0c0b2:"/mnt/pyfluent/.//turek_hron_fluid-0-00010.dat.h5" in NODE0 mode and compression level 1 ...
Writing results.
Done.
Create new project file for writing: turek_hron_fluid_resolved.flprj
Flow time = 0.1s, time step = 10
Writing to 2e13acf0c0b2:"/mnt/pyfluent/.//turek_hron_fluid-0-00010.cas.h5" in NODE0 mode and compression level 1 ...
Grouping cells for Laplace smoothing ...
6728 cells, 1 zone ...
27150 faces, 8 zones ...
13932 nodes, 1 zone ...
Done.
Done.
Writing to 2e13acf0c0b2:"/mnt/pyfluent/.//turek_hron_fluid-0-00010.dat.h5" in NODE0 mode and compression level 1 ...
Writing results.
Done.
+=============================================================================+
| Timing Summary [s] |
+=============================================================================+
| Total Time : 6.48841E+01 |
| Coupling Participant Time |
| FLUENT-1 : 5.23695E+01 |
| MAPDL-2 : 5.43232E+00 |
| Total : 5.78018E+01 |
| Coupling Engine Time |
| Solution Control : 3.95598E+00 |
| Mesh Import : 1.52678E-02 |
| Mapping Setup : 2.78401E-03 |
| Mapping : 6.51860E-03 |
| Numerics : 1.94266E-02 |
| Misc. : 3.08232E+00 |
| Total : 7.08230E+00 |
+=============================================================================+
+=============================================================================+
| System coupling run completed successfully. |
+=============================================================================+
Postprocessing#
Postprocess the structural results#
mapdl.finish()
mapdl.post1()
mapdl.nsel("s", "loc", "x", 0.45) # select the right side of the beam
mapdl.nsel("r", "loc", "y", 0.00) # select the top of the beam
tip_node = mapdl.nsel("r", "loc", "z", 0.005)[0] # select the tip node
tip_y_0 = mapdl.get_value("node", tip_node, "loc", "y")
tip_y = []
nsets = mapdl.post_processing.nsets
for i in range(1, nsets + 1):
mapdl.set(i, 1)
u_y = mapdl.get_value("node", tip_node, "u", "y")
tip_y.append(tip_y_0 + u_y)
time_values = mapdl.post_processing.time_values
plt.plot(time_values, tip_y)
plt.xlabel("t (s)")
plt.ylabel(r"$x_{tip}$ (m)")
plt.savefig("turek_horn_fsi2_tip_disp.png")
# If you want to see the in-line plot, comment following line.
plt.close() # close the plot to avoid showing it in the docs.
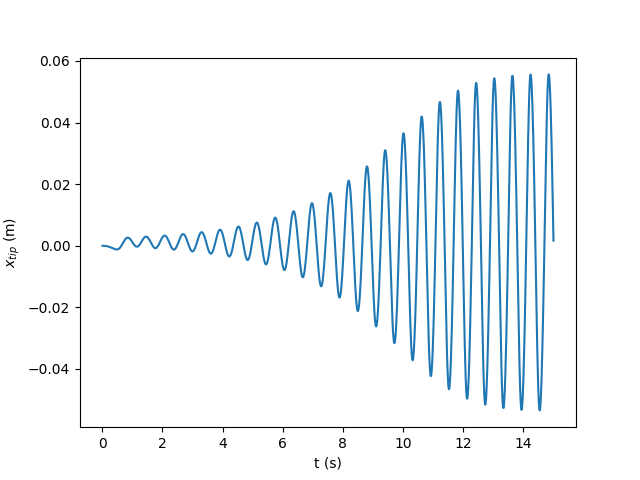
Postprocess the fluids results#
use_window_resolution option not active inside containers or Ansys Lab environment
if fluent.results.graphics.picture.use_window_resolution.is_active():
fluent.results.graphics.picture.use_window_resolution = False
fluent.results.graphics.picture.x_resolution = 1920
fluent.results.graphics.picture.y_resolution = 1440
fluent.results.graphics.contour["contour_static_pressure"] = {}
contour = fluent.results.graphics.contour["contour_static_pressure"]
contour.coloring.option = "banded"
contour.field = "pressure"
contour.filled = True
contour.surfaces_list = ["symmetry_bot"]
contour.display()
fluent.results.graphics.views.restore_view(view_name="front")
fluent.results.graphics.views.auto_scale()
fluent.results.graphics.picture.save_picture(
file_name="turek_horn_fsi2_pressure_contour.png"
)
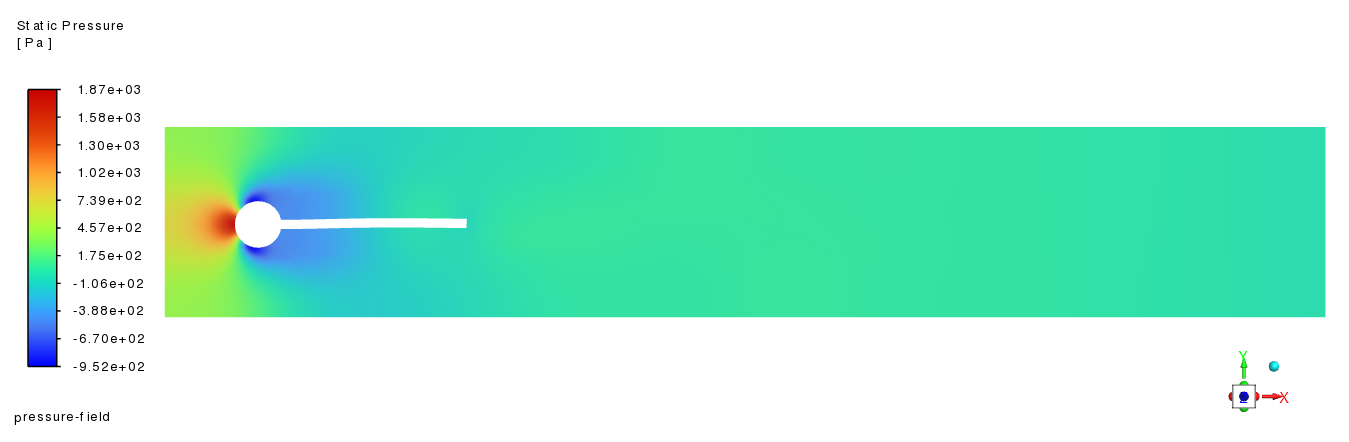
Exit#
syc.exit()
fluent.exit()
mapdl.exit()
/opt/hostedtoolcache/Python/3.10.15/x64/lib/python3.10/site-packages/ansys/mapdl/core/launcher.py:822: UserWarning: The environment variable 'PYMAPDL_START_INSTANCE' is set, hence the argument 'start_instance' is overwritten.
warnings.warn(
Note#
The results shown in this example are for illustrative purposes only. To get similar results, you may need to run the simulation with an end_time equal to 15[s]. Refer to the section time step size, end time, output controls section to update the end time.
# The results shown below are at end_time as 15 [s] and it took approx. 2 hrs based on
# the compute resource used.Please note that the runtime may vary depending on the
# compute resources used.
# Velocity field at 15 [sec]
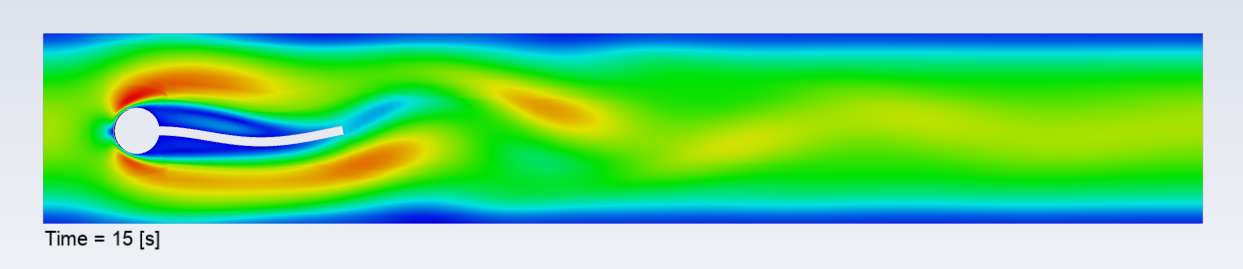
# Pressure field at 15 [sec]
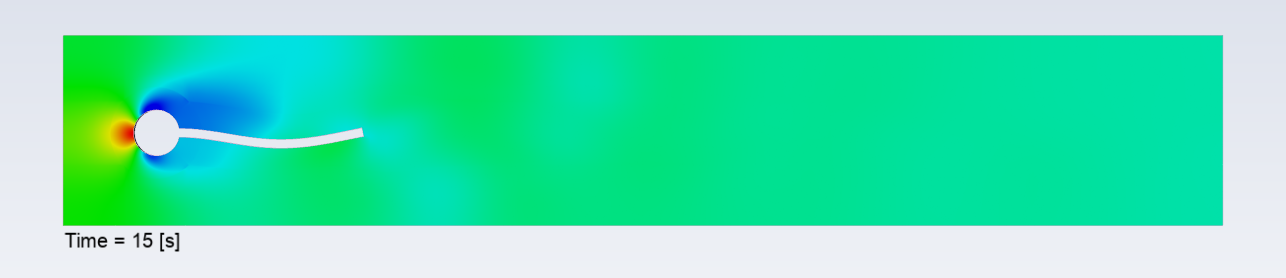
References#
[1]. Turek, S., & Hron, J. (2006). Proposal for numerical benchmarking of fluid-structure interaction between an elastic object and laminar incompressible flow (pp. 371-385). Springer Berlin Heidelberg.
Total running time of the script: (1 minutes 45.161 seconds)